version 0.0.1 - HTTP & SocketIo services on server
This commit is contained in:
parent
5898ce49ca
commit
edd511b402
21
LICENSE
Normal file
21
LICENSE
Normal file
@ -0,0 +1,21 @@
|
||||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) 2015 Duco Winterwerp
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
58
nodePong.js
Normal file
58
nodePong.js
Normal file
@ -0,0 +1,58 @@
|
||||
/**
|
||||
* @file nodePong.js
|
||||
* @author frtk
|
||||
*/
|
||||
|
||||
|
||||
/*
|
||||
* nodejs modules
|
||||
*/
|
||||
//---
|
||||
var fs = require('fs');
|
||||
var colors = require('colors');
|
||||
var express = require('express');
|
||||
var http = require('http');
|
||||
var iosocket = require('socket.io');
|
||||
//--- server app libs & params
|
||||
var servU = require('./server/server_utils.js');
|
||||
var servP = require('./server/server_params.js');
|
||||
|
||||
|
||||
/*
|
||||
* starting HTTP and socket.io services
|
||||
*/
|
||||
//---
|
||||
serverMsg("$ ##### nodePong - v0.0.1 ");
|
||||
|
||||
//--- HTTP server
|
||||
serverMsg("$ # starting http service on port " + servP.params.port);
|
||||
var app = express();
|
||||
var httpserv = http.createServer(app);
|
||||
httpserv.listen(servP.params.port);
|
||||
//--- allow access to static files from "/public" directory
|
||||
app.use(express.static(__dirname + '/public/'));
|
||||
|
||||
//--- socket.io
|
||||
serverMsg('$ # registering socket.io service on port ' + servP.params.port);
|
||||
var io = require('socket.io').listen(httpserv, {log: false});
|
||||
|
||||
serverMsg('$ #####');
|
||||
|
||||
|
||||
|
||||
|
||||
/*
|
||||
* running nodePong server
|
||||
*/
|
||||
//---
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
/*
|
||||
* FUNCTIONS
|
||||
*/
|
||||
function serverMsg(msg) {
|
||||
return console.log((servU.datelib.newDateToString() + msg).yellow);
|
||||
}
|
23
node_modules/colors/LICENSE
generated
vendored
Normal file
23
node_modules/colors/LICENSE
generated
vendored
Normal file
@ -0,0 +1,23 @@
|
||||
Original Library
|
||||
- Copyright (c) Marak Squires
|
||||
|
||||
Additional Functionality
|
||||
- Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com)
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
178
node_modules/colors/ReadMe.md
generated
vendored
Normal file
178
node_modules/colors/ReadMe.md
generated
vendored
Normal file
@ -0,0 +1,178 @@
|
||||
# colors.js [](https://travis-ci.org/Marak/colors.js)
|
||||
|
||||
## get color and style in your node.js console
|
||||
|
||||
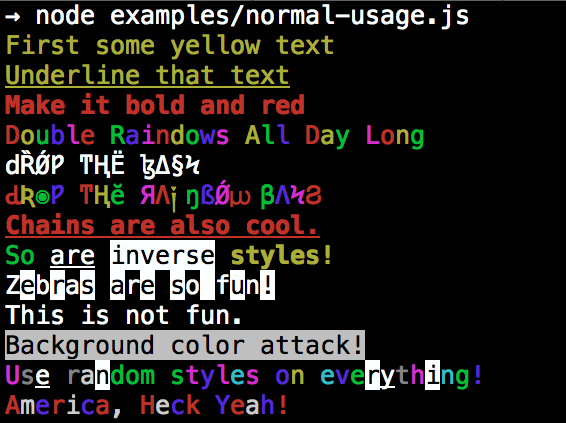
|
||||
|
||||
## Installation
|
||||
|
||||
npm install colors
|
||||
|
||||
## colors and styles!
|
||||
|
||||
### text colors
|
||||
|
||||
- black
|
||||
- red
|
||||
- green
|
||||
- yellow
|
||||
- blue
|
||||
- magenta
|
||||
- cyan
|
||||
- white
|
||||
- gray
|
||||
- grey
|
||||
|
||||
### background colors
|
||||
|
||||
- bgBlack
|
||||
- bgRed
|
||||
- bgGreen
|
||||
- bgYellow
|
||||
- bgBlue
|
||||
- bgMagenta
|
||||
- bgCyan
|
||||
- bgWhite
|
||||
|
||||
### styles
|
||||
|
||||
- reset
|
||||
- bold
|
||||
- dim
|
||||
- italic
|
||||
- underline
|
||||
- inverse
|
||||
- hidden
|
||||
- strikethrough
|
||||
|
||||
### extras
|
||||
|
||||
- rainbow
|
||||
- zebra
|
||||
- america
|
||||
- trap
|
||||
- random
|
||||
|
||||
|
||||
## Usage
|
||||
|
||||
By popular demand, `colors` now ships with two types of usages!
|
||||
|
||||
The super nifty way
|
||||
|
||||
```js
|
||||
var colors = require('colors');
|
||||
|
||||
console.log('hello'.green); // outputs green text
|
||||
console.log('i like cake and pies'.underline.red) // outputs red underlined text
|
||||
console.log('inverse the color'.inverse); // inverses the color
|
||||
console.log('OMG Rainbows!'.rainbow); // rainbow
|
||||
console.log('Run the trap'.trap); // Drops the bass
|
||||
|
||||
```
|
||||
|
||||
or a slightly less nifty way which doesn't extend `String.prototype`
|
||||
|
||||
```js
|
||||
var colors = require('colors/safe');
|
||||
|
||||
console.log(colors.green('hello')); // outputs green text
|
||||
console.log(colors.red.underline('i like cake and pies')) // outputs red underlined text
|
||||
console.log(colors.inverse('inverse the color')); // inverses the color
|
||||
console.log(colors.rainbow('OMG Rainbows!')); // rainbow
|
||||
console.log(colors.trap('Run the trap')); // Drops the bass
|
||||
|
||||
```
|
||||
|
||||
I prefer the first way. Some people seem to be afraid of extending `String.prototype` and prefer the second way.
|
||||
|
||||
If you are writing good code you will never have an issue with the first approach. If you really don't want to touch `String.prototype`, the second usage will not touch `String` native object.
|
||||
|
||||
## Disabling Colors
|
||||
|
||||
To disable colors you can pass the following arguments in the command line to your application:
|
||||
|
||||
```bash
|
||||
node myapp.js --no-color
|
||||
```
|
||||
|
||||
## Console.log [string substitution](http://nodejs.org/docs/latest/api/console.html#console_console_log_data)
|
||||
|
||||
```js
|
||||
var name = 'Marak';
|
||||
console.log(colors.green('Hello %s'), name);
|
||||
// outputs -> 'Hello Marak'
|
||||
```
|
||||
|
||||
## Custom themes
|
||||
|
||||
### Using standard API
|
||||
|
||||
```js
|
||||
|
||||
var colors = require('colors');
|
||||
|
||||
colors.setTheme({
|
||||
silly: 'rainbow',
|
||||
input: 'grey',
|
||||
verbose: 'cyan',
|
||||
prompt: 'grey',
|
||||
info: 'green',
|
||||
data: 'grey',
|
||||
help: 'cyan',
|
||||
warn: 'yellow',
|
||||
debug: 'blue',
|
||||
error: 'red'
|
||||
});
|
||||
|
||||
// outputs red text
|
||||
console.log("this is an error".error);
|
||||
|
||||
// outputs yellow text
|
||||
console.log("this is a warning".warn);
|
||||
```
|
||||
|
||||
### Using string safe API
|
||||
|
||||
```js
|
||||
var colors = require('colors/safe');
|
||||
|
||||
// set single property
|
||||
var error = colors.red;
|
||||
error('this is red');
|
||||
|
||||
// set theme
|
||||
colors.setTheme({
|
||||
silly: 'rainbow',
|
||||
input: 'grey',
|
||||
verbose: 'cyan',
|
||||
prompt: 'grey',
|
||||
info: 'green',
|
||||
data: 'grey',
|
||||
help: 'cyan',
|
||||
warn: 'yellow',
|
||||
debug: 'blue',
|
||||
error: 'red'
|
||||
});
|
||||
|
||||
// outputs red text
|
||||
console.log(colors.error("this is an error"));
|
||||
|
||||
// outputs yellow text
|
||||
console.log(colors.warn("this is a warning"));
|
||||
|
||||
```
|
||||
|
||||
You can also combine them:
|
||||
|
||||
```javascript
|
||||
var colors = require('colors');
|
||||
|
||||
colors.setTheme({
|
||||
custom: ['red', 'underline']
|
||||
});
|
||||
|
||||
console.log('test'.custom);
|
||||
```
|
||||
|
||||
*Protip: There is a secret undocumented style in `colors`. If you find the style you can summon him.*
|
74
node_modules/colors/examples/normal-usage.js
generated
vendored
Normal file
74
node_modules/colors/examples/normal-usage.js
generated
vendored
Normal file
@ -0,0 +1,74 @@
|
||||
var colors = require('../lib/index');
|
||||
|
||||
console.log("First some yellow text".yellow);
|
||||
|
||||
console.log("Underline that text".yellow.underline);
|
||||
|
||||
console.log("Make it bold and red".red.bold);
|
||||
|
||||
console.log(("Double Raindows All Day Long").rainbow)
|
||||
|
||||
console.log("Drop the bass".trap)
|
||||
|
||||
console.log("DROP THE RAINBOW BASS".trap.rainbow)
|
||||
|
||||
|
||||
console.log('Chains are also cool.'.bold.italic.underline.red); // styles not widely supported
|
||||
|
||||
console.log('So '.green + 'are'.underline + ' ' + 'inverse'.inverse + ' styles! '.yellow.bold); // styles not widely supported
|
||||
console.log("Zebras are so fun!".zebra);
|
||||
|
||||
//
|
||||
// Remark: .strikethrough may not work with Mac OS Terminal App
|
||||
//
|
||||
console.log("This is " + "not".strikethrough + " fun.");
|
||||
|
||||
console.log('Background color attack!'.black.bgWhite)
|
||||
console.log('Use random styles on everything!'.random)
|
||||
console.log('America, Heck Yeah!'.america)
|
||||
|
||||
|
||||
console.log('Setting themes is useful')
|
||||
|
||||
//
|
||||
// Custom themes
|
||||
//
|
||||
console.log('Generic logging theme as JSON'.green.bold.underline);
|
||||
// Load theme with JSON literal
|
||||
colors.setTheme({
|
||||
silly: 'rainbow',
|
||||
input: 'grey',
|
||||
verbose: 'cyan',
|
||||
prompt: 'grey',
|
||||
info: 'green',
|
||||
data: 'grey',
|
||||
help: 'cyan',
|
||||
warn: 'yellow',
|
||||
debug: 'blue',
|
||||
error: 'red'
|
||||
});
|
||||
|
||||
// outputs red text
|
||||
console.log("this is an error".error);
|
||||
|
||||
// outputs yellow text
|
||||
console.log("this is a warning".warn);
|
||||
|
||||
// outputs grey text
|
||||
console.log("this is an input".input);
|
||||
|
||||
console.log('Generic logging theme as file'.green.bold.underline);
|
||||
|
||||
// Load a theme from file
|
||||
colors.setTheme(__dirname + '/../themes/generic-logging.js');
|
||||
|
||||
// outputs red text
|
||||
console.log("this is an error".error);
|
||||
|
||||
// outputs yellow text
|
||||
console.log("this is a warning".warn);
|
||||
|
||||
// outputs grey text
|
||||
console.log("this is an input".input);
|
||||
|
||||
//console.log("Don't summon".zalgo)
|
76
node_modules/colors/examples/safe-string.js
generated
vendored
Normal file
76
node_modules/colors/examples/safe-string.js
generated
vendored
Normal file
@ -0,0 +1,76 @@
|
||||
var colors = require('../safe');
|
||||
|
||||
console.log(colors.yellow("First some yellow text"));
|
||||
|
||||
console.log(colors.yellow.underline("Underline that text"));
|
||||
|
||||
console.log(colors.red.bold("Make it bold and red"));
|
||||
|
||||
console.log(colors.rainbow("Double Raindows All Day Long"))
|
||||
|
||||
console.log(colors.trap("Drop the bass"))
|
||||
|
||||
console.log(colors.rainbow(colors.trap("DROP THE RAINBOW BASS")));
|
||||
|
||||
console.log(colors.bold.italic.underline.red('Chains are also cool.')); // styles not widely supported
|
||||
|
||||
|
||||
console.log(colors.green('So ') + colors.underline('are') + ' ' + colors.inverse('inverse') + colors.yellow.bold(' styles! ')); // styles not widely supported
|
||||
|
||||
console.log(colors.zebra("Zebras are so fun!"));
|
||||
|
||||
console.log("This is " + colors.strikethrough("not") + " fun.");
|
||||
|
||||
|
||||
console.log(colors.black.bgWhite('Background color attack!'));
|
||||
console.log(colors.random('Use random styles on everything!'))
|
||||
console.log(colors.america('America, Heck Yeah!'));
|
||||
|
||||
console.log('Setting themes is useful')
|
||||
|
||||
//
|
||||
// Custom themes
|
||||
//
|
||||
//console.log('Generic logging theme as JSON'.green.bold.underline);
|
||||
// Load theme with JSON literal
|
||||
colors.setTheme({
|
||||
silly: 'rainbow',
|
||||
input: 'grey',
|
||||
verbose: 'cyan',
|
||||
prompt: 'grey',
|
||||
info: 'green',
|
||||
data: 'grey',
|
||||
help: 'cyan',
|
||||
warn: 'yellow',
|
||||
debug: 'blue',
|
||||
error: 'red'
|
||||
});
|
||||
|
||||
// outputs red text
|
||||
console.log(colors.error("this is an error"));
|
||||
|
||||
// outputs yellow text
|
||||
console.log(colors.warn("this is a warning"));
|
||||
|
||||
// outputs grey text
|
||||
console.log(colors.input("this is an input"));
|
||||
|
||||
|
||||
// console.log('Generic logging theme as file'.green.bold.underline);
|
||||
|
||||
// Load a theme from file
|
||||
colors.setTheme(__dirname + '/../themes/generic-logging.js');
|
||||
|
||||
// outputs red text
|
||||
console.log(colors.error("this is an error"));
|
||||
|
||||
// outputs yellow text
|
||||
console.log(colors.warn("this is a warning"));
|
||||
|
||||
// outputs grey text
|
||||
console.log(colors.input("this is an input"));
|
||||
|
||||
// console.log(colors.zalgo("Don't summon him"))
|
||||
|
||||
|
||||
|
187
node_modules/colors/lib/colors.js
generated
vendored
Normal file
187
node_modules/colors/lib/colors.js
generated
vendored
Normal file
@ -0,0 +1,187 @@
|
||||
/*
|
||||
|
||||
The MIT License (MIT)
|
||||
|
||||
Original Library
|
||||
- Copyright (c) Marak Squires
|
||||
|
||||
Additional functionality
|
||||
- Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com)
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
||||
|
||||
*/
|
||||
|
||||
var colors = {};
|
||||
module['exports'] = colors;
|
||||
|
||||
colors.themes = {};
|
||||
|
||||
var ansiStyles = colors.styles = require('./styles');
|
||||
var defineProps = Object.defineProperties;
|
||||
|
||||
colors.supportsColor = require('./system/supports-colors');
|
||||
|
||||
if (typeof colors.enabled === "undefined") {
|
||||
colors.enabled = colors.supportsColor;
|
||||
}
|
||||
|
||||
colors.stripColors = colors.strip = function(str){
|
||||
return ("" + str).replace(/\x1B\[\d+m/g, '');
|
||||
};
|
||||
|
||||
|
||||
var stylize = colors.stylize = function stylize (str, style) {
|
||||
if (!colors.enabled) {
|
||||
return str+'';
|
||||
}
|
||||
|
||||
return ansiStyles[style].open + str + ansiStyles[style].close;
|
||||
}
|
||||
|
||||
var matchOperatorsRe = /[|\\{}()[\]^$+*?.]/g;
|
||||
var escapeStringRegexp = function (str) {
|
||||
if (typeof str !== 'string') {
|
||||
throw new TypeError('Expected a string');
|
||||
}
|
||||
return str.replace(matchOperatorsRe, '\\$&');
|
||||
}
|
||||
|
||||
function build(_styles) {
|
||||
var builder = function builder() {
|
||||
return applyStyle.apply(builder, arguments);
|
||||
};
|
||||
builder._styles = _styles;
|
||||
// __proto__ is used because we must return a function, but there is
|
||||
// no way to create a function with a different prototype.
|
||||
builder.__proto__ = proto;
|
||||
return builder;
|
||||
}
|
||||
|
||||
var styles = (function () {
|
||||
var ret = {};
|
||||
ansiStyles.grey = ansiStyles.gray;
|
||||
Object.keys(ansiStyles).forEach(function (key) {
|
||||
ansiStyles[key].closeRe = new RegExp(escapeStringRegexp(ansiStyles[key].close), 'g');
|
||||
ret[key] = {
|
||||
get: function () {
|
||||
return build(this._styles.concat(key));
|
||||
}
|
||||
};
|
||||
});
|
||||
return ret;
|
||||
})();
|
||||
|
||||
var proto = defineProps(function colors() {}, styles);
|
||||
|
||||
function applyStyle() {
|
||||
var args = arguments;
|
||||
var argsLen = args.length;
|
||||
var str = argsLen !== 0 && String(arguments[0]);
|
||||
if (argsLen > 1) {
|
||||
for (var a = 1; a < argsLen; a++) {
|
||||
str += ' ' + args[a];
|
||||
}
|
||||
}
|
||||
|
||||
if (!colors.enabled || !str) {
|
||||
return str;
|
||||
}
|
||||
|
||||
var nestedStyles = this._styles;
|
||||
|
||||
var i = nestedStyles.length;
|
||||
while (i--) {
|
||||
var code = ansiStyles[nestedStyles[i]];
|
||||
str = code.open + str.replace(code.closeRe, code.open) + code.close;
|
||||
}
|
||||
|
||||
return str;
|
||||
}
|
||||
|
||||
function applyTheme (theme) {
|
||||
for (var style in theme) {
|
||||
(function(style){
|
||||
colors[style] = function(str){
|
||||
if (typeof theme[style] === 'object'){
|
||||
var out = str;
|
||||
for (var i in theme[style]){
|
||||
out = colors[theme[style][i]](out);
|
||||
}
|
||||
return out;
|
||||
}
|
||||
return colors[theme[style]](str);
|
||||
};
|
||||
})(style)
|
||||
}
|
||||
}
|
||||
|
||||
colors.setTheme = function (theme) {
|
||||
if (typeof theme === 'string') {
|
||||
try {
|
||||
colors.themes[theme] = require(theme);
|
||||
applyTheme(colors.themes[theme]);
|
||||
return colors.themes[theme];
|
||||
} catch (err) {
|
||||
console.log(err);
|
||||
return err;
|
||||
}
|
||||
} else {
|
||||
applyTheme(theme);
|
||||
}
|
||||
};
|
||||
|
||||
function init() {
|
||||
var ret = {};
|
||||
Object.keys(styles).forEach(function (name) {
|
||||
ret[name] = {
|
||||
get: function () {
|
||||
return build([name]);
|
||||
}
|
||||
};
|
||||
});
|
||||
return ret;
|
||||
}
|
||||
|
||||
var sequencer = function sequencer (map, str) {
|
||||
var exploded = str.split(""), i = 0;
|
||||
exploded = exploded.map(map);
|
||||
return exploded.join("");
|
||||
};
|
||||
|
||||
// custom formatter methods
|
||||
colors.trap = require('./custom/trap');
|
||||
colors.zalgo = require('./custom/zalgo');
|
||||
|
||||
// maps
|
||||
colors.maps = {};
|
||||
colors.maps.america = require('./maps/america');
|
||||
colors.maps.zebra = require('./maps/zebra');
|
||||
colors.maps.rainbow = require('./maps/rainbow');
|
||||
colors.maps.random = require('./maps/random')
|
||||
|
||||
for (var map in colors.maps) {
|
||||
(function(map){
|
||||
colors[map] = function (str) {
|
||||
return sequencer(colors.maps[map], str);
|
||||
}
|
||||
})(map)
|
||||
}
|
||||
|
||||
defineProps(colors, init());
|
45
node_modules/colors/lib/custom/trap.js
generated
vendored
Normal file
45
node_modules/colors/lib/custom/trap.js
generated
vendored
Normal file
@ -0,0 +1,45 @@
|
||||
module['exports'] = function runTheTrap (text, options) {
|
||||
var result = "";
|
||||
text = text || "Run the trap, drop the bass";
|
||||
text = text.split('');
|
||||
var trap = {
|
||||
a: ["\u0040", "\u0104", "\u023a", "\u0245", "\u0394", "\u039b", "\u0414"],
|
||||
b: ["\u00df", "\u0181", "\u0243", "\u026e", "\u03b2", "\u0e3f"],
|
||||
c: ["\u00a9", "\u023b", "\u03fe"],
|
||||
d: ["\u00d0", "\u018a", "\u0500" , "\u0501" ,"\u0502", "\u0503"],
|
||||
e: ["\u00cb", "\u0115", "\u018e", "\u0258", "\u03a3", "\u03be", "\u04bc", "\u0a6c"],
|
||||
f: ["\u04fa"],
|
||||
g: ["\u0262"],
|
||||
h: ["\u0126", "\u0195", "\u04a2", "\u04ba", "\u04c7", "\u050a"],
|
||||
i: ["\u0f0f"],
|
||||
j: ["\u0134"],
|
||||
k: ["\u0138", "\u04a0", "\u04c3", "\u051e"],
|
||||
l: ["\u0139"],
|
||||
m: ["\u028d", "\u04cd", "\u04ce", "\u0520", "\u0521", "\u0d69"],
|
||||
n: ["\u00d1", "\u014b", "\u019d", "\u0376", "\u03a0", "\u048a"],
|
||||
o: ["\u00d8", "\u00f5", "\u00f8", "\u01fe", "\u0298", "\u047a", "\u05dd", "\u06dd", "\u0e4f"],
|
||||
p: ["\u01f7", "\u048e"],
|
||||
q: ["\u09cd"],
|
||||
r: ["\u00ae", "\u01a6", "\u0210", "\u024c", "\u0280", "\u042f"],
|
||||
s: ["\u00a7", "\u03de", "\u03df", "\u03e8"],
|
||||
t: ["\u0141", "\u0166", "\u0373"],
|
||||
u: ["\u01b1", "\u054d"],
|
||||
v: ["\u05d8"],
|
||||
w: ["\u0428", "\u0460", "\u047c", "\u0d70"],
|
||||
x: ["\u04b2", "\u04fe", "\u04fc", "\u04fd"],
|
||||
y: ["\u00a5", "\u04b0", "\u04cb"],
|
||||
z: ["\u01b5", "\u0240"]
|
||||
}
|
||||
text.forEach(function(c){
|
||||
c = c.toLowerCase();
|
||||
var chars = trap[c] || [" "];
|
||||
var rand = Math.floor(Math.random() * chars.length);
|
||||
if (typeof trap[c] !== "undefined") {
|
||||
result += trap[c][rand];
|
||||
} else {
|
||||
result += c;
|
||||
}
|
||||
});
|
||||
return result;
|
||||
|
||||
}
|
104
node_modules/colors/lib/custom/zalgo.js
generated
vendored
Normal file
104
node_modules/colors/lib/custom/zalgo.js
generated
vendored
Normal file
@ -0,0 +1,104 @@
|
||||
// please no
|
||||
module['exports'] = function zalgo(text, options) {
|
||||
text = text || " he is here ";
|
||||
var soul = {
|
||||
"up" : [
|
||||
'̍', '̎', '̄', '̅',
|
||||
'̿', '̑', '̆', '̐',
|
||||
'͒', '͗', '͑', '̇',
|
||||
'̈', '̊', '͂', '̓',
|
||||
'̈', '͊', '͋', '͌',
|
||||
'̃', '̂', '̌', '͐',
|
||||
'̀', '́', '̋', '̏',
|
||||
'̒', '̓', '̔', '̽',
|
||||
'̉', 'ͣ', 'ͤ', 'ͥ',
|
||||
'ͦ', 'ͧ', 'ͨ', 'ͩ',
|
||||
'ͪ', 'ͫ', 'ͬ', 'ͭ',
|
||||
'ͮ', 'ͯ', '̾', '͛',
|
||||
'͆', '̚'
|
||||
],
|
||||
"down" : [
|
||||
'̖', '̗', '̘', '̙',
|
||||
'̜', '̝', '̞', '̟',
|
||||
'̠', '̤', '̥', '̦',
|
||||
'̩', '̪', '̫', '̬',
|
||||
'̭', '̮', '̯', '̰',
|
||||
'̱', '̲', '̳', '̹',
|
||||
'̺', '̻', '̼', 'ͅ',
|
||||
'͇', '͈', '͉', '͍',
|
||||
'͎', '͓', '͔', '͕',
|
||||
'͖', '͙', '͚', '̣'
|
||||
],
|
||||
"mid" : [
|
||||
'̕', '̛', '̀', '́',
|
||||
'͘', '̡', '̢', '̧',
|
||||
'̨', '̴', '̵', '̶',
|
||||
'͜', '͝', '͞',
|
||||
'͟', '͠', '͢', '̸',
|
||||
'̷', '͡', ' ҉'
|
||||
]
|
||||
},
|
||||
all = [].concat(soul.up, soul.down, soul.mid),
|
||||
zalgo = {};
|
||||
|
||||
function randomNumber(range) {
|
||||
var r = Math.floor(Math.random() * range);
|
||||
return r;
|
||||
}
|
||||
|
||||
function is_char(character) {
|
||||
var bool = false;
|
||||
all.filter(function (i) {
|
||||
bool = (i === character);
|
||||
});
|
||||
return bool;
|
||||
}
|
||||
|
||||
|
||||
function heComes(text, options) {
|
||||
var result = '', counts, l;
|
||||
options = options || {};
|
||||
options["up"] = typeof options["up"] !== 'undefined' ? options["up"] : true;
|
||||
options["mid"] = typeof options["mid"] !== 'undefined' ? options["mid"] : true;
|
||||
options["down"] = typeof options["down"] !== 'undefined' ? options["down"] : true;
|
||||
options["size"] = typeof options["size"] !== 'undefined' ? options["size"] : "maxi";
|
||||
text = text.split('');
|
||||
for (l in text) {
|
||||
if (is_char(l)) {
|
||||
continue;
|
||||
}
|
||||
result = result + text[l];
|
||||
counts = {"up" : 0, "down" : 0, "mid" : 0};
|
||||
switch (options.size) {
|
||||
case 'mini':
|
||||
counts.up = randomNumber(8);
|
||||
counts.mid = randomNumber(2);
|
||||
counts.down = randomNumber(8);
|
||||
break;
|
||||
case 'maxi':
|
||||
counts.up = randomNumber(16) + 3;
|
||||
counts.mid = randomNumber(4) + 1;
|
||||
counts.down = randomNumber(64) + 3;
|
||||
break;
|
||||
default:
|
||||
counts.up = randomNumber(8) + 1;
|
||||
counts.mid = randomNumber(6) / 2;
|
||||
counts.down = randomNumber(8) + 1;
|
||||
break;
|
||||
}
|
||||
|
||||
var arr = ["up", "mid", "down"];
|
||||
for (var d in arr) {
|
||||
var index = arr[d];
|
||||
for (var i = 0 ; i <= counts[index]; i++) {
|
||||
if (options[index]) {
|
||||
result = result + soul[index][randomNumber(soul[index].length)];
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
return result;
|
||||
}
|
||||
// don't summon him
|
||||
return heComes(text, options);
|
||||
}
|
113
node_modules/colors/lib/extendStringPrototype.js
generated
vendored
Normal file
113
node_modules/colors/lib/extendStringPrototype.js
generated
vendored
Normal file
@ -0,0 +1,113 @@
|
||||
var colors = require('./colors');
|
||||
|
||||
module['exports'] = function () {
|
||||
|
||||
//
|
||||
// Extends prototype of native string object to allow for "foo".red syntax
|
||||
//
|
||||
var addProperty = function (color, func) {
|
||||
String.prototype.__defineGetter__(color, func);
|
||||
};
|
||||
|
||||
var sequencer = function sequencer (map, str) {
|
||||
return function () {
|
||||
var exploded = this.split(""), i = 0;
|
||||
exploded = exploded.map(map);
|
||||
return exploded.join("");
|
||||
}
|
||||
};
|
||||
|
||||
addProperty('strip', function () {
|
||||
return colors.strip(this);
|
||||
});
|
||||
|
||||
addProperty('stripColors', function () {
|
||||
return colors.strip(this);
|
||||
});
|
||||
|
||||
addProperty("trap", function(){
|
||||
return colors.trap(this);
|
||||
});
|
||||
|
||||
addProperty("zalgo", function(){
|
||||
return colors.zalgo(this);
|
||||
});
|
||||
|
||||
addProperty("zebra", function(){
|
||||
return colors.zebra(this);
|
||||
});
|
||||
|
||||
addProperty("rainbow", function(){
|
||||
return colors.rainbow(this);
|
||||
});
|
||||
|
||||
addProperty("random", function(){
|
||||
return colors.random(this);
|
||||
});
|
||||
|
||||
addProperty("america", function(){
|
||||
return colors.america(this);
|
||||
});
|
||||
|
||||
//
|
||||
// Iterate through all default styles and colors
|
||||
//
|
||||
var x = Object.keys(colors.styles);
|
||||
x.forEach(function (style) {
|
||||
addProperty(style, function () {
|
||||
return colors.stylize(this, style);
|
||||
});
|
||||
});
|
||||
|
||||
function applyTheme(theme) {
|
||||
//
|
||||
// Remark: This is a list of methods that exist
|
||||
// on String that you should not overwrite.
|
||||
//
|
||||
var stringPrototypeBlacklist = [
|
||||
'__defineGetter__', '__defineSetter__', '__lookupGetter__', '__lookupSetter__', 'charAt', 'constructor',
|
||||
'hasOwnProperty', 'isPrototypeOf', 'propertyIsEnumerable', 'toLocaleString', 'toString', 'valueOf', 'charCodeAt',
|
||||
'indexOf', 'lastIndexof', 'length', 'localeCompare', 'match', 'replace', 'search', 'slice', 'split', 'substring',
|
||||
'toLocaleLowerCase', 'toLocaleUpperCase', 'toLowerCase', 'toUpperCase', 'trim', 'trimLeft', 'trimRight'
|
||||
];
|
||||
|
||||
Object.keys(theme).forEach(function (prop) {
|
||||
if (stringPrototypeBlacklist.indexOf(prop) !== -1) {
|
||||
console.log('warn: '.red + ('String.prototype' + prop).magenta + ' is probably something you don\'t want to override. Ignoring style name');
|
||||
}
|
||||
else {
|
||||
if (typeof(theme[prop]) === 'string') {
|
||||
colors[prop] = colors[theme[prop]];
|
||||
addProperty(prop, function () {
|
||||
return colors[theme[prop]](this);
|
||||
});
|
||||
}
|
||||
else {
|
||||
addProperty(prop, function () {
|
||||
var ret = this;
|
||||
for (var t = 0; t < theme[prop].length; t++) {
|
||||
ret = colors[theme[prop][t]](ret);
|
||||
}
|
||||
return ret;
|
||||
});
|
||||
}
|
||||
}
|
||||
});
|
||||
}
|
||||
|
||||
colors.setTheme = function (theme) {
|
||||
if (typeof theme === 'string') {
|
||||
try {
|
||||
colors.themes[theme] = require(theme);
|
||||
applyTheme(colors.themes[theme]);
|
||||
return colors.themes[theme];
|
||||
} catch (err) {
|
||||
console.log(err);
|
||||
return err;
|
||||
}
|
||||
} else {
|
||||
applyTheme(theme);
|
||||
}
|
||||
};
|
||||
|
||||
};
|
12
node_modules/colors/lib/index.js
generated
vendored
Normal file
12
node_modules/colors/lib/index.js
generated
vendored
Normal file
@ -0,0 +1,12 @@
|
||||
var colors = require('./colors');
|
||||
module['exports'] = colors;
|
||||
|
||||
// Remark: By default, colors will add style properties to String.prototype
|
||||
//
|
||||
// If you don't wish to extend String.prototype you can do this instead and native String will not be touched
|
||||
//
|
||||
// var colors = require('colors/safe);
|
||||
// colors.red("foo")
|
||||
//
|
||||
//
|
||||
require('./extendStringPrototype')();
|
12
node_modules/colors/lib/maps/america.js
generated
vendored
Normal file
12
node_modules/colors/lib/maps/america.js
generated
vendored
Normal file
@ -0,0 +1,12 @@
|
||||
var colors = require('../colors');
|
||||
|
||||
module['exports'] = (function() {
|
||||
return function (letter, i, exploded) {
|
||||
if(letter === " ") return letter;
|
||||
switch(i%3) {
|
||||
case 0: return colors.red(letter);
|
||||
case 1: return colors.white(letter)
|
||||
case 2: return colors.blue(letter)
|
||||
}
|
||||
}
|
||||
})();
|
13
node_modules/colors/lib/maps/rainbow.js
generated
vendored
Normal file
13
node_modules/colors/lib/maps/rainbow.js
generated
vendored
Normal file
@ -0,0 +1,13 @@
|
||||
var colors = require('../colors');
|
||||
|
||||
module['exports'] = (function () {
|
||||
var rainbowColors = ['red', 'yellow', 'green', 'blue', 'magenta']; //RoY G BiV
|
||||
return function (letter, i, exploded) {
|
||||
if (letter === " ") {
|
||||
return letter;
|
||||
} else {
|
||||
return colors[rainbowColors[i++ % rainbowColors.length]](letter);
|
||||
}
|
||||
};
|
||||
})();
|
||||
|
8
node_modules/colors/lib/maps/random.js
generated
vendored
Normal file
8
node_modules/colors/lib/maps/random.js
generated
vendored
Normal file
@ -0,0 +1,8 @@
|
||||
var colors = require('../colors');
|
||||
|
||||
module['exports'] = (function () {
|
||||
var available = ['underline', 'inverse', 'grey', 'yellow', 'red', 'green', 'blue', 'white', 'cyan', 'magenta'];
|
||||
return function(letter, i, exploded) {
|
||||
return letter === " " ? letter : colors[available[Math.round(Math.random() * (available.length - 1))]](letter);
|
||||
};
|
||||
})();
|
5
node_modules/colors/lib/maps/zebra.js
generated
vendored
Normal file
5
node_modules/colors/lib/maps/zebra.js
generated
vendored
Normal file
@ -0,0 +1,5 @@
|
||||
var colors = require('../colors');
|
||||
|
||||
module['exports'] = function (letter, i, exploded) {
|
||||
return i % 2 === 0 ? letter : colors.inverse(letter);
|
||||
};
|
77
node_modules/colors/lib/styles.js
generated
vendored
Normal file
77
node_modules/colors/lib/styles.js
generated
vendored
Normal file
@ -0,0 +1,77 @@
|
||||
/*
|
||||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com)
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
||||
|
||||
*/
|
||||
|
||||
var styles = {};
|
||||
module['exports'] = styles;
|
||||
|
||||
var codes = {
|
||||
reset: [0, 0],
|
||||
|
||||
bold: [1, 22],
|
||||
dim: [2, 22],
|
||||
italic: [3, 23],
|
||||
underline: [4, 24],
|
||||
inverse: [7, 27],
|
||||
hidden: [8, 28],
|
||||
strikethrough: [9, 29],
|
||||
|
||||
black: [30, 39],
|
||||
red: [31, 39],
|
||||
green: [32, 39],
|
||||
yellow: [33, 39],
|
||||
blue: [34, 39],
|
||||
magenta: [35, 39],
|
||||
cyan: [36, 39],
|
||||
white: [37, 39],
|
||||
gray: [90, 39],
|
||||
grey: [90, 39],
|
||||
|
||||
bgBlack: [40, 49],
|
||||
bgRed: [41, 49],
|
||||
bgGreen: [42, 49],
|
||||
bgYellow: [43, 49],
|
||||
bgBlue: [44, 49],
|
||||
bgMagenta: [45, 49],
|
||||
bgCyan: [46, 49],
|
||||
bgWhite: [47, 49],
|
||||
|
||||
// legacy styles for colors pre v1.0.0
|
||||
blackBG: [40, 49],
|
||||
redBG: [41, 49],
|
||||
greenBG: [42, 49],
|
||||
yellowBG: [43, 49],
|
||||
blueBG: [44, 49],
|
||||
magentaBG: [45, 49],
|
||||
cyanBG: [46, 49],
|
||||
whiteBG: [47, 49]
|
||||
|
||||
};
|
||||
|
||||
Object.keys(codes).forEach(function (key) {
|
||||
var val = codes[key];
|
||||
var style = styles[key] = [];
|
||||
style.open = '\u001b[' + val[0] + 'm';
|
||||
style.close = '\u001b[' + val[1] + 'm';
|
||||
});
|
61
node_modules/colors/lib/system/supports-colors.js
generated
vendored
Normal file
61
node_modules/colors/lib/system/supports-colors.js
generated
vendored
Normal file
@ -0,0 +1,61 @@
|
||||
/*
|
||||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com)
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
||||
|
||||
*/
|
||||
|
||||
var argv = process.argv;
|
||||
|
||||
module.exports = (function () {
|
||||
if (argv.indexOf('--no-color') !== -1 ||
|
||||
argv.indexOf('--color=false') !== -1) {
|
||||
return false;
|
||||
}
|
||||
|
||||
if (argv.indexOf('--color') !== -1 ||
|
||||
argv.indexOf('--color=true') !== -1 ||
|
||||
argv.indexOf('--color=always') !== -1) {
|
||||
return true;
|
||||
}
|
||||
|
||||
if (process.stdout && !process.stdout.isTTY) {
|
||||
return false;
|
||||
}
|
||||
|
||||
if (process.platform === 'win32') {
|
||||
return true;
|
||||
}
|
||||
|
||||
if ('COLORTERM' in process.env) {
|
||||
return true;
|
||||
}
|
||||
|
||||
if (process.env.TERM === 'dumb') {
|
||||
return false;
|
||||
}
|
||||
|
||||
if (/^screen|^xterm|^vt100|color|ansi|cygwin|linux/i.test(process.env.TERM)) {
|
||||
return true;
|
||||
}
|
||||
|
||||
return false;
|
||||
})();
|
58
node_modules/colors/package.json
generated
vendored
Normal file
58
node_modules/colors/package.json
generated
vendored
Normal file
@ -0,0 +1,58 @@
|
||||
{
|
||||
"name": "colors",
|
||||
"description": "get colors in your node.js console",
|
||||
"version": "1.1.2",
|
||||
"author": {
|
||||
"name": "Marak Squires"
|
||||
},
|
||||
"homepage": "https://github.com/Marak/colors.js",
|
||||
"bugs": {
|
||||
"url": "https://github.com/Marak/colors.js/issues"
|
||||
},
|
||||
"keywords": [
|
||||
"ansi",
|
||||
"terminal",
|
||||
"colors"
|
||||
],
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "http://github.com/Marak/colors.js.git"
|
||||
},
|
||||
"license": "MIT",
|
||||
"scripts": {
|
||||
"test": "node tests/basic-test.js && node tests/safe-test.js"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=0.1.90"
|
||||
},
|
||||
"main": "lib",
|
||||
"files": [
|
||||
"examples",
|
||||
"lib",
|
||||
"LICENSE",
|
||||
"safe.js",
|
||||
"themes"
|
||||
],
|
||||
"gitHead": "8bf2ad9fa695dcb30b7e9fd83691b139fd6655c4",
|
||||
"_id": "colors@1.1.2",
|
||||
"_shasum": "168a4701756b6a7f51a12ce0c97bfa28c084ed63",
|
||||
"_from": "colors@",
|
||||
"_npmVersion": "2.1.8",
|
||||
"_nodeVersion": "0.11.13",
|
||||
"_npmUser": {
|
||||
"name": "marak",
|
||||
"email": "marak.squires@gmail.com"
|
||||
},
|
||||
"maintainers": [
|
||||
{
|
||||
"name": "marak",
|
||||
"email": "marak.squires@gmail.com"
|
||||
}
|
||||
],
|
||||
"dist": {
|
||||
"shasum": "168a4701756b6a7f51a12ce0c97bfa28c084ed63",
|
||||
"tarball": "http://registry.npmjs.org/colors/-/colors-1.1.2.tgz"
|
||||
},
|
||||
"directories": {},
|
||||
"_resolved": "https://registry.npmjs.org/colors/-/colors-1.1.2.tgz"
|
||||
}
|
9
node_modules/colors/safe.js
generated
vendored
Normal file
9
node_modules/colors/safe.js
generated
vendored
Normal file
@ -0,0 +1,9 @@
|
||||
//
|
||||
// Remark: Requiring this file will use the "safe" colors API which will not touch String.prototype
|
||||
//
|
||||
// var colors = require('colors/safe);
|
||||
// colors.red("foo")
|
||||
//
|
||||
//
|
||||
var colors = require('./lib/colors');
|
||||
module['exports'] = colors;
|
12
node_modules/colors/themes/generic-logging.js
generated
vendored
Normal file
12
node_modules/colors/themes/generic-logging.js
generated
vendored
Normal file
@ -0,0 +1,12 @@
|
||||
module['exports'] = {
|
||||
silly: 'rainbow',
|
||||
input: 'grey',
|
||||
verbose: 'cyan',
|
||||
prompt: 'grey',
|
||||
info: 'green',
|
||||
data: 'grey',
|
||||
help: 'cyan',
|
||||
warn: 'yellow',
|
||||
debug: 'blue',
|
||||
error: 'red'
|
||||
};
|
3062
node_modules/express/History.md
generated
vendored
Normal file
3062
node_modules/express/History.md
generated
vendored
Normal file
File diff suppressed because it is too large
Load Diff
24
node_modules/express/LICENSE
generated
vendored
Normal file
24
node_modules/express/LICENSE
generated
vendored
Normal file
@ -0,0 +1,24 @@
|
||||
(The MIT License)
|
||||
|
||||
Copyright (c) 2009-2014 TJ Holowaychuk <tj@vision-media.ca>
|
||||
Copyright (c) 2013-2014 Roman Shtylman <shtylman+expressjs@gmail.com>
|
||||
Copyright (c) 2014-2015 Douglas Christopher Wilson <doug@somethingdoug.com>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of this software and associated documentation files (the
|
||||
'Software'), to deal in the Software without restriction, including
|
||||
without limitation the rights to use, copy, modify, merge, publish,
|
||||
distribute, sublicense, and/or sell copies of the Software, and to
|
||||
permit persons to whom the Software is furnished to do so, subject to
|
||||
the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
|
||||
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
|
||||
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
|
||||
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
|
||||
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
138
node_modules/express/Readme.md
generated
vendored
Normal file
138
node_modules/express/Readme.md
generated
vendored
Normal file
@ -0,0 +1,138 @@
|
||||
[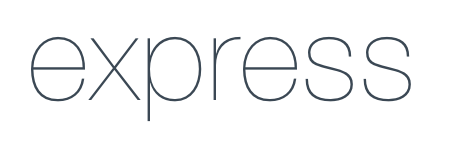](http://expressjs.com/)
|
||||
|
||||
Fast, unopinionated, minimalist web framework for [node](http://nodejs.org).
|
||||
|
||||
[![NPM Version][npm-image]][npm-url]
|
||||
[![NPM Downloads][downloads-image]][downloads-url]
|
||||
[![Linux Build][travis-image]][travis-url]
|
||||
[![Windows Build][appveyor-image]][appveyor-url]
|
||||
[![Test Coverage][coveralls-image]][coveralls-url]
|
||||
|
||||
```js
|
||||
var express = require('express')
|
||||
var app = express()
|
||||
|
||||
app.get('/', function (req, res) {
|
||||
res.send('Hello World')
|
||||
})
|
||||
|
||||
app.listen(3000)
|
||||
```
|
||||
|
||||
## Installation
|
||||
|
||||
```bash
|
||||
$ npm install express
|
||||
```
|
||||
|
||||
## Features
|
||||
|
||||
* Robust routing
|
||||
* Focus on high performance
|
||||
* Super-high test coverage
|
||||
* HTTP helpers (redirection, caching, etc)
|
||||
* View system supporting 14+ template engines
|
||||
* Content negotiation
|
||||
* Executable for generating applications quickly
|
||||
|
||||
## Docs & Community
|
||||
|
||||
* [#express](https://webchat.freenode.net/?channels=express) on freenode IRC
|
||||
* [Github Organization](https://github.com/expressjs) for Official Middleware & Modules
|
||||
* [Google Group](https://groups.google.com/group/express-js) for discussion
|
||||
* [Gitter](https://gitter.im/expressjs/express) for support and discussion
|
||||
* [Русскоязычная документация](http://jsman.ru/express/)
|
||||
|
||||
###Security Issues
|
||||
|
||||
If you discover a security vulnerability in Express, please see [Security Policies and Procedures](Security.md).
|
||||
|
||||
## Quick Start
|
||||
|
||||
The quickest way to get started with express is to utilize the executable [`express(1)`](https://github.com/expressjs/generator) to generate an application as shown below:
|
||||
|
||||
Install the executable. The executable's major version will match Express's:
|
||||
|
||||
```bash
|
||||
$ npm install -g express-generator@4
|
||||
```
|
||||
|
||||
Create the app:
|
||||
|
||||
```bash
|
||||
$ express /tmp/foo && cd /tmp/foo
|
||||
```
|
||||
|
||||
Install dependencies:
|
||||
|
||||
```bash
|
||||
$ npm install
|
||||
```
|
||||
|
||||
Start the server:
|
||||
|
||||
```bash
|
||||
$ npm start
|
||||
```
|
||||
|
||||
## Philosophy
|
||||
|
||||
The Express philosophy is to provide small, robust tooling for HTTP servers, making
|
||||
it a great solution for single page applications, web sites, hybrids, or public
|
||||
HTTP APIs.
|
||||
|
||||
Express does not force you to use any specific ORM or template engine. With support for over
|
||||
14 template engines via [Consolidate.js](https://github.com/tj/consolidate.js),
|
||||
you can quickly craft your perfect framework.
|
||||
|
||||
## Examples
|
||||
|
||||
To view the examples, clone the Express repo and install the dependencies:
|
||||
|
||||
```bash
|
||||
$ git clone git://github.com/expressjs/express.git --depth 1
|
||||
$ cd express
|
||||
$ npm install
|
||||
```
|
||||
|
||||
Then run whichever example you want:
|
||||
|
||||
```bash
|
||||
$ node examples/content-negotiation
|
||||
```
|
||||
|
||||
## Tests
|
||||
|
||||
To run the test suite, first install the dependencies, then run `npm test`:
|
||||
|
||||
```bash
|
||||
$ npm install
|
||||
$ npm test
|
||||
```
|
||||
|
||||
## People
|
||||
|
||||
The original author of Express is [TJ Holowaychuk](https://github.com/tj) [![TJ's Gratipay][gratipay-image-visionmedia]][gratipay-url-visionmedia]
|
||||
|
||||
The current lead maintainer is [Douglas Christopher Wilson](https://github.com/dougwilson) [![Doug's Gratipay][gratipay-image-dougwilson]][gratipay-url-dougwilson]
|
||||
|
||||
[List of all contributors](https://github.com/expressjs/express/graphs/contributors)
|
||||
|
||||
## License
|
||||
|
||||
[MIT](LICENSE)
|
||||
|
||||
[npm-image]: https://img.shields.io/npm/v/express.svg
|
||||
[npm-url]: https://npmjs.org/package/express
|
||||
[downloads-image]: https://img.shields.io/npm/dm/express.svg
|
||||
[downloads-url]: https://npmjs.org/package/express
|
||||
[travis-image]: https://img.shields.io/travis/expressjs/express/master.svg?label=linux
|
||||
[travis-url]: https://travis-ci.org/expressjs/express
|
||||
[appveyor-image]: https://img.shields.io/appveyor/ci/dougwilson/express/master.svg?label=windows
|
||||
[appveyor-url]: https://ci.appveyor.com/project/dougwilson/express
|
||||
[coveralls-image]: https://img.shields.io/coveralls/expressjs/express/master.svg
|
||||
[coveralls-url]: https://coveralls.io/r/expressjs/express?branch=master
|
||||
[gratipay-image-visionmedia]: https://img.shields.io/gratipay/visionmedia.svg
|
||||
[gratipay-url-visionmedia]: https://gratipay.com/visionmedia/
|
||||
[gratipay-image-dougwilson]: https://img.shields.io/gratipay/dougwilson.svg
|
||||
[gratipay-url-dougwilson]: https://gratipay.com/dougwilson/
|
11
node_modules/express/index.js
generated
vendored
Normal file
11
node_modules/express/index.js
generated
vendored
Normal file
@ -0,0 +1,11 @@
|
||||
/*!
|
||||
* express
|
||||
* Copyright(c) 2009-2013 TJ Holowaychuk
|
||||
* Copyright(c) 2013 Roman Shtylman
|
||||
* Copyright(c) 2014-2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict';
|
||||
|
||||
module.exports = require('./lib/express');
|
643
node_modules/express/lib/application.js
generated
vendored
Normal file
643
node_modules/express/lib/application.js
generated
vendored
Normal file
@ -0,0 +1,643 @@
|
||||
/*!
|
||||
* express
|
||||
* Copyright(c) 2009-2013 TJ Holowaychuk
|
||||
* Copyright(c) 2013 Roman Shtylman
|
||||
* Copyright(c) 2014-2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict';
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var finalhandler = require('finalhandler');
|
||||
var Router = require('./router');
|
||||
var methods = require('methods');
|
||||
var middleware = require('./middleware/init');
|
||||
var query = require('./middleware/query');
|
||||
var debug = require('debug')('express:application');
|
||||
var View = require('./view');
|
||||
var http = require('http');
|
||||
var compileETag = require('./utils').compileETag;
|
||||
var compileQueryParser = require('./utils').compileQueryParser;
|
||||
var compileTrust = require('./utils').compileTrust;
|
||||
var deprecate = require('depd')('express');
|
||||
var flatten = require('array-flatten');
|
||||
var merge = require('utils-merge');
|
||||
var resolve = require('path').resolve;
|
||||
var slice = Array.prototype.slice;
|
||||
|
||||
/**
|
||||
* Application prototype.
|
||||
*/
|
||||
|
||||
var app = exports = module.exports = {};
|
||||
|
||||
/**
|
||||
* Variable for trust proxy inheritance back-compat
|
||||
* @private
|
||||
*/
|
||||
|
||||
var trustProxyDefaultSymbol = '@@symbol:trust_proxy_default';
|
||||
|
||||
/**
|
||||
* Initialize the server.
|
||||
*
|
||||
* - setup default configuration
|
||||
* - setup default middleware
|
||||
* - setup route reflection methods
|
||||
*
|
||||
* @private
|
||||
*/
|
||||
|
||||
app.init = function init() {
|
||||
this.cache = {};
|
||||
this.engines = {};
|
||||
this.settings = {};
|
||||
|
||||
this.defaultConfiguration();
|
||||
};
|
||||
|
||||
/**
|
||||
* Initialize application configuration.
|
||||
* @private
|
||||
*/
|
||||
|
||||
app.defaultConfiguration = function defaultConfiguration() {
|
||||
var env = process.env.NODE_ENV || 'development';
|
||||
|
||||
// default settings
|
||||
this.enable('x-powered-by');
|
||||
this.set('etag', 'weak');
|
||||
this.set('env', env);
|
||||
this.set('query parser', 'extended');
|
||||
this.set('subdomain offset', 2);
|
||||
this.set('trust proxy', false);
|
||||
|
||||
// trust proxy inherit back-compat
|
||||
Object.defineProperty(this.settings, trustProxyDefaultSymbol, {
|
||||
configurable: true,
|
||||
value: true
|
||||
});
|
||||
|
||||
debug('booting in %s mode', env);
|
||||
|
||||
this.on('mount', function onmount(parent) {
|
||||
// inherit trust proxy
|
||||
if (this.settings[trustProxyDefaultSymbol] === true
|
||||
&& typeof parent.settings['trust proxy fn'] === 'function') {
|
||||
delete this.settings['trust proxy'];
|
||||
delete this.settings['trust proxy fn'];
|
||||
}
|
||||
|
||||
// inherit protos
|
||||
this.request.__proto__ = parent.request;
|
||||
this.response.__proto__ = parent.response;
|
||||
this.engines.__proto__ = parent.engines;
|
||||
this.settings.__proto__ = parent.settings;
|
||||
});
|
||||
|
||||
// setup locals
|
||||
this.locals = Object.create(null);
|
||||
|
||||
// top-most app is mounted at /
|
||||
this.mountpath = '/';
|
||||
|
||||
// default locals
|
||||
this.locals.settings = this.settings;
|
||||
|
||||
// default configuration
|
||||
this.set('view', View);
|
||||
this.set('views', resolve('views'));
|
||||
this.set('jsonp callback name', 'callback');
|
||||
|
||||
if (env === 'production') {
|
||||
this.enable('view cache');
|
||||
}
|
||||
|
||||
Object.defineProperty(this, 'router', {
|
||||
get: function() {
|
||||
throw new Error('\'app.router\' is deprecated!\nPlease see the 3.x to 4.x migration guide for details on how to update your app.');
|
||||
}
|
||||
});
|
||||
};
|
||||
|
||||
/**
|
||||
* lazily adds the base router if it has not yet been added.
|
||||
*
|
||||
* We cannot add the base router in the defaultConfiguration because
|
||||
* it reads app settings which might be set after that has run.
|
||||
*
|
||||
* @private
|
||||
*/
|
||||
app.lazyrouter = function lazyrouter() {
|
||||
if (!this._router) {
|
||||
this._router = new Router({
|
||||
caseSensitive: this.enabled('case sensitive routing'),
|
||||
strict: this.enabled('strict routing')
|
||||
});
|
||||
|
||||
this._router.use(query(this.get('query parser fn')));
|
||||
this._router.use(middleware.init(this));
|
||||
}
|
||||
};
|
||||
|
||||
/**
|
||||
* Dispatch a req, res pair into the application. Starts pipeline processing.
|
||||
*
|
||||
* If no callback is provided, then default error handlers will respond
|
||||
* in the event of an error bubbling through the stack.
|
||||
*
|
||||
* @private
|
||||
*/
|
||||
|
||||
app.handle = function handle(req, res, callback) {
|
||||
var router = this._router;
|
||||
|
||||
// final handler
|
||||
var done = callback || finalhandler(req, res, {
|
||||
env: this.get('env'),
|
||||
onerror: logerror.bind(this)
|
||||
});
|
||||
|
||||
// no routes
|
||||
if (!router) {
|
||||
debug('no routes defined on app');
|
||||
done();
|
||||
return;
|
||||
}
|
||||
|
||||
router.handle(req, res, done);
|
||||
};
|
||||
|
||||
/**
|
||||
* Proxy `Router#use()` to add middleware to the app router.
|
||||
* See Router#use() documentation for details.
|
||||
*
|
||||
* If the _fn_ parameter is an express app, then it will be
|
||||
* mounted at the _route_ specified.
|
||||
*
|
||||
* @public
|
||||
*/
|
||||
|
||||
app.use = function use(fn) {
|
||||
var offset = 0;
|
||||
var path = '/';
|
||||
|
||||
// default path to '/'
|
||||
// disambiguate app.use([fn])
|
||||
if (typeof fn !== 'function') {
|
||||
var arg = fn;
|
||||
|
||||
while (Array.isArray(arg) && arg.length !== 0) {
|
||||
arg = arg[0];
|
||||
}
|
||||
|
||||
// first arg is the path
|
||||
if (typeof arg !== 'function') {
|
||||
offset = 1;
|
||||
path = fn;
|
||||
}
|
||||
}
|
||||
|
||||
var fns = flatten(slice.call(arguments, offset));
|
||||
|
||||
if (fns.length === 0) {
|
||||
throw new TypeError('app.use() requires middleware functions');
|
||||
}
|
||||
|
||||
// setup router
|
||||
this.lazyrouter();
|
||||
var router = this._router;
|
||||
|
||||
fns.forEach(function (fn) {
|
||||
// non-express app
|
||||
if (!fn || !fn.handle || !fn.set) {
|
||||
return router.use(path, fn);
|
||||
}
|
||||
|
||||
debug('.use app under %s', path);
|
||||
fn.mountpath = path;
|
||||
fn.parent = this;
|
||||
|
||||
// restore .app property on req and res
|
||||
router.use(path, function mounted_app(req, res, next) {
|
||||
var orig = req.app;
|
||||
fn.handle(req, res, function (err) {
|
||||
req.__proto__ = orig.request;
|
||||
res.__proto__ = orig.response;
|
||||
next(err);
|
||||
});
|
||||
});
|
||||
|
||||
// mounted an app
|
||||
fn.emit('mount', this);
|
||||
}, this);
|
||||
|
||||
return this;
|
||||
};
|
||||
|
||||
/**
|
||||
* Proxy to the app `Router#route()`
|
||||
* Returns a new `Route` instance for the _path_.
|
||||
*
|
||||
* Routes are isolated middleware stacks for specific paths.
|
||||
* See the Route api docs for details.
|
||||
*
|
||||
* @public
|
||||
*/
|
||||
|
||||
app.route = function route(path) {
|
||||
this.lazyrouter();
|
||||
return this._router.route(path);
|
||||
};
|
||||
|
||||
/**
|
||||
* Register the given template engine callback `fn`
|
||||
* as `ext`.
|
||||
*
|
||||
* By default will `require()` the engine based on the
|
||||
* file extension. For example if you try to render
|
||||
* a "foo.jade" file Express will invoke the following internally:
|
||||
*
|
||||
* app.engine('jade', require('jade').__express);
|
||||
*
|
||||
* For engines that do not provide `.__express` out of the box,
|
||||
* or if you wish to "map" a different extension to the template engine
|
||||
* you may use this method. For example mapping the EJS template engine to
|
||||
* ".html" files:
|
||||
*
|
||||
* app.engine('html', require('ejs').renderFile);
|
||||
*
|
||||
* In this case EJS provides a `.renderFile()` method with
|
||||
* the same signature that Express expects: `(path, options, callback)`,
|
||||
* though note that it aliases this method as `ejs.__express` internally
|
||||
* so if you're using ".ejs" extensions you dont need to do anything.
|
||||
*
|
||||
* Some template engines do not follow this convention, the
|
||||
* [Consolidate.js](https://github.com/tj/consolidate.js)
|
||||
* library was created to map all of node's popular template
|
||||
* engines to follow this convention, thus allowing them to
|
||||
* work seamlessly within Express.
|
||||
*
|
||||
* @param {String} ext
|
||||
* @param {Function} fn
|
||||
* @return {app} for chaining
|
||||
* @public
|
||||
*/
|
||||
|
||||
app.engine = function engine(ext, fn) {
|
||||
if (typeof fn !== 'function') {
|
||||
throw new Error('callback function required');
|
||||
}
|
||||
|
||||
// get file extension
|
||||
var extension = ext[0] !== '.'
|
||||
? '.' + ext
|
||||
: ext;
|
||||
|
||||
// store engine
|
||||
this.engines[extension] = fn;
|
||||
|
||||
return this;
|
||||
};
|
||||
|
||||
/**
|
||||
* Proxy to `Router#param()` with one added api feature. The _name_ parameter
|
||||
* can be an array of names.
|
||||
*
|
||||
* See the Router#param() docs for more details.
|
||||
*
|
||||
* @param {String|Array} name
|
||||
* @param {Function} fn
|
||||
* @return {app} for chaining
|
||||
* @public
|
||||
*/
|
||||
|
||||
app.param = function param(name, fn) {
|
||||
this.lazyrouter();
|
||||
|
||||
if (Array.isArray(name)) {
|
||||
for (var i = 0; i < name.length; i++) {
|
||||
this.param(name[i], fn);
|
||||
}
|
||||
|
||||
return this;
|
||||
}
|
||||
|
||||
this._router.param(name, fn);
|
||||
|
||||
return this;
|
||||
};
|
||||
|
||||
/**
|
||||
* Assign `setting` to `val`, or return `setting`'s value.
|
||||
*
|
||||
* app.set('foo', 'bar');
|
||||
* app.get('foo');
|
||||
* // => "bar"
|
||||
*
|
||||
* Mounted servers inherit their parent server's settings.
|
||||
*
|
||||
* @param {String} setting
|
||||
* @param {*} [val]
|
||||
* @return {Server} for chaining
|
||||
* @public
|
||||
*/
|
||||
|
||||
app.set = function set(setting, val) {
|
||||
if (arguments.length === 1) {
|
||||
// app.get(setting)
|
||||
return this.settings[setting];
|
||||
}
|
||||
|
||||
debug('set "%s" to %o', setting, val);
|
||||
|
||||
// set value
|
||||
this.settings[setting] = val;
|
||||
|
||||
// trigger matched settings
|
||||
switch (setting) {
|
||||
case 'etag':
|
||||
this.set('etag fn', compileETag(val));
|
||||
break;
|
||||
case 'query parser':
|
||||
this.set('query parser fn', compileQueryParser(val));
|
||||
break;
|
||||
case 'trust proxy':
|
||||
this.set('trust proxy fn', compileTrust(val));
|
||||
|
||||
// trust proxy inherit back-compat
|
||||
Object.defineProperty(this.settings, trustProxyDefaultSymbol, {
|
||||
configurable: true,
|
||||
value: false
|
||||
});
|
||||
|
||||
break;
|
||||
}
|
||||
|
||||
return this;
|
||||
};
|
||||
|
||||
/**
|
||||
* Return the app's absolute pathname
|
||||
* based on the parent(s) that have
|
||||
* mounted it.
|
||||
*
|
||||
* For example if the application was
|
||||
* mounted as "/admin", which itself
|
||||
* was mounted as "/blog" then the
|
||||
* return value would be "/blog/admin".
|
||||
*
|
||||
* @return {String}
|
||||
* @private
|
||||
*/
|
||||
|
||||
app.path = function path() {
|
||||
return this.parent
|
||||
? this.parent.path() + this.mountpath
|
||||
: '';
|
||||
};
|
||||
|
||||
/**
|
||||
* Check if `setting` is enabled (truthy).
|
||||
*
|
||||
* app.enabled('foo')
|
||||
* // => false
|
||||
*
|
||||
* app.enable('foo')
|
||||
* app.enabled('foo')
|
||||
* // => true
|
||||
*
|
||||
* @param {String} setting
|
||||
* @return {Boolean}
|
||||
* @public
|
||||
*/
|
||||
|
||||
app.enabled = function enabled(setting) {
|
||||
return Boolean(this.set(setting));
|
||||
};
|
||||
|
||||
/**
|
||||
* Check if `setting` is disabled.
|
||||
*
|
||||
* app.disabled('foo')
|
||||
* // => true
|
||||
*
|
||||
* app.enable('foo')
|
||||
* app.disabled('foo')
|
||||
* // => false
|
||||
*
|
||||
* @param {String} setting
|
||||
* @return {Boolean}
|
||||
* @public
|
||||
*/
|
||||
|
||||
app.disabled = function disabled(setting) {
|
||||
return !this.set(setting);
|
||||
};
|
||||
|
||||
/**
|
||||
* Enable `setting`.
|
||||
*
|
||||
* @param {String} setting
|
||||
* @return {app} for chaining
|
||||
* @public
|
||||
*/
|
||||
|
||||
app.enable = function enable(setting) {
|
||||
return this.set(setting, true);
|
||||
};
|
||||
|
||||
/**
|
||||
* Disable `setting`.
|
||||
*
|
||||
* @param {String} setting
|
||||
* @return {app} for chaining
|
||||
* @public
|
||||
*/
|
||||
|
||||
app.disable = function disable(setting) {
|
||||
return this.set(setting, false);
|
||||
};
|
||||
|
||||
/**
|
||||
* Delegate `.VERB(...)` calls to `router.VERB(...)`.
|
||||
*/
|
||||
|
||||
methods.forEach(function(method){
|
||||
app[method] = function(path){
|
||||
if (method === 'get' && arguments.length === 1) {
|
||||
// app.get(setting)
|
||||
return this.set(path);
|
||||
}
|
||||
|
||||
this.lazyrouter();
|
||||
|
||||
var route = this._router.route(path);
|
||||
route[method].apply(route, slice.call(arguments, 1));
|
||||
return this;
|
||||
};
|
||||
});
|
||||
|
||||
/**
|
||||
* Special-cased "all" method, applying the given route `path`,
|
||||
* middleware, and callback to _every_ HTTP method.
|
||||
*
|
||||
* @param {String} path
|
||||
* @param {Function} ...
|
||||
* @return {app} for chaining
|
||||
* @public
|
||||
*/
|
||||
|
||||
app.all = function all(path) {
|
||||
this.lazyrouter();
|
||||
|
||||
var route = this._router.route(path);
|
||||
var args = slice.call(arguments, 1);
|
||||
|
||||
for (var i = 0; i < methods.length; i++) {
|
||||
route[methods[i]].apply(route, args);
|
||||
}
|
||||
|
||||
return this;
|
||||
};
|
||||
|
||||
// del -> delete alias
|
||||
|
||||
app.del = deprecate.function(app.delete, 'app.del: Use app.delete instead');
|
||||
|
||||
/**
|
||||
* Render the given view `name` name with `options`
|
||||
* and a callback accepting an error and the
|
||||
* rendered template string.
|
||||
*
|
||||
* Example:
|
||||
*
|
||||
* app.render('email', { name: 'Tobi' }, function(err, html){
|
||||
* // ...
|
||||
* })
|
||||
*
|
||||
* @param {String} name
|
||||
* @param {Object|Function} options or fn
|
||||
* @param {Function} callback
|
||||
* @public
|
||||
*/
|
||||
|
||||
app.render = function render(name, options, callback) {
|
||||
var cache = this.cache;
|
||||
var done = callback;
|
||||
var engines = this.engines;
|
||||
var opts = options;
|
||||
var renderOptions = {};
|
||||
var view;
|
||||
|
||||
// support callback function as second arg
|
||||
if (typeof options === 'function') {
|
||||
done = options;
|
||||
opts = {};
|
||||
}
|
||||
|
||||
// merge app.locals
|
||||
merge(renderOptions, this.locals);
|
||||
|
||||
// merge options._locals
|
||||
if (opts._locals) {
|
||||
merge(renderOptions, opts._locals);
|
||||
}
|
||||
|
||||
// merge options
|
||||
merge(renderOptions, opts);
|
||||
|
||||
// set .cache unless explicitly provided
|
||||
if (renderOptions.cache == null) {
|
||||
renderOptions.cache = this.enabled('view cache');
|
||||
}
|
||||
|
||||
// primed cache
|
||||
if (renderOptions.cache) {
|
||||
view = cache[name];
|
||||
}
|
||||
|
||||
// view
|
||||
if (!view) {
|
||||
var View = this.get('view');
|
||||
|
||||
view = new View(name, {
|
||||
defaultEngine: this.get('view engine'),
|
||||
root: this.get('views'),
|
||||
engines: engines
|
||||
});
|
||||
|
||||
if (!view.path) {
|
||||
var dirs = Array.isArray(view.root) && view.root.length > 1
|
||||
? 'directories "' + view.root.slice(0, -1).join('", "') + '" or "' + view.root[view.root.length - 1] + '"'
|
||||
: 'directory "' + view.root + '"'
|
||||
var err = new Error('Failed to lookup view "' + name + '" in views ' + dirs);
|
||||
err.view = view;
|
||||
return done(err);
|
||||
}
|
||||
|
||||
// prime the cache
|
||||
if (renderOptions.cache) {
|
||||
cache[name] = view;
|
||||
}
|
||||
}
|
||||
|
||||
// render
|
||||
tryRender(view, renderOptions, done);
|
||||
};
|
||||
|
||||
/**
|
||||
* Listen for connections.
|
||||
*
|
||||
* A node `http.Server` is returned, with this
|
||||
* application (which is a `Function`) as its
|
||||
* callback. If you wish to create both an HTTP
|
||||
* and HTTPS server you may do so with the "http"
|
||||
* and "https" modules as shown here:
|
||||
*
|
||||
* var http = require('http')
|
||||
* , https = require('https')
|
||||
* , express = require('express')
|
||||
* , app = express();
|
||||
*
|
||||
* http.createServer(app).listen(80);
|
||||
* https.createServer({ ... }, app).listen(443);
|
||||
*
|
||||
* @return {http.Server}
|
||||
* @public
|
||||
*/
|
||||
|
||||
app.listen = function listen() {
|
||||
var server = http.createServer(this);
|
||||
return server.listen.apply(server, arguments);
|
||||
};
|
||||
|
||||
/**
|
||||
* Log error using console.error.
|
||||
*
|
||||
* @param {Error} err
|
||||
* @private
|
||||
*/
|
||||
|
||||
function logerror(err) {
|
||||
/* istanbul ignore next */
|
||||
if (this.get('env') !== 'test') console.error(err.stack || err.toString());
|
||||
}
|
||||
|
||||
/**
|
||||
* Try rendering a view.
|
||||
* @private
|
||||
*/
|
||||
|
||||
function tryRender(view, options, callback) {
|
||||
try {
|
||||
view.render(options, callback);
|
||||
} catch (err) {
|
||||
callback(err);
|
||||
}
|
||||
}
|
103
node_modules/express/lib/express.js
generated
vendored
Normal file
103
node_modules/express/lib/express.js
generated
vendored
Normal file
@ -0,0 +1,103 @@
|
||||
/*!
|
||||
* express
|
||||
* Copyright(c) 2009-2013 TJ Holowaychuk
|
||||
* Copyright(c) 2013 Roman Shtylman
|
||||
* Copyright(c) 2014-2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict';
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
*/
|
||||
|
||||
var EventEmitter = require('events').EventEmitter;
|
||||
var mixin = require('merge-descriptors');
|
||||
var proto = require('./application');
|
||||
var Route = require('./router/route');
|
||||
var Router = require('./router');
|
||||
var req = require('./request');
|
||||
var res = require('./response');
|
||||
|
||||
/**
|
||||
* Expose `createApplication()`.
|
||||
*/
|
||||
|
||||
exports = module.exports = createApplication;
|
||||
|
||||
/**
|
||||
* Create an express application.
|
||||
*
|
||||
* @return {Function}
|
||||
* @api public
|
||||
*/
|
||||
|
||||
function createApplication() {
|
||||
var app = function(req, res, next) {
|
||||
app.handle(req, res, next);
|
||||
};
|
||||
|
||||
mixin(app, EventEmitter.prototype, false);
|
||||
mixin(app, proto, false);
|
||||
|
||||
app.request = { __proto__: req, app: app };
|
||||
app.response = { __proto__: res, app: app };
|
||||
app.init();
|
||||
return app;
|
||||
}
|
||||
|
||||
/**
|
||||
* Expose the prototypes.
|
||||
*/
|
||||
|
||||
exports.application = proto;
|
||||
exports.request = req;
|
||||
exports.response = res;
|
||||
|
||||
/**
|
||||
* Expose constructors.
|
||||
*/
|
||||
|
||||
exports.Route = Route;
|
||||
exports.Router = Router;
|
||||
|
||||
/**
|
||||
* Expose middleware
|
||||
*/
|
||||
|
||||
exports.query = require('./middleware/query');
|
||||
exports.static = require('serve-static');
|
||||
|
||||
/**
|
||||
* Replace removed middleware with an appropriate error message.
|
||||
*/
|
||||
|
||||
[
|
||||
'json',
|
||||
'urlencoded',
|
||||
'bodyParser',
|
||||
'compress',
|
||||
'cookieSession',
|
||||
'session',
|
||||
'logger',
|
||||
'cookieParser',
|
||||
'favicon',
|
||||
'responseTime',
|
||||
'errorHandler',
|
||||
'timeout',
|
||||
'methodOverride',
|
||||
'vhost',
|
||||
'csrf',
|
||||
'directory',
|
||||
'limit',
|
||||
'multipart',
|
||||
'staticCache',
|
||||
].forEach(function (name) {
|
||||
Object.defineProperty(exports, name, {
|
||||
get: function () {
|
||||
throw new Error('Most middleware (like ' + name + ') is no longer bundled with Express and must be installed separately. Please see https://github.com/senchalabs/connect#middleware.');
|
||||
},
|
||||
configurable: true
|
||||
});
|
||||
});
|
36
node_modules/express/lib/middleware/init.js
generated
vendored
Normal file
36
node_modules/express/lib/middleware/init.js
generated
vendored
Normal file
@ -0,0 +1,36 @@
|
||||
/*!
|
||||
* express
|
||||
* Copyright(c) 2009-2013 TJ Holowaychuk
|
||||
* Copyright(c) 2013 Roman Shtylman
|
||||
* Copyright(c) 2014-2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict';
|
||||
|
||||
/**
|
||||
* Initialization middleware, exposing the
|
||||
* request and response to each other, as well
|
||||
* as defaulting the X-Powered-By header field.
|
||||
*
|
||||
* @param {Function} app
|
||||
* @return {Function}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
exports.init = function(app){
|
||||
return function expressInit(req, res, next){
|
||||
if (app.enabled('x-powered-by')) res.setHeader('X-Powered-By', 'Express');
|
||||
req.res = res;
|
||||
res.req = req;
|
||||
req.next = next;
|
||||
|
||||
req.__proto__ = app.request;
|
||||
res.__proto__ = app.response;
|
||||
|
||||
res.locals = res.locals || Object.create(null);
|
||||
|
||||
next();
|
||||
};
|
||||
};
|
||||
|
51
node_modules/express/lib/middleware/query.js
generated
vendored
Normal file
51
node_modules/express/lib/middleware/query.js
generated
vendored
Normal file
@ -0,0 +1,51 @@
|
||||
/*!
|
||||
* express
|
||||
* Copyright(c) 2009-2013 TJ Holowaychuk
|
||||
* Copyright(c) 2013 Roman Shtylman
|
||||
* Copyright(c) 2014-2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict';
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
*/
|
||||
|
||||
var parseUrl = require('parseurl');
|
||||
var qs = require('qs');
|
||||
|
||||
/**
|
||||
* @param {Object} options
|
||||
* @return {Function}
|
||||
* @api public
|
||||
*/
|
||||
|
||||
module.exports = function query(options) {
|
||||
var opts = Object.create(options || null);
|
||||
var queryparse = qs.parse;
|
||||
|
||||
if (typeof options === 'function') {
|
||||
queryparse = options;
|
||||
opts = undefined;
|
||||
}
|
||||
|
||||
if (opts !== undefined) {
|
||||
if (opts.allowDots === undefined) {
|
||||
opts.allowDots = false;
|
||||
}
|
||||
|
||||
if (opts.allowPrototypes === undefined) {
|
||||
opts.allowPrototypes = true;
|
||||
}
|
||||
}
|
||||
|
||||
return function query(req, res, next){
|
||||
if (!req.query) {
|
||||
var val = parseUrl(req).query;
|
||||
req.query = queryparse(val, opts);
|
||||
}
|
||||
|
||||
next();
|
||||
};
|
||||
};
|
489
node_modules/express/lib/request.js
generated
vendored
Normal file
489
node_modules/express/lib/request.js
generated
vendored
Normal file
@ -0,0 +1,489 @@
|
||||
/*!
|
||||
* express
|
||||
* Copyright(c) 2009-2013 TJ Holowaychuk
|
||||
* Copyright(c) 2013 Roman Shtylman
|
||||
* Copyright(c) 2014-2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict';
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var accepts = require('accepts');
|
||||
var deprecate = require('depd')('express');
|
||||
var isIP = require('net').isIP;
|
||||
var typeis = require('type-is');
|
||||
var http = require('http');
|
||||
var fresh = require('fresh');
|
||||
var parseRange = require('range-parser');
|
||||
var parse = require('parseurl');
|
||||
var proxyaddr = require('proxy-addr');
|
||||
|
||||
/**
|
||||
* Request prototype.
|
||||
*/
|
||||
|
||||
var req = exports = module.exports = {
|
||||
__proto__: http.IncomingMessage.prototype
|
||||
};
|
||||
|
||||
/**
|
||||
* Return request header.
|
||||
*
|
||||
* The `Referrer` header field is special-cased,
|
||||
* both `Referrer` and `Referer` are interchangeable.
|
||||
*
|
||||
* Examples:
|
||||
*
|
||||
* req.get('Content-Type');
|
||||
* // => "text/plain"
|
||||
*
|
||||
* req.get('content-type');
|
||||
* // => "text/plain"
|
||||
*
|
||||
* req.get('Something');
|
||||
* // => undefined
|
||||
*
|
||||
* Aliased as `req.header()`.
|
||||
*
|
||||
* @param {String} name
|
||||
* @return {String}
|
||||
* @public
|
||||
*/
|
||||
|
||||
req.get =
|
||||
req.header = function header(name) {
|
||||
var lc = name.toLowerCase();
|
||||
|
||||
switch (lc) {
|
||||
case 'referer':
|
||||
case 'referrer':
|
||||
return this.headers.referrer
|
||||
|| this.headers.referer;
|
||||
default:
|
||||
return this.headers[lc];
|
||||
}
|
||||
};
|
||||
|
||||
/**
|
||||
* To do: update docs.
|
||||
*
|
||||
* Check if the given `type(s)` is acceptable, returning
|
||||
* the best match when true, otherwise `undefined`, in which
|
||||
* case you should respond with 406 "Not Acceptable".
|
||||
*
|
||||
* The `type` value may be a single MIME type string
|
||||
* such as "application/json", an extension name
|
||||
* such as "json", a comma-delimited list such as "json, html, text/plain",
|
||||
* an argument list such as `"json", "html", "text/plain"`,
|
||||
* or an array `["json", "html", "text/plain"]`. When a list
|
||||
* or array is given, the _best_ match, if any is returned.
|
||||
*
|
||||
* Examples:
|
||||
*
|
||||
* // Accept: text/html
|
||||
* req.accepts('html');
|
||||
* // => "html"
|
||||
*
|
||||
* // Accept: text/*, application/json
|
||||
* req.accepts('html');
|
||||
* // => "html"
|
||||
* req.accepts('text/html');
|
||||
* // => "text/html"
|
||||
* req.accepts('json, text');
|
||||
* // => "json"
|
||||
* req.accepts('application/json');
|
||||
* // => "application/json"
|
||||
*
|
||||
* // Accept: text/*, application/json
|
||||
* req.accepts('image/png');
|
||||
* req.accepts('png');
|
||||
* // => undefined
|
||||
*
|
||||
* // Accept: text/*;q=.5, application/json
|
||||
* req.accepts(['html', 'json']);
|
||||
* req.accepts('html', 'json');
|
||||
* req.accepts('html, json');
|
||||
* // => "json"
|
||||
*
|
||||
* @param {String|Array} type(s)
|
||||
* @return {String|Array|Boolean}
|
||||
* @public
|
||||
*/
|
||||
|
||||
req.accepts = function(){
|
||||
var accept = accepts(this);
|
||||
return accept.types.apply(accept, arguments);
|
||||
};
|
||||
|
||||
/**
|
||||
* Check if the given `encoding`s are accepted.
|
||||
*
|
||||
* @param {String} ...encoding
|
||||
* @return {String|Array}
|
||||
* @public
|
||||
*/
|
||||
|
||||
req.acceptsEncodings = function(){
|
||||
var accept = accepts(this);
|
||||
return accept.encodings.apply(accept, arguments);
|
||||
};
|
||||
|
||||
req.acceptsEncoding = deprecate.function(req.acceptsEncodings,
|
||||
'req.acceptsEncoding: Use acceptsEncodings instead');
|
||||
|
||||
/**
|
||||
* Check if the given `charset`s are acceptable,
|
||||
* otherwise you should respond with 406 "Not Acceptable".
|
||||
*
|
||||
* @param {String} ...charset
|
||||
* @return {String|Array}
|
||||
* @public
|
||||
*/
|
||||
|
||||
req.acceptsCharsets = function(){
|
||||
var accept = accepts(this);
|
||||
return accept.charsets.apply(accept, arguments);
|
||||
};
|
||||
|
||||
req.acceptsCharset = deprecate.function(req.acceptsCharsets,
|
||||
'req.acceptsCharset: Use acceptsCharsets instead');
|
||||
|
||||
/**
|
||||
* Check if the given `lang`s are acceptable,
|
||||
* otherwise you should respond with 406 "Not Acceptable".
|
||||
*
|
||||
* @param {String} ...lang
|
||||
* @return {String|Array}
|
||||
* @public
|
||||
*/
|
||||
|
||||
req.acceptsLanguages = function(){
|
||||
var accept = accepts(this);
|
||||
return accept.languages.apply(accept, arguments);
|
||||
};
|
||||
|
||||
req.acceptsLanguage = deprecate.function(req.acceptsLanguages,
|
||||
'req.acceptsLanguage: Use acceptsLanguages instead');
|
||||
|
||||
/**
|
||||
* Parse Range header field,
|
||||
* capping to the given `size`.
|
||||
*
|
||||
* Unspecified ranges such as "0-" require
|
||||
* knowledge of your resource length. In
|
||||
* the case of a byte range this is of course
|
||||
* the total number of bytes. If the Range
|
||||
* header field is not given `null` is returned,
|
||||
* `-1` when unsatisfiable, `-2` when syntactically invalid.
|
||||
*
|
||||
* NOTE: remember that ranges are inclusive, so
|
||||
* for example "Range: users=0-3" should respond
|
||||
* with 4 users when available, not 3.
|
||||
*
|
||||
* @param {Number} size
|
||||
* @return {Array}
|
||||
* @public
|
||||
*/
|
||||
|
||||
req.range = function(size){
|
||||
var range = this.get('Range');
|
||||
if (!range) return;
|
||||
return parseRange(size, range);
|
||||
};
|
||||
|
||||
/**
|
||||
* Return the value of param `name` when present or `defaultValue`.
|
||||
*
|
||||
* - Checks route placeholders, ex: _/user/:id_
|
||||
* - Checks body params, ex: id=12, {"id":12}
|
||||
* - Checks query string params, ex: ?id=12
|
||||
*
|
||||
* To utilize request bodies, `req.body`
|
||||
* should be an object. This can be done by using
|
||||
* the `bodyParser()` middleware.
|
||||
*
|
||||
* @param {String} name
|
||||
* @param {Mixed} [defaultValue]
|
||||
* @return {String}
|
||||
* @public
|
||||
*/
|
||||
|
||||
req.param = function param(name, defaultValue) {
|
||||
var params = this.params || {};
|
||||
var body = this.body || {};
|
||||
var query = this.query || {};
|
||||
|
||||
var args = arguments.length === 1
|
||||
? 'name'
|
||||
: 'name, default';
|
||||
deprecate('req.param(' + args + '): Use req.params, req.body, or req.query instead');
|
||||
|
||||
if (null != params[name] && params.hasOwnProperty(name)) return params[name];
|
||||
if (null != body[name]) return body[name];
|
||||
if (null != query[name]) return query[name];
|
||||
|
||||
return defaultValue;
|
||||
};
|
||||
|
||||
/**
|
||||
* Check if the incoming request contains the "Content-Type"
|
||||
* header field, and it contains the give mime `type`.
|
||||
*
|
||||
* Examples:
|
||||
*
|
||||
* // With Content-Type: text/html; charset=utf-8
|
||||
* req.is('html');
|
||||
* req.is('text/html');
|
||||
* req.is('text/*');
|
||||
* // => true
|
||||
*
|
||||
* // When Content-Type is application/json
|
||||
* req.is('json');
|
||||
* req.is('application/json');
|
||||
* req.is('application/*');
|
||||
* // => true
|
||||
*
|
||||
* req.is('html');
|
||||
* // => false
|
||||
*
|
||||
* @param {String|Array} types...
|
||||
* @return {String|false|null}
|
||||
* @public
|
||||
*/
|
||||
|
||||
req.is = function is(types) {
|
||||
var arr = types;
|
||||
|
||||
// support flattened arguments
|
||||
if (!Array.isArray(types)) {
|
||||
arr = new Array(arguments.length);
|
||||
for (var i = 0; i < arr.length; i++) {
|
||||
arr[i] = arguments[i];
|
||||
}
|
||||
}
|
||||
|
||||
return typeis(this, arr);
|
||||
};
|
||||
|
||||
/**
|
||||
* Return the protocol string "http" or "https"
|
||||
* when requested with TLS. When the "trust proxy"
|
||||
* setting trusts the socket address, the
|
||||
* "X-Forwarded-Proto" header field will be trusted
|
||||
* and used if present.
|
||||
*
|
||||
* If you're running behind a reverse proxy that
|
||||
* supplies https for you this may be enabled.
|
||||
*
|
||||
* @return {String}
|
||||
* @public
|
||||
*/
|
||||
|
||||
defineGetter(req, 'protocol', function protocol(){
|
||||
var proto = this.connection.encrypted
|
||||
? 'https'
|
||||
: 'http';
|
||||
var trust = this.app.get('trust proxy fn');
|
||||
|
||||
if (!trust(this.connection.remoteAddress, 0)) {
|
||||
return proto;
|
||||
}
|
||||
|
||||
// Note: X-Forwarded-Proto is normally only ever a
|
||||
// single value, but this is to be safe.
|
||||
proto = this.get('X-Forwarded-Proto') || proto;
|
||||
return proto.split(/\s*,\s*/)[0];
|
||||
});
|
||||
|
||||
/**
|
||||
* Short-hand for:
|
||||
*
|
||||
* req.protocol == 'https'
|
||||
*
|
||||
* @return {Boolean}
|
||||
* @public
|
||||
*/
|
||||
|
||||
defineGetter(req, 'secure', function secure(){
|
||||
return this.protocol === 'https';
|
||||
});
|
||||
|
||||
/**
|
||||
* Return the remote address from the trusted proxy.
|
||||
*
|
||||
* The is the remote address on the socket unless
|
||||
* "trust proxy" is set.
|
||||
*
|
||||
* @return {String}
|
||||
* @public
|
||||
*/
|
||||
|
||||
defineGetter(req, 'ip', function ip(){
|
||||
var trust = this.app.get('trust proxy fn');
|
||||
return proxyaddr(this, trust);
|
||||
});
|
||||
|
||||
/**
|
||||
* When "trust proxy" is set, trusted proxy addresses + client.
|
||||
*
|
||||
* For example if the value were "client, proxy1, proxy2"
|
||||
* you would receive the array `["client", "proxy1", "proxy2"]`
|
||||
* where "proxy2" is the furthest down-stream and "proxy1" and
|
||||
* "proxy2" were trusted.
|
||||
*
|
||||
* @return {Array}
|
||||
* @public
|
||||
*/
|
||||
|
||||
defineGetter(req, 'ips', function ips() {
|
||||
var trust = this.app.get('trust proxy fn');
|
||||
var addrs = proxyaddr.all(this, trust);
|
||||
return addrs.slice(1).reverse();
|
||||
});
|
||||
|
||||
/**
|
||||
* Return subdomains as an array.
|
||||
*
|
||||
* Subdomains are the dot-separated parts of the host before the main domain of
|
||||
* the app. By default, the domain of the app is assumed to be the last two
|
||||
* parts of the host. This can be changed by setting "subdomain offset".
|
||||
*
|
||||
* For example, if the domain is "tobi.ferrets.example.com":
|
||||
* If "subdomain offset" is not set, req.subdomains is `["ferrets", "tobi"]`.
|
||||
* If "subdomain offset" is 3, req.subdomains is `["tobi"]`.
|
||||
*
|
||||
* @return {Array}
|
||||
* @public
|
||||
*/
|
||||
|
||||
defineGetter(req, 'subdomains', function subdomains() {
|
||||
var hostname = this.hostname;
|
||||
|
||||
if (!hostname) return [];
|
||||
|
||||
var offset = this.app.get('subdomain offset');
|
||||
var subdomains = !isIP(hostname)
|
||||
? hostname.split('.').reverse()
|
||||
: [hostname];
|
||||
|
||||
return subdomains.slice(offset);
|
||||
});
|
||||
|
||||
/**
|
||||
* Short-hand for `url.parse(req.url).pathname`.
|
||||
*
|
||||
* @return {String}
|
||||
* @public
|
||||
*/
|
||||
|
||||
defineGetter(req, 'path', function path() {
|
||||
return parse(this).pathname;
|
||||
});
|
||||
|
||||
/**
|
||||
* Parse the "Host" header field to a hostname.
|
||||
*
|
||||
* When the "trust proxy" setting trusts the socket
|
||||
* address, the "X-Forwarded-Host" header field will
|
||||
* be trusted.
|
||||
*
|
||||
* @return {String}
|
||||
* @public
|
||||
*/
|
||||
|
||||
defineGetter(req, 'hostname', function hostname(){
|
||||
var trust = this.app.get('trust proxy fn');
|
||||
var host = this.get('X-Forwarded-Host');
|
||||
|
||||
if (!host || !trust(this.connection.remoteAddress, 0)) {
|
||||
host = this.get('Host');
|
||||
}
|
||||
|
||||
if (!host) return;
|
||||
|
||||
// IPv6 literal support
|
||||
var offset = host[0] === '['
|
||||
? host.indexOf(']') + 1
|
||||
: 0;
|
||||
var index = host.indexOf(':', offset);
|
||||
|
||||
return index !== -1
|
||||
? host.substring(0, index)
|
||||
: host;
|
||||
});
|
||||
|
||||
// TODO: change req.host to return host in next major
|
||||
|
||||
defineGetter(req, 'host', deprecate.function(function host(){
|
||||
return this.hostname;
|
||||
}, 'req.host: Use req.hostname instead'));
|
||||
|
||||
/**
|
||||
* Check if the request is fresh, aka
|
||||
* Last-Modified and/or the ETag
|
||||
* still match.
|
||||
*
|
||||
* @return {Boolean}
|
||||
* @public
|
||||
*/
|
||||
|
||||
defineGetter(req, 'fresh', function(){
|
||||
var method = this.method;
|
||||
var s = this.res.statusCode;
|
||||
|
||||
// GET or HEAD for weak freshness validation only
|
||||
if ('GET' != method && 'HEAD' != method) return false;
|
||||
|
||||
// 2xx or 304 as per rfc2616 14.26
|
||||
if ((s >= 200 && s < 300) || 304 == s) {
|
||||
return fresh(this.headers, (this.res._headers || {}));
|
||||
}
|
||||
|
||||
return false;
|
||||
});
|
||||
|
||||
/**
|
||||
* Check if the request is stale, aka
|
||||
* "Last-Modified" and / or the "ETag" for the
|
||||
* resource has changed.
|
||||
*
|
||||
* @return {Boolean}
|
||||
* @public
|
||||
*/
|
||||
|
||||
defineGetter(req, 'stale', function stale(){
|
||||
return !this.fresh;
|
||||
});
|
||||
|
||||
/**
|
||||
* Check if the request was an _XMLHttpRequest_.
|
||||
*
|
||||
* @return {Boolean}
|
||||
* @public
|
||||
*/
|
||||
|
||||
defineGetter(req, 'xhr', function xhr(){
|
||||
var val = this.get('X-Requested-With') || '';
|
||||
return val.toLowerCase() === 'xmlhttprequest';
|
||||
});
|
||||
|
||||
/**
|
||||
* Helper function for creating a getter on an object.
|
||||
*
|
||||
* @param {Object} obj
|
||||
* @param {String} name
|
||||
* @param {Function} getter
|
||||
* @private
|
||||
*/
|
||||
function defineGetter(obj, name, getter) {
|
||||
Object.defineProperty(obj, name, {
|
||||
configurable: true,
|
||||
enumerable: true,
|
||||
get: getter
|
||||
});
|
||||
};
|
1053
node_modules/express/lib/response.js
generated
vendored
Normal file
1053
node_modules/express/lib/response.js
generated
vendored
Normal file
File diff suppressed because it is too large
Load Diff
645
node_modules/express/lib/router/index.js
generated
vendored
Normal file
645
node_modules/express/lib/router/index.js
generated
vendored
Normal file
@ -0,0 +1,645 @@
|
||||
/*!
|
||||
* express
|
||||
* Copyright(c) 2009-2013 TJ Holowaychuk
|
||||
* Copyright(c) 2013 Roman Shtylman
|
||||
* Copyright(c) 2014-2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict';
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var Route = require('./route');
|
||||
var Layer = require('./layer');
|
||||
var methods = require('methods');
|
||||
var mixin = require('utils-merge');
|
||||
var debug = require('debug')('express:router');
|
||||
var deprecate = require('depd')('express');
|
||||
var flatten = require('array-flatten');
|
||||
var parseUrl = require('parseurl');
|
||||
|
||||
/**
|
||||
* Module variables.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var objectRegExp = /^\[object (\S+)\]$/;
|
||||
var slice = Array.prototype.slice;
|
||||
var toString = Object.prototype.toString;
|
||||
|
||||
/**
|
||||
* Initialize a new `Router` with the given `options`.
|
||||
*
|
||||
* @param {Object} options
|
||||
* @return {Router} which is an callable function
|
||||
* @public
|
||||
*/
|
||||
|
||||
var proto = module.exports = function(options) {
|
||||
var opts = options || {};
|
||||
|
||||
function router(req, res, next) {
|
||||
router.handle(req, res, next);
|
||||
}
|
||||
|
||||
// mixin Router class functions
|
||||
router.__proto__ = proto;
|
||||
|
||||
router.params = {};
|
||||
router._params = [];
|
||||
router.caseSensitive = opts.caseSensitive;
|
||||
router.mergeParams = opts.mergeParams;
|
||||
router.strict = opts.strict;
|
||||
router.stack = [];
|
||||
|
||||
return router;
|
||||
};
|
||||
|
||||
/**
|
||||
* Map the given param placeholder `name`(s) to the given callback.
|
||||
*
|
||||
* Parameter mapping is used to provide pre-conditions to routes
|
||||
* which use normalized placeholders. For example a _:user_id_ parameter
|
||||
* could automatically load a user's information from the database without
|
||||
* any additional code,
|
||||
*
|
||||
* The callback uses the same signature as middleware, the only difference
|
||||
* being that the value of the placeholder is passed, in this case the _id_
|
||||
* of the user. Once the `next()` function is invoked, just like middleware
|
||||
* it will continue on to execute the route, or subsequent parameter functions.
|
||||
*
|
||||
* Just like in middleware, you must either respond to the request or call next
|
||||
* to avoid stalling the request.
|
||||
*
|
||||
* app.param('user_id', function(req, res, next, id){
|
||||
* User.find(id, function(err, user){
|
||||
* if (err) {
|
||||
* return next(err);
|
||||
* } else if (!user) {
|
||||
* return next(new Error('failed to load user'));
|
||||
* }
|
||||
* req.user = user;
|
||||
* next();
|
||||
* });
|
||||
* });
|
||||
*
|
||||
* @param {String} name
|
||||
* @param {Function} fn
|
||||
* @return {app} for chaining
|
||||
* @public
|
||||
*/
|
||||
|
||||
proto.param = function param(name, fn) {
|
||||
// param logic
|
||||
if (typeof name === 'function') {
|
||||
deprecate('router.param(fn): Refactor to use path params');
|
||||
this._params.push(name);
|
||||
return;
|
||||
}
|
||||
|
||||
// apply param functions
|
||||
var params = this._params;
|
||||
var len = params.length;
|
||||
var ret;
|
||||
|
||||
if (name[0] === ':') {
|
||||
deprecate('router.param(' + JSON.stringify(name) + ', fn): Use router.param(' + JSON.stringify(name.substr(1)) + ', fn) instead');
|
||||
name = name.substr(1);
|
||||
}
|
||||
|
||||
for (var i = 0; i < len; ++i) {
|
||||
if (ret = params[i](name, fn)) {
|
||||
fn = ret;
|
||||
}
|
||||
}
|
||||
|
||||
// ensure we end up with a
|
||||
// middleware function
|
||||
if ('function' != typeof fn) {
|
||||
throw new Error('invalid param() call for ' + name + ', got ' + fn);
|
||||
}
|
||||
|
||||
(this.params[name] = this.params[name] || []).push(fn);
|
||||
return this;
|
||||
};
|
||||
|
||||
/**
|
||||
* Dispatch a req, res into the router.
|
||||
* @private
|
||||
*/
|
||||
|
||||
proto.handle = function handle(req, res, out) {
|
||||
var self = this;
|
||||
|
||||
debug('dispatching %s %s', req.method, req.url);
|
||||
|
||||
var search = 1 + req.url.indexOf('?');
|
||||
var pathlength = search ? search - 1 : req.url.length;
|
||||
var fqdn = req.url[0] !== '/' && 1 + req.url.substr(0, pathlength).indexOf('://');
|
||||
var protohost = fqdn ? req.url.substr(0, req.url.indexOf('/', 2 + fqdn)) : '';
|
||||
var idx = 0;
|
||||
var removed = '';
|
||||
var slashAdded = false;
|
||||
var paramcalled = {};
|
||||
|
||||
// store options for OPTIONS request
|
||||
// only used if OPTIONS request
|
||||
var options = [];
|
||||
|
||||
// middleware and routes
|
||||
var stack = self.stack;
|
||||
|
||||
// manage inter-router variables
|
||||
var parentParams = req.params;
|
||||
var parentUrl = req.baseUrl || '';
|
||||
var done = restore(out, req, 'baseUrl', 'next', 'params');
|
||||
|
||||
// setup next layer
|
||||
req.next = next;
|
||||
|
||||
// for options requests, respond with a default if nothing else responds
|
||||
if (req.method === 'OPTIONS') {
|
||||
done = wrap(done, function(old, err) {
|
||||
if (err || options.length === 0) return old(err);
|
||||
sendOptionsResponse(res, options, old);
|
||||
});
|
||||
}
|
||||
|
||||
// setup basic req values
|
||||
req.baseUrl = parentUrl;
|
||||
req.originalUrl = req.originalUrl || req.url;
|
||||
|
||||
next();
|
||||
|
||||
function next(err) {
|
||||
var layerError = err === 'route'
|
||||
? null
|
||||
: err;
|
||||
|
||||
// remove added slash
|
||||
if (slashAdded) {
|
||||
req.url = req.url.substr(1);
|
||||
slashAdded = false;
|
||||
}
|
||||
|
||||
// restore altered req.url
|
||||
if (removed.length !== 0) {
|
||||
req.baseUrl = parentUrl;
|
||||
req.url = protohost + removed + req.url.substr(protohost.length);
|
||||
removed = '';
|
||||
}
|
||||
|
||||
// no more matching layers
|
||||
if (idx >= stack.length) {
|
||||
setImmediate(done, layerError);
|
||||
return;
|
||||
}
|
||||
|
||||
// get pathname of request
|
||||
var path = getPathname(req);
|
||||
|
||||
if (path == null) {
|
||||
return done(layerError);
|
||||
}
|
||||
|
||||
// find next matching layer
|
||||
var layer;
|
||||
var match;
|
||||
var route;
|
||||
|
||||
while (match !== true && idx < stack.length) {
|
||||
layer = stack[idx++];
|
||||
match = matchLayer(layer, path);
|
||||
route = layer.route;
|
||||
|
||||
if (typeof match !== 'boolean') {
|
||||
// hold on to layerError
|
||||
layerError = layerError || match;
|
||||
}
|
||||
|
||||
if (match !== true) {
|
||||
continue;
|
||||
}
|
||||
|
||||
if (!route) {
|
||||
// process non-route handlers normally
|
||||
continue;
|
||||
}
|
||||
|
||||
if (layerError) {
|
||||
// routes do not match with a pending error
|
||||
match = false;
|
||||
continue;
|
||||
}
|
||||
|
||||
var method = req.method;
|
||||
var has_method = route._handles_method(method);
|
||||
|
||||
// build up automatic options response
|
||||
if (!has_method && method === 'OPTIONS') {
|
||||
appendMethods(options, route._options());
|
||||
}
|
||||
|
||||
// don't even bother matching route
|
||||
if (!has_method && method !== 'HEAD') {
|
||||
match = false;
|
||||
continue;
|
||||
}
|
||||
}
|
||||
|
||||
// no match
|
||||
if (match !== true) {
|
||||
return done(layerError);
|
||||
}
|
||||
|
||||
// store route for dispatch on change
|
||||
if (route) {
|
||||
req.route = route;
|
||||
}
|
||||
|
||||
// Capture one-time layer values
|
||||
req.params = self.mergeParams
|
||||
? mergeParams(layer.params, parentParams)
|
||||
: layer.params;
|
||||
var layerPath = layer.path;
|
||||
|
||||
// this should be done for the layer
|
||||
self.process_params(layer, paramcalled, req, res, function (err) {
|
||||
if (err) {
|
||||
return next(layerError || err);
|
||||
}
|
||||
|
||||
if (route) {
|
||||
return layer.handle_request(req, res, next);
|
||||
}
|
||||
|
||||
trim_prefix(layer, layerError, layerPath, path);
|
||||
});
|
||||
}
|
||||
|
||||
function trim_prefix(layer, layerError, layerPath, path) {
|
||||
var c = path[layerPath.length];
|
||||
if (c && '/' !== c && '.' !== c) return next(layerError);
|
||||
|
||||
// Trim off the part of the url that matches the route
|
||||
// middleware (.use stuff) needs to have the path stripped
|
||||
if (layerPath.length !== 0) {
|
||||
debug('trim prefix (%s) from url %s', layerPath, req.url);
|
||||
removed = layerPath;
|
||||
req.url = protohost + req.url.substr(protohost.length + removed.length);
|
||||
|
||||
// Ensure leading slash
|
||||
if (!fqdn && req.url[0] !== '/') {
|
||||
req.url = '/' + req.url;
|
||||
slashAdded = true;
|
||||
}
|
||||
|
||||
// Setup base URL (no trailing slash)
|
||||
req.baseUrl = parentUrl + (removed[removed.length - 1] === '/'
|
||||
? removed.substring(0, removed.length - 1)
|
||||
: removed);
|
||||
}
|
||||
|
||||
debug('%s %s : %s', layer.name, layerPath, req.originalUrl);
|
||||
|
||||
if (layerError) {
|
||||
layer.handle_error(layerError, req, res, next);
|
||||
} else {
|
||||
layer.handle_request(req, res, next);
|
||||
}
|
||||
}
|
||||
};
|
||||
|
||||
/**
|
||||
* Process any parameters for the layer.
|
||||
* @private
|
||||
*/
|
||||
|
||||
proto.process_params = function process_params(layer, called, req, res, done) {
|
||||
var params = this.params;
|
||||
|
||||
// captured parameters from the layer, keys and values
|
||||
var keys = layer.keys;
|
||||
|
||||
// fast track
|
||||
if (!keys || keys.length === 0) {
|
||||
return done();
|
||||
}
|
||||
|
||||
var i = 0;
|
||||
var name;
|
||||
var paramIndex = 0;
|
||||
var key;
|
||||
var paramVal;
|
||||
var paramCallbacks;
|
||||
var paramCalled;
|
||||
|
||||
// process params in order
|
||||
// param callbacks can be async
|
||||
function param(err) {
|
||||
if (err) {
|
||||
return done(err);
|
||||
}
|
||||
|
||||
if (i >= keys.length ) {
|
||||
return done();
|
||||
}
|
||||
|
||||
paramIndex = 0;
|
||||
key = keys[i++];
|
||||
|
||||
if (!key) {
|
||||
return done();
|
||||
}
|
||||
|
||||
name = key.name;
|
||||
paramVal = req.params[name];
|
||||
paramCallbacks = params[name];
|
||||
paramCalled = called[name];
|
||||
|
||||
if (paramVal === undefined || !paramCallbacks) {
|
||||
return param();
|
||||
}
|
||||
|
||||
// param previously called with same value or error occurred
|
||||
if (paramCalled && (paramCalled.match === paramVal
|
||||
|| (paramCalled.error && paramCalled.error !== 'route'))) {
|
||||
// restore value
|
||||
req.params[name] = paramCalled.value;
|
||||
|
||||
// next param
|
||||
return param(paramCalled.error);
|
||||
}
|
||||
|
||||
called[name] = paramCalled = {
|
||||
error: null,
|
||||
match: paramVal,
|
||||
value: paramVal
|
||||
};
|
||||
|
||||
paramCallback();
|
||||
}
|
||||
|
||||
// single param callbacks
|
||||
function paramCallback(err) {
|
||||
var fn = paramCallbacks[paramIndex++];
|
||||
|
||||
// store updated value
|
||||
paramCalled.value = req.params[key.name];
|
||||
|
||||
if (err) {
|
||||
// store error
|
||||
paramCalled.error = err;
|
||||
param(err);
|
||||
return;
|
||||
}
|
||||
|
||||
if (!fn) return param();
|
||||
|
||||
try {
|
||||
fn(req, res, paramCallback, paramVal, key.name);
|
||||
} catch (e) {
|
||||
paramCallback(e);
|
||||
}
|
||||
}
|
||||
|
||||
param();
|
||||
};
|
||||
|
||||
/**
|
||||
* Use the given middleware function, with optional path, defaulting to "/".
|
||||
*
|
||||
* Use (like `.all`) will run for any http METHOD, but it will not add
|
||||
* handlers for those methods so OPTIONS requests will not consider `.use`
|
||||
* functions even if they could respond.
|
||||
*
|
||||
* The other difference is that _route_ path is stripped and not visible
|
||||
* to the handler function. The main effect of this feature is that mounted
|
||||
* handlers can operate without any code changes regardless of the "prefix"
|
||||
* pathname.
|
||||
*
|
||||
* @public
|
||||
*/
|
||||
|
||||
proto.use = function use(fn) {
|
||||
var offset = 0;
|
||||
var path = '/';
|
||||
|
||||
// default path to '/'
|
||||
// disambiguate router.use([fn])
|
||||
if (typeof fn !== 'function') {
|
||||
var arg = fn;
|
||||
|
||||
while (Array.isArray(arg) && arg.length !== 0) {
|
||||
arg = arg[0];
|
||||
}
|
||||
|
||||
// first arg is the path
|
||||
if (typeof arg !== 'function') {
|
||||
offset = 1;
|
||||
path = fn;
|
||||
}
|
||||
}
|
||||
|
||||
var callbacks = flatten(slice.call(arguments, offset));
|
||||
|
||||
if (callbacks.length === 0) {
|
||||
throw new TypeError('Router.use() requires middleware functions');
|
||||
}
|
||||
|
||||
for (var i = 0; i < callbacks.length; i++) {
|
||||
var fn = callbacks[i];
|
||||
|
||||
if (typeof fn !== 'function') {
|
||||
throw new TypeError('Router.use() requires middleware function but got a ' + gettype(fn));
|
||||
}
|
||||
|
||||
// add the middleware
|
||||
debug('use %s %s', path, fn.name || '<anonymous>');
|
||||
|
||||
var layer = new Layer(path, {
|
||||
sensitive: this.caseSensitive,
|
||||
strict: false,
|
||||
end: false
|
||||
}, fn);
|
||||
|
||||
layer.route = undefined;
|
||||
|
||||
this.stack.push(layer);
|
||||
}
|
||||
|
||||
return this;
|
||||
};
|
||||
|
||||
/**
|
||||
* Create a new Route for the given path.
|
||||
*
|
||||
* Each route contains a separate middleware stack and VERB handlers.
|
||||
*
|
||||
* See the Route api documentation for details on adding handlers
|
||||
* and middleware to routes.
|
||||
*
|
||||
* @param {String} path
|
||||
* @return {Route}
|
||||
* @public
|
||||
*/
|
||||
|
||||
proto.route = function route(path) {
|
||||
var route = new Route(path);
|
||||
|
||||
var layer = new Layer(path, {
|
||||
sensitive: this.caseSensitive,
|
||||
strict: this.strict,
|
||||
end: true
|
||||
}, route.dispatch.bind(route));
|
||||
|
||||
layer.route = route;
|
||||
|
||||
this.stack.push(layer);
|
||||
return route;
|
||||
};
|
||||
|
||||
// create Router#VERB functions
|
||||
methods.concat('all').forEach(function(method){
|
||||
proto[method] = function(path){
|
||||
var route = this.route(path)
|
||||
route[method].apply(route, slice.call(arguments, 1));
|
||||
return this;
|
||||
};
|
||||
});
|
||||
|
||||
// append methods to a list of methods
|
||||
function appendMethods(list, addition) {
|
||||
for (var i = 0; i < addition.length; i++) {
|
||||
var method = addition[i];
|
||||
if (list.indexOf(method) === -1) {
|
||||
list.push(method);
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
// get pathname of request
|
||||
function getPathname(req) {
|
||||
try {
|
||||
return parseUrl(req).pathname;
|
||||
} catch (err) {
|
||||
return undefined;
|
||||
}
|
||||
}
|
||||
|
||||
// get type for error message
|
||||
function gettype(obj) {
|
||||
var type = typeof obj;
|
||||
|
||||
if (type !== 'object') {
|
||||
return type;
|
||||
}
|
||||
|
||||
// inspect [[Class]] for objects
|
||||
return toString.call(obj)
|
||||
.replace(objectRegExp, '$1');
|
||||
}
|
||||
|
||||
/**
|
||||
* Match path to a layer.
|
||||
*
|
||||
* @param {Layer} layer
|
||||
* @param {string} path
|
||||
* @private
|
||||
*/
|
||||
|
||||
function matchLayer(layer, path) {
|
||||
try {
|
||||
return layer.match(path);
|
||||
} catch (err) {
|
||||
return err;
|
||||
}
|
||||
}
|
||||
|
||||
// merge params with parent params
|
||||
function mergeParams(params, parent) {
|
||||
if (typeof parent !== 'object' || !parent) {
|
||||
return params;
|
||||
}
|
||||
|
||||
// make copy of parent for base
|
||||
var obj = mixin({}, parent);
|
||||
|
||||
// simple non-numeric merging
|
||||
if (!(0 in params) || !(0 in parent)) {
|
||||
return mixin(obj, params);
|
||||
}
|
||||
|
||||
var i = 0;
|
||||
var o = 0;
|
||||
|
||||
// determine numeric gaps
|
||||
while (i in params) {
|
||||
i++;
|
||||
}
|
||||
|
||||
while (o in parent) {
|
||||
o++;
|
||||
}
|
||||
|
||||
// offset numeric indices in params before merge
|
||||
for (i--; i >= 0; i--) {
|
||||
params[i + o] = params[i];
|
||||
|
||||
// create holes for the merge when necessary
|
||||
if (i < o) {
|
||||
delete params[i];
|
||||
}
|
||||
}
|
||||
|
||||
return mixin(obj, params);
|
||||
}
|
||||
|
||||
// restore obj props after function
|
||||
function restore(fn, obj) {
|
||||
var props = new Array(arguments.length - 2);
|
||||
var vals = new Array(arguments.length - 2);
|
||||
|
||||
for (var i = 0; i < props.length; i++) {
|
||||
props[i] = arguments[i + 2];
|
||||
vals[i] = obj[props[i]];
|
||||
}
|
||||
|
||||
return function(err){
|
||||
// restore vals
|
||||
for (var i = 0; i < props.length; i++) {
|
||||
obj[props[i]] = vals[i];
|
||||
}
|
||||
|
||||
return fn.apply(this, arguments);
|
||||
};
|
||||
}
|
||||
|
||||
// send an OPTIONS response
|
||||
function sendOptionsResponse(res, options, next) {
|
||||
try {
|
||||
var body = options.join(',');
|
||||
res.set('Allow', body);
|
||||
res.send(body);
|
||||
} catch (err) {
|
||||
next(err);
|
||||
}
|
||||
}
|
||||
|
||||
// wrap a function
|
||||
function wrap(old, fn) {
|
||||
return function proxy() {
|
||||
var args = new Array(arguments.length + 1);
|
||||
|
||||
args[0] = old;
|
||||
for (var i = 0, len = arguments.length; i < len; i++) {
|
||||
args[i + 1] = arguments[i];
|
||||
}
|
||||
|
||||
fn.apply(this, args);
|
||||
};
|
||||
}
|
176
node_modules/express/lib/router/layer.js
generated
vendored
Normal file
176
node_modules/express/lib/router/layer.js
generated
vendored
Normal file
@ -0,0 +1,176 @@
|
||||
/*!
|
||||
* express
|
||||
* Copyright(c) 2009-2013 TJ Holowaychuk
|
||||
* Copyright(c) 2013 Roman Shtylman
|
||||
* Copyright(c) 2014-2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict';
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var pathRegexp = require('path-to-regexp');
|
||||
var debug = require('debug')('express:router:layer');
|
||||
|
||||
/**
|
||||
* Module variables.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var hasOwnProperty = Object.prototype.hasOwnProperty;
|
||||
|
||||
/**
|
||||
* Module exports.
|
||||
* @public
|
||||
*/
|
||||
|
||||
module.exports = Layer;
|
||||
|
||||
function Layer(path, options, fn) {
|
||||
if (!(this instanceof Layer)) {
|
||||
return new Layer(path, options, fn);
|
||||
}
|
||||
|
||||
debug('new %s', path);
|
||||
var opts = options || {};
|
||||
|
||||
this.handle = fn;
|
||||
this.name = fn.name || '<anonymous>';
|
||||
this.params = undefined;
|
||||
this.path = undefined;
|
||||
this.regexp = pathRegexp(path, this.keys = [], opts);
|
||||
|
||||
if (path === '/' && opts.end === false) {
|
||||
this.regexp.fast_slash = true;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Handle the error for the layer.
|
||||
*
|
||||
* @param {Error} error
|
||||
* @param {Request} req
|
||||
* @param {Response} res
|
||||
* @param {function} next
|
||||
* @api private
|
||||
*/
|
||||
|
||||
Layer.prototype.handle_error = function handle_error(error, req, res, next) {
|
||||
var fn = this.handle;
|
||||
|
||||
if (fn.length !== 4) {
|
||||
// not a standard error handler
|
||||
return next(error);
|
||||
}
|
||||
|
||||
try {
|
||||
fn(error, req, res, next);
|
||||
} catch (err) {
|
||||
next(err);
|
||||
}
|
||||
};
|
||||
|
||||
/**
|
||||
* Handle the request for the layer.
|
||||
*
|
||||
* @param {Request} req
|
||||
* @param {Response} res
|
||||
* @param {function} next
|
||||
* @api private
|
||||
*/
|
||||
|
||||
Layer.prototype.handle_request = function handle(req, res, next) {
|
||||
var fn = this.handle;
|
||||
|
||||
if (fn.length > 3) {
|
||||
// not a standard request handler
|
||||
return next();
|
||||
}
|
||||
|
||||
try {
|
||||
fn(req, res, next);
|
||||
} catch (err) {
|
||||
next(err);
|
||||
}
|
||||
};
|
||||
|
||||
/**
|
||||
* Check if this route matches `path`, if so
|
||||
* populate `.params`.
|
||||
*
|
||||
* @param {String} path
|
||||
* @return {Boolean}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
Layer.prototype.match = function match(path) {
|
||||
if (path == null) {
|
||||
// no path, nothing matches
|
||||
this.params = undefined;
|
||||
this.path = undefined;
|
||||
return false;
|
||||
}
|
||||
|
||||
if (this.regexp.fast_slash) {
|
||||
// fast path non-ending match for / (everything matches)
|
||||
this.params = {};
|
||||
this.path = '';
|
||||
return true;
|
||||
}
|
||||
|
||||
var m = this.regexp.exec(path);
|
||||
|
||||
if (!m) {
|
||||
this.params = undefined;
|
||||
this.path = undefined;
|
||||
return false;
|
||||
}
|
||||
|
||||
// store values
|
||||
this.params = {};
|
||||
this.path = m[0];
|
||||
|
||||
var keys = this.keys;
|
||||
var params = this.params;
|
||||
|
||||
for (var i = 1; i < m.length; i++) {
|
||||
var key = keys[i - 1];
|
||||
var prop = key.name;
|
||||
var val = decode_param(m[i]);
|
||||
|
||||
if (val !== undefined || !(hasOwnProperty.call(params, prop))) {
|
||||
params[prop] = val;
|
||||
}
|
||||
}
|
||||
|
||||
return true;
|
||||
};
|
||||
|
||||
/**
|
||||
* Decode param value.
|
||||
*
|
||||
* @param {string} val
|
||||
* @return {string}
|
||||
* @private
|
||||
*/
|
||||
|
||||
function decode_param(val) {
|
||||
if (typeof val !== 'string' || val.length === 0) {
|
||||
return val;
|
||||
}
|
||||
|
||||
try {
|
||||
return decodeURIComponent(val);
|
||||
} catch (err) {
|
||||
if (err instanceof URIError) {
|
||||
err.message = 'Failed to decode param \'' + val + '\'';
|
||||
err.status = err.statusCode = 400;
|
||||
}
|
||||
|
||||
throw err;
|
||||
}
|
||||
}
|
210
node_modules/express/lib/router/route.js
generated
vendored
Normal file
210
node_modules/express/lib/router/route.js
generated
vendored
Normal file
@ -0,0 +1,210 @@
|
||||
/*!
|
||||
* express
|
||||
* Copyright(c) 2009-2013 TJ Holowaychuk
|
||||
* Copyright(c) 2013 Roman Shtylman
|
||||
* Copyright(c) 2014-2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict';
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var debug = require('debug')('express:router:route');
|
||||
var flatten = require('array-flatten');
|
||||
var Layer = require('./layer');
|
||||
var methods = require('methods');
|
||||
|
||||
/**
|
||||
* Module variables.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var slice = Array.prototype.slice;
|
||||
var toString = Object.prototype.toString;
|
||||
|
||||
/**
|
||||
* Module exports.
|
||||
* @public
|
||||
*/
|
||||
|
||||
module.exports = Route;
|
||||
|
||||
/**
|
||||
* Initialize `Route` with the given `path`,
|
||||
*
|
||||
* @param {String} path
|
||||
* @public
|
||||
*/
|
||||
|
||||
function Route(path) {
|
||||
this.path = path;
|
||||
this.stack = [];
|
||||
|
||||
debug('new %s', path);
|
||||
|
||||
// route handlers for various http methods
|
||||
this.methods = {};
|
||||
}
|
||||
|
||||
/**
|
||||
* Determine if the route handles a given method.
|
||||
* @private
|
||||
*/
|
||||
|
||||
Route.prototype._handles_method = function _handles_method(method) {
|
||||
if (this.methods._all) {
|
||||
return true;
|
||||
}
|
||||
|
||||
var name = method.toLowerCase();
|
||||
|
||||
if (name === 'head' && !this.methods['head']) {
|
||||
name = 'get';
|
||||
}
|
||||
|
||||
return Boolean(this.methods[name]);
|
||||
};
|
||||
|
||||
/**
|
||||
* @return {Array} supported HTTP methods
|
||||
* @private
|
||||
*/
|
||||
|
||||
Route.prototype._options = function _options() {
|
||||
var methods = Object.keys(this.methods);
|
||||
|
||||
// append automatic head
|
||||
if (this.methods.get && !this.methods.head) {
|
||||
methods.push('head');
|
||||
}
|
||||
|
||||
for (var i = 0; i < methods.length; i++) {
|
||||
// make upper case
|
||||
methods[i] = methods[i].toUpperCase();
|
||||
}
|
||||
|
||||
return methods;
|
||||
};
|
||||
|
||||
/**
|
||||
* dispatch req, res into this route
|
||||
* @private
|
||||
*/
|
||||
|
||||
Route.prototype.dispatch = function dispatch(req, res, done) {
|
||||
var idx = 0;
|
||||
var stack = this.stack;
|
||||
if (stack.length === 0) {
|
||||
return done();
|
||||
}
|
||||
|
||||
var method = req.method.toLowerCase();
|
||||
if (method === 'head' && !this.methods['head']) {
|
||||
method = 'get';
|
||||
}
|
||||
|
||||
req.route = this;
|
||||
|
||||
next();
|
||||
|
||||
function next(err) {
|
||||
if (err && err === 'route') {
|
||||
return done();
|
||||
}
|
||||
|
||||
var layer = stack[idx++];
|
||||
if (!layer) {
|
||||
return done(err);
|
||||
}
|
||||
|
||||
if (layer.method && layer.method !== method) {
|
||||
return next(err);
|
||||
}
|
||||
|
||||
if (err) {
|
||||
layer.handle_error(err, req, res, next);
|
||||
} else {
|
||||
layer.handle_request(req, res, next);
|
||||
}
|
||||
}
|
||||
};
|
||||
|
||||
/**
|
||||
* Add a handler for all HTTP verbs to this route.
|
||||
*
|
||||
* Behaves just like middleware and can respond or call `next`
|
||||
* to continue processing.
|
||||
*
|
||||
* You can use multiple `.all` call to add multiple handlers.
|
||||
*
|
||||
* function check_something(req, res, next){
|
||||
* next();
|
||||
* };
|
||||
*
|
||||
* function validate_user(req, res, next){
|
||||
* next();
|
||||
* };
|
||||
*
|
||||
* route
|
||||
* .all(validate_user)
|
||||
* .all(check_something)
|
||||
* .get(function(req, res, next){
|
||||
* res.send('hello world');
|
||||
* });
|
||||
*
|
||||
* @param {function} handler
|
||||
* @return {Route} for chaining
|
||||
* @api public
|
||||
*/
|
||||
|
||||
Route.prototype.all = function all() {
|
||||
var handles = flatten(slice.call(arguments));
|
||||
|
||||
for (var i = 0; i < handles.length; i++) {
|
||||
var handle = handles[i];
|
||||
|
||||
if (typeof handle !== 'function') {
|
||||
var type = toString.call(handle);
|
||||
var msg = 'Route.all() requires callback functions but got a ' + type;
|
||||
throw new TypeError(msg);
|
||||
}
|
||||
|
||||
var layer = Layer('/', {}, handle);
|
||||
layer.method = undefined;
|
||||
|
||||
this.methods._all = true;
|
||||
this.stack.push(layer);
|
||||
}
|
||||
|
||||
return this;
|
||||
};
|
||||
|
||||
methods.forEach(function(method){
|
||||
Route.prototype[method] = function(){
|
||||
var handles = flatten(slice.call(arguments));
|
||||
|
||||
for (var i = 0; i < handles.length; i++) {
|
||||
var handle = handles[i];
|
||||
|
||||
if (typeof handle !== 'function') {
|
||||
var type = toString.call(handle);
|
||||
var msg = 'Route.' + method + '() requires callback functions but got a ' + type;
|
||||
throw new Error(msg);
|
||||
}
|
||||
|
||||
debug('%s %s', method, this.path);
|
||||
|
||||
var layer = Layer('/', {}, handle);
|
||||
layer.method = method;
|
||||
|
||||
this.methods[method] = true;
|
||||
this.stack.push(layer);
|
||||
}
|
||||
|
||||
return this;
|
||||
};
|
||||
});
|
300
node_modules/express/lib/utils.js
generated
vendored
Normal file
300
node_modules/express/lib/utils.js
generated
vendored
Normal file
@ -0,0 +1,300 @@
|
||||
/*!
|
||||
* express
|
||||
* Copyright(c) 2009-2013 TJ Holowaychuk
|
||||
* Copyright(c) 2014-2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict';
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
* @api private
|
||||
*/
|
||||
|
||||
var contentDisposition = require('content-disposition');
|
||||
var contentType = require('content-type');
|
||||
var deprecate = require('depd')('express');
|
||||
var flatten = require('array-flatten');
|
||||
var mime = require('send').mime;
|
||||
var basename = require('path').basename;
|
||||
var etag = require('etag');
|
||||
var proxyaddr = require('proxy-addr');
|
||||
var qs = require('qs');
|
||||
var querystring = require('querystring');
|
||||
|
||||
/**
|
||||
* Return strong ETag for `body`.
|
||||
*
|
||||
* @param {String|Buffer} body
|
||||
* @param {String} [encoding]
|
||||
* @return {String}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
exports.etag = function (body, encoding) {
|
||||
var buf = !Buffer.isBuffer(body)
|
||||
? new Buffer(body, encoding)
|
||||
: body;
|
||||
|
||||
return etag(buf, {weak: false});
|
||||
};
|
||||
|
||||
/**
|
||||
* Return weak ETag for `body`.
|
||||
*
|
||||
* @param {String|Buffer} body
|
||||
* @param {String} [encoding]
|
||||
* @return {String}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
exports.wetag = function wetag(body, encoding){
|
||||
var buf = !Buffer.isBuffer(body)
|
||||
? new Buffer(body, encoding)
|
||||
: body;
|
||||
|
||||
return etag(buf, {weak: true});
|
||||
};
|
||||
|
||||
/**
|
||||
* Check if `path` looks absolute.
|
||||
*
|
||||
* @param {String} path
|
||||
* @return {Boolean}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
exports.isAbsolute = function(path){
|
||||
if ('/' == path[0]) return true;
|
||||
if (':' == path[1] && '\\' == path[2]) return true;
|
||||
if ('\\\\' == path.substring(0, 2)) return true; // Microsoft Azure absolute path
|
||||
};
|
||||
|
||||
/**
|
||||
* Flatten the given `arr`.
|
||||
*
|
||||
* @param {Array} arr
|
||||
* @return {Array}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
exports.flatten = deprecate.function(flatten,
|
||||
'utils.flatten: use array-flatten npm module instead');
|
||||
|
||||
/**
|
||||
* Normalize the given `type`, for example "html" becomes "text/html".
|
||||
*
|
||||
* @param {String} type
|
||||
* @return {Object}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
exports.normalizeType = function(type){
|
||||
return ~type.indexOf('/')
|
||||
? acceptParams(type)
|
||||
: { value: mime.lookup(type), params: {} };
|
||||
};
|
||||
|
||||
/**
|
||||
* Normalize `types`, for example "html" becomes "text/html".
|
||||
*
|
||||
* @param {Array} types
|
||||
* @return {Array}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
exports.normalizeTypes = function(types){
|
||||
var ret = [];
|
||||
|
||||
for (var i = 0; i < types.length; ++i) {
|
||||
ret.push(exports.normalizeType(types[i]));
|
||||
}
|
||||
|
||||
return ret;
|
||||
};
|
||||
|
||||
/**
|
||||
* Generate Content-Disposition header appropriate for the filename.
|
||||
* non-ascii filenames are urlencoded and a filename* parameter is added
|
||||
*
|
||||
* @param {String} filename
|
||||
* @return {String}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
exports.contentDisposition = deprecate.function(contentDisposition,
|
||||
'utils.contentDisposition: use content-disposition npm module instead');
|
||||
|
||||
/**
|
||||
* Parse accept params `str` returning an
|
||||
* object with `.value`, `.quality` and `.params`.
|
||||
* also includes `.originalIndex` for stable sorting
|
||||
*
|
||||
* @param {String} str
|
||||
* @return {Object}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function acceptParams(str, index) {
|
||||
var parts = str.split(/ *; */);
|
||||
var ret = { value: parts[0], quality: 1, params: {}, originalIndex: index };
|
||||
|
||||
for (var i = 1; i < parts.length; ++i) {
|
||||
var pms = parts[i].split(/ *= */);
|
||||
if ('q' == pms[0]) {
|
||||
ret.quality = parseFloat(pms[1]);
|
||||
} else {
|
||||
ret.params[pms[0]] = pms[1];
|
||||
}
|
||||
}
|
||||
|
||||
return ret;
|
||||
}
|
||||
|
||||
/**
|
||||
* Compile "etag" value to function.
|
||||
*
|
||||
* @param {Boolean|String|Function} val
|
||||
* @return {Function}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
exports.compileETag = function(val) {
|
||||
var fn;
|
||||
|
||||
if (typeof val === 'function') {
|
||||
return val;
|
||||
}
|
||||
|
||||
switch (val) {
|
||||
case true:
|
||||
fn = exports.wetag;
|
||||
break;
|
||||
case false:
|
||||
break;
|
||||
case 'strong':
|
||||
fn = exports.etag;
|
||||
break;
|
||||
case 'weak':
|
||||
fn = exports.wetag;
|
||||
break;
|
||||
default:
|
||||
throw new TypeError('unknown value for etag function: ' + val);
|
||||
}
|
||||
|
||||
return fn;
|
||||
}
|
||||
|
||||
/**
|
||||
* Compile "query parser" value to function.
|
||||
*
|
||||
* @param {String|Function} val
|
||||
* @return {Function}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
exports.compileQueryParser = function compileQueryParser(val) {
|
||||
var fn;
|
||||
|
||||
if (typeof val === 'function') {
|
||||
return val;
|
||||
}
|
||||
|
||||
switch (val) {
|
||||
case true:
|
||||
fn = querystring.parse;
|
||||
break;
|
||||
case false:
|
||||
fn = newObject;
|
||||
break;
|
||||
case 'extended':
|
||||
fn = parseExtendedQueryString;
|
||||
break;
|
||||
case 'simple':
|
||||
fn = querystring.parse;
|
||||
break;
|
||||
default:
|
||||
throw new TypeError('unknown value for query parser function: ' + val);
|
||||
}
|
||||
|
||||
return fn;
|
||||
}
|
||||
|
||||
/**
|
||||
* Compile "proxy trust" value to function.
|
||||
*
|
||||
* @param {Boolean|String|Number|Array|Function} val
|
||||
* @return {Function}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
exports.compileTrust = function(val) {
|
||||
if (typeof val === 'function') return val;
|
||||
|
||||
if (val === true) {
|
||||
// Support plain true/false
|
||||
return function(){ return true };
|
||||
}
|
||||
|
||||
if (typeof val === 'number') {
|
||||
// Support trusting hop count
|
||||
return function(a, i){ return i < val };
|
||||
}
|
||||
|
||||
if (typeof val === 'string') {
|
||||
// Support comma-separated values
|
||||
val = val.split(/ *, */);
|
||||
}
|
||||
|
||||
return proxyaddr.compile(val || []);
|
||||
}
|
||||
|
||||
/**
|
||||
* Set the charset in a given Content-Type string.
|
||||
*
|
||||
* @param {String} type
|
||||
* @param {String} charset
|
||||
* @return {String}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
exports.setCharset = function setCharset(type, charset) {
|
||||
if (!type || !charset) {
|
||||
return type;
|
||||
}
|
||||
|
||||
// parse type
|
||||
var parsed = contentType.parse(type);
|
||||
|
||||
// set charset
|
||||
parsed.parameters.charset = charset;
|
||||
|
||||
// format type
|
||||
return contentType.format(parsed);
|
||||
};
|
||||
|
||||
/**
|
||||
* Parse an extended query string with qs.
|
||||
*
|
||||
* @return {Object}
|
||||
* @private
|
||||
*/
|
||||
|
||||
function parseExtendedQueryString(str) {
|
||||
return qs.parse(str, {
|
||||
allowDots: false,
|
||||
allowPrototypes: true
|
||||
});
|
||||
}
|
||||
|
||||
/**
|
||||
* Return new empty object.
|
||||
*
|
||||
* @return {Object}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function newObject() {
|
||||
return {};
|
||||
}
|
173
node_modules/express/lib/view.js
generated
vendored
Normal file
173
node_modules/express/lib/view.js
generated
vendored
Normal file
@ -0,0 +1,173 @@
|
||||
/*!
|
||||
* express
|
||||
* Copyright(c) 2009-2013 TJ Holowaychuk
|
||||
* Copyright(c) 2013 Roman Shtylman
|
||||
* Copyright(c) 2014-2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict';
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var debug = require('debug')('express:view');
|
||||
var path = require('path');
|
||||
var fs = require('fs');
|
||||
var utils = require('./utils');
|
||||
|
||||
/**
|
||||
* Module variables.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var dirname = path.dirname;
|
||||
var basename = path.basename;
|
||||
var extname = path.extname;
|
||||
var join = path.join;
|
||||
var resolve = path.resolve;
|
||||
|
||||
/**
|
||||
* Module exports.
|
||||
* @public
|
||||
*/
|
||||
|
||||
module.exports = View;
|
||||
|
||||
/**
|
||||
* Initialize a new `View` with the given `name`.
|
||||
*
|
||||
* Options:
|
||||
*
|
||||
* - `defaultEngine` the default template engine name
|
||||
* - `engines` template engine require() cache
|
||||
* - `root` root path for view lookup
|
||||
*
|
||||
* @param {string} name
|
||||
* @param {object} options
|
||||
* @public
|
||||
*/
|
||||
|
||||
function View(name, options) {
|
||||
var opts = options || {};
|
||||
|
||||
this.defaultEngine = opts.defaultEngine;
|
||||
this.ext = extname(name);
|
||||
this.name = name;
|
||||
this.root = opts.root;
|
||||
|
||||
if (!this.ext && !this.defaultEngine) {
|
||||
throw new Error('No default engine was specified and no extension was provided.');
|
||||
}
|
||||
|
||||
var fileName = name;
|
||||
|
||||
if (!this.ext) {
|
||||
// get extension from default engine name
|
||||
this.ext = this.defaultEngine[0] !== '.'
|
||||
? '.' + this.defaultEngine
|
||||
: this.defaultEngine;
|
||||
|
||||
fileName += this.ext;
|
||||
}
|
||||
|
||||
if (!opts.engines[this.ext]) {
|
||||
// load engine
|
||||
opts.engines[this.ext] = require(this.ext.substr(1)).__express;
|
||||
}
|
||||
|
||||
// store loaded engine
|
||||
this.engine = opts.engines[this.ext];
|
||||
|
||||
// lookup path
|
||||
this.path = this.lookup(fileName);
|
||||
}
|
||||
|
||||
/**
|
||||
* Lookup view by the given `name`
|
||||
*
|
||||
* @param {string} name
|
||||
* @private
|
||||
*/
|
||||
|
||||
View.prototype.lookup = function lookup(name) {
|
||||
var path;
|
||||
var roots = [].concat(this.root);
|
||||
|
||||
debug('lookup "%s"', name);
|
||||
|
||||
for (var i = 0; i < roots.length && !path; i++) {
|
||||
var root = roots[i];
|
||||
|
||||
// resolve the path
|
||||
var loc = resolve(root, name);
|
||||
var dir = dirname(loc);
|
||||
var file = basename(loc);
|
||||
|
||||
// resolve the file
|
||||
path = this.resolve(dir, file);
|
||||
}
|
||||
|
||||
return path;
|
||||
};
|
||||
|
||||
/**
|
||||
* Render with the given options.
|
||||
*
|
||||
* @param {object} options
|
||||
* @param {function} callback
|
||||
* @private
|
||||
*/
|
||||
|
||||
View.prototype.render = function render(options, callback) {
|
||||
debug('render "%s"', this.path);
|
||||
this.engine(this.path, options, callback);
|
||||
};
|
||||
|
||||
/**
|
||||
* Resolve the file within the given directory.
|
||||
*
|
||||
* @param {string} dir
|
||||
* @param {string} file
|
||||
* @private
|
||||
*/
|
||||
|
||||
View.prototype.resolve = function resolve(dir, file) {
|
||||
var ext = this.ext;
|
||||
|
||||
// <path>.<ext>
|
||||
var path = join(dir, file);
|
||||
var stat = tryStat(path);
|
||||
|
||||
if (stat && stat.isFile()) {
|
||||
return path;
|
||||
}
|
||||
|
||||
// <path>/index.<ext>
|
||||
path = join(dir, basename(file, ext), 'index' + ext);
|
||||
stat = tryStat(path);
|
||||
|
||||
if (stat && stat.isFile()) {
|
||||
return path;
|
||||
}
|
||||
};
|
||||
|
||||
/**
|
||||
* Return a stat, maybe.
|
||||
*
|
||||
* @param {string} path
|
||||
* @return {fs.Stats}
|
||||
* @private
|
||||
*/
|
||||
|
||||
function tryStat(path) {
|
||||
debug('stat "%s"', path);
|
||||
|
||||
try {
|
||||
return fs.statSync(path);
|
||||
} catch (e) {
|
||||
return undefined;
|
||||
}
|
||||
}
|
170
node_modules/express/node_modules/accepts/HISTORY.md
generated
vendored
Normal file
170
node_modules/express/node_modules/accepts/HISTORY.md
generated
vendored
Normal file
@ -0,0 +1,170 @@
|
||||
1.2.13 / 2015-09-06
|
||||
===================
|
||||
|
||||
* deps: mime-types@~2.1.6
|
||||
- deps: mime-db@~1.18.0
|
||||
|
||||
1.2.12 / 2015-07-30
|
||||
===================
|
||||
|
||||
* deps: mime-types@~2.1.4
|
||||
- deps: mime-db@~1.16.0
|
||||
|
||||
1.2.11 / 2015-07-16
|
||||
===================
|
||||
|
||||
* deps: mime-types@~2.1.3
|
||||
- deps: mime-db@~1.15.0
|
||||
|
||||
1.2.10 / 2015-07-01
|
||||
===================
|
||||
|
||||
* deps: mime-types@~2.1.2
|
||||
- deps: mime-db@~1.14.0
|
||||
|
||||
1.2.9 / 2015-06-08
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.1.1
|
||||
- perf: fix deopt during mapping
|
||||
|
||||
1.2.8 / 2015-06-07
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.1.0
|
||||
- deps: mime-db@~1.13.0
|
||||
* perf: avoid argument reassignment & argument slice
|
||||
* perf: avoid negotiator recursive construction
|
||||
* perf: enable strict mode
|
||||
* perf: remove unnecessary bitwise operator
|
||||
|
||||
1.2.7 / 2015-05-10
|
||||
==================
|
||||
|
||||
* deps: negotiator@0.5.3
|
||||
- Fix media type parameter matching to be case-insensitive
|
||||
|
||||
1.2.6 / 2015-05-07
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.11
|
||||
- deps: mime-db@~1.9.1
|
||||
* deps: negotiator@0.5.2
|
||||
- Fix comparing media types with quoted values
|
||||
- Fix splitting media types with quoted commas
|
||||
|
||||
1.2.5 / 2015-03-13
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.10
|
||||
- deps: mime-db@~1.8.0
|
||||
|
||||
1.2.4 / 2015-02-14
|
||||
==================
|
||||
|
||||
* Support Node.js 0.6
|
||||
* deps: mime-types@~2.0.9
|
||||
- deps: mime-db@~1.7.0
|
||||
* deps: negotiator@0.5.1
|
||||
- Fix preference sorting to be stable for long acceptable lists
|
||||
|
||||
1.2.3 / 2015-01-31
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.8
|
||||
- deps: mime-db@~1.6.0
|
||||
|
||||
1.2.2 / 2014-12-30
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.7
|
||||
- deps: mime-db@~1.5.0
|
||||
|
||||
1.2.1 / 2014-12-30
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.5
|
||||
- deps: mime-db@~1.3.1
|
||||
|
||||
1.2.0 / 2014-12-19
|
||||
==================
|
||||
|
||||
* deps: negotiator@0.5.0
|
||||
- Fix list return order when large accepted list
|
||||
- Fix missing identity encoding when q=0 exists
|
||||
- Remove dynamic building of Negotiator class
|
||||
|
||||
1.1.4 / 2014-12-10
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.4
|
||||
- deps: mime-db@~1.3.0
|
||||
|
||||
1.1.3 / 2014-11-09
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.3
|
||||
- deps: mime-db@~1.2.0
|
||||
|
||||
1.1.2 / 2014-10-14
|
||||
==================
|
||||
|
||||
* deps: negotiator@0.4.9
|
||||
- Fix error when media type has invalid parameter
|
||||
|
||||
1.1.1 / 2014-09-28
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.0.2
|
||||
- deps: mime-db@~1.1.0
|
||||
* deps: negotiator@0.4.8
|
||||
- Fix all negotiations to be case-insensitive
|
||||
- Stable sort preferences of same quality according to client order
|
||||
|
||||
1.1.0 / 2014-09-02
|
||||
==================
|
||||
|
||||
* update `mime-types`
|
||||
|
||||
1.0.7 / 2014-07-04
|
||||
==================
|
||||
|
||||
* Fix wrong type returned from `type` when match after unknown extension
|
||||
|
||||
1.0.6 / 2014-06-24
|
||||
==================
|
||||
|
||||
* deps: negotiator@0.4.7
|
||||
|
||||
1.0.5 / 2014-06-20
|
||||
==================
|
||||
|
||||
* fix crash when unknown extension given
|
||||
|
||||
1.0.4 / 2014-06-19
|
||||
==================
|
||||
|
||||
* use `mime-types`
|
||||
|
||||
1.0.3 / 2014-06-11
|
||||
==================
|
||||
|
||||
* deps: negotiator@0.4.6
|
||||
- Order by specificity when quality is the same
|
||||
|
||||
1.0.2 / 2014-05-29
|
||||
==================
|
||||
|
||||
* Fix interpretation when header not in request
|
||||
* deps: pin negotiator@0.4.5
|
||||
|
||||
1.0.1 / 2014-01-18
|
||||
==================
|
||||
|
||||
* Identity encoding isn't always acceptable
|
||||
* deps: negotiator@~0.4.0
|
||||
|
||||
1.0.0 / 2013-12-27
|
||||
==================
|
||||
|
||||
* Genesis
|
23
node_modules/express/node_modules/accepts/LICENSE
generated
vendored
Normal file
23
node_modules/express/node_modules/accepts/LICENSE
generated
vendored
Normal file
@ -0,0 +1,23 @@
|
||||
(The MIT License)
|
||||
|
||||
Copyright (c) 2014 Jonathan Ong <me@jongleberry.com>
|
||||
Copyright (c) 2015 Douglas Christopher Wilson <doug@somethingdoug.com>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of this software and associated documentation files (the
|
||||
'Software'), to deal in the Software without restriction, including
|
||||
without limitation the rights to use, copy, modify, merge, publish,
|
||||
distribute, sublicense, and/or sell copies of the Software, and to
|
||||
permit persons to whom the Software is furnished to do so, subject to
|
||||
the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
|
||||
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
|
||||
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
|
||||
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
|
||||
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
135
node_modules/express/node_modules/accepts/README.md
generated
vendored
Normal file
135
node_modules/express/node_modules/accepts/README.md
generated
vendored
Normal file
@ -0,0 +1,135 @@
|
||||
# accepts
|
||||
|
||||
[![NPM Version][npm-image]][npm-url]
|
||||
[![NPM Downloads][downloads-image]][downloads-url]
|
||||
[![Node.js Version][node-version-image]][node-version-url]
|
||||
[![Build Status][travis-image]][travis-url]
|
||||
[![Test Coverage][coveralls-image]][coveralls-url]
|
||||
|
||||
Higher level content negotiation based on [negotiator](https://www.npmjs.com/package/negotiator). Extracted from [koa](https://www.npmjs.com/package/koa) for general use.
|
||||
|
||||
In addition to negotiator, it allows:
|
||||
|
||||
- Allows types as an array or arguments list, ie `(['text/html', 'application/json'])` as well as `('text/html', 'application/json')`.
|
||||
- Allows type shorthands such as `json`.
|
||||
- Returns `false` when no types match
|
||||
- Treats non-existent headers as `*`
|
||||
|
||||
## Installation
|
||||
|
||||
```sh
|
||||
npm install accepts
|
||||
```
|
||||
|
||||
## API
|
||||
|
||||
```js
|
||||
var accepts = require('accepts')
|
||||
```
|
||||
|
||||
### accepts(req)
|
||||
|
||||
Create a new `Accepts` object for the given `req`.
|
||||
|
||||
#### .charset(charsets)
|
||||
|
||||
Return the first accepted charset. If nothing in `charsets` is accepted,
|
||||
then `false` is returned.
|
||||
|
||||
#### .charsets()
|
||||
|
||||
Return the charsets that the request accepts, in the order of the client's
|
||||
preference (most preferred first).
|
||||
|
||||
#### .encoding(encodings)
|
||||
|
||||
Return the first accepted encoding. If nothing in `encodings` is accepted,
|
||||
then `false` is returned.
|
||||
|
||||
#### .encodings()
|
||||
|
||||
Return the encodings that the request accepts, in the order of the client's
|
||||
preference (most preferred first).
|
||||
|
||||
#### .language(languages)
|
||||
|
||||
Return the first accepted language. If nothing in `languages` is accepted,
|
||||
then `false` is returned.
|
||||
|
||||
#### .languages()
|
||||
|
||||
Return the languages that the request accepts, in the order of the client's
|
||||
preference (most preferred first).
|
||||
|
||||
#### .type(types)
|
||||
|
||||
Return the first accepted type (and it is returned as the same text as what
|
||||
appears in the `types` array). If nothing in `types` is accepted, then `false`
|
||||
is returned.
|
||||
|
||||
The `types` array can contain full MIME types or file extensions. Any value
|
||||
that is not a full MIME types is passed to `require('mime-types').lookup`.
|
||||
|
||||
#### .types()
|
||||
|
||||
Return the types that the request accepts, in the order of the client's
|
||||
preference (most preferred first).
|
||||
|
||||
## Examples
|
||||
|
||||
### Simple type negotiation
|
||||
|
||||
This simple example shows how to use `accepts` to return a different typed
|
||||
respond body based on what the client wants to accept. The server lists it's
|
||||
preferences in order and will get back the best match between the client and
|
||||
server.
|
||||
|
||||
```js
|
||||
var accepts = require('accepts')
|
||||
var http = require('http')
|
||||
|
||||
function app(req, res) {
|
||||
var accept = accepts(req)
|
||||
|
||||
// the order of this list is significant; should be server preferred order
|
||||
switch(accept.type(['json', 'html'])) {
|
||||
case 'json':
|
||||
res.setHeader('Content-Type', 'application/json')
|
||||
res.write('{"hello":"world!"}')
|
||||
break
|
||||
case 'html':
|
||||
res.setHeader('Content-Type', 'text/html')
|
||||
res.write('<b>hello, world!</b>')
|
||||
break
|
||||
default:
|
||||
// the fallback is text/plain, so no need to specify it above
|
||||
res.setHeader('Content-Type', 'text/plain')
|
||||
res.write('hello, world!')
|
||||
break
|
||||
}
|
||||
|
||||
res.end()
|
||||
}
|
||||
|
||||
http.createServer(app).listen(3000)
|
||||
```
|
||||
|
||||
You can test this out with the cURL program:
|
||||
```sh
|
||||
curl -I -H'Accept: text/html' http://localhost:3000/
|
||||
```
|
||||
|
||||
## License
|
||||
|
||||
[MIT](LICENSE)
|
||||
|
||||
[npm-image]: https://img.shields.io/npm/v/accepts.svg
|
||||
[npm-url]: https://npmjs.org/package/accepts
|
||||
[node-version-image]: https://img.shields.io/node/v/accepts.svg
|
||||
[node-version-url]: http://nodejs.org/download/
|
||||
[travis-image]: https://img.shields.io/travis/jshttp/accepts/master.svg
|
||||
[travis-url]: https://travis-ci.org/jshttp/accepts
|
||||
[coveralls-image]: https://img.shields.io/coveralls/jshttp/accepts/master.svg
|
||||
[coveralls-url]: https://coveralls.io/r/jshttp/accepts
|
||||
[downloads-image]: https://img.shields.io/npm/dm/accepts.svg
|
||||
[downloads-url]: https://npmjs.org/package/accepts
|
231
node_modules/express/node_modules/accepts/index.js
generated
vendored
Normal file
231
node_modules/express/node_modules/accepts/index.js
generated
vendored
Normal file
@ -0,0 +1,231 @@
|
||||
/*!
|
||||
* accepts
|
||||
* Copyright(c) 2014 Jonathan Ong
|
||||
* Copyright(c) 2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict'
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var Negotiator = require('negotiator')
|
||||
var mime = require('mime-types')
|
||||
|
||||
/**
|
||||
* Module exports.
|
||||
* @public
|
||||
*/
|
||||
|
||||
module.exports = Accepts
|
||||
|
||||
/**
|
||||
* Create a new Accepts object for the given req.
|
||||
*
|
||||
* @param {object} req
|
||||
* @public
|
||||
*/
|
||||
|
||||
function Accepts(req) {
|
||||
if (!(this instanceof Accepts))
|
||||
return new Accepts(req)
|
||||
|
||||
this.headers = req.headers
|
||||
this.negotiator = new Negotiator(req)
|
||||
}
|
||||
|
||||
/**
|
||||
* Check if the given `type(s)` is acceptable, returning
|
||||
* the best match when true, otherwise `undefined`, in which
|
||||
* case you should respond with 406 "Not Acceptable".
|
||||
*
|
||||
* The `type` value may be a single mime type string
|
||||
* such as "application/json", the extension name
|
||||
* such as "json" or an array `["json", "html", "text/plain"]`. When a list
|
||||
* or array is given the _best_ match, if any is returned.
|
||||
*
|
||||
* Examples:
|
||||
*
|
||||
* // Accept: text/html
|
||||
* this.types('html');
|
||||
* // => "html"
|
||||
*
|
||||
* // Accept: text/*, application/json
|
||||
* this.types('html');
|
||||
* // => "html"
|
||||
* this.types('text/html');
|
||||
* // => "text/html"
|
||||
* this.types('json', 'text');
|
||||
* // => "json"
|
||||
* this.types('application/json');
|
||||
* // => "application/json"
|
||||
*
|
||||
* // Accept: text/*, application/json
|
||||
* this.types('image/png');
|
||||
* this.types('png');
|
||||
* // => undefined
|
||||
*
|
||||
* // Accept: text/*;q=.5, application/json
|
||||
* this.types(['html', 'json']);
|
||||
* this.types('html', 'json');
|
||||
* // => "json"
|
||||
*
|
||||
* @param {String|Array} types...
|
||||
* @return {String|Array|Boolean}
|
||||
* @public
|
||||
*/
|
||||
|
||||
Accepts.prototype.type =
|
||||
Accepts.prototype.types = function (types_) {
|
||||
var types = types_
|
||||
|
||||
// support flattened arguments
|
||||
if (types && !Array.isArray(types)) {
|
||||
types = new Array(arguments.length)
|
||||
for (var i = 0; i < types.length; i++) {
|
||||
types[i] = arguments[i]
|
||||
}
|
||||
}
|
||||
|
||||
// no types, return all requested types
|
||||
if (!types || types.length === 0) {
|
||||
return this.negotiator.mediaTypes()
|
||||
}
|
||||
|
||||
if (!this.headers.accept) return types[0];
|
||||
var mimes = types.map(extToMime);
|
||||
var accepts = this.negotiator.mediaTypes(mimes.filter(validMime));
|
||||
var first = accepts[0];
|
||||
if (!first) return false;
|
||||
return types[mimes.indexOf(first)];
|
||||
}
|
||||
|
||||
/**
|
||||
* Return accepted encodings or best fit based on `encodings`.
|
||||
*
|
||||
* Given `Accept-Encoding: gzip, deflate`
|
||||
* an array sorted by quality is returned:
|
||||
*
|
||||
* ['gzip', 'deflate']
|
||||
*
|
||||
* @param {String|Array} encodings...
|
||||
* @return {String|Array}
|
||||
* @public
|
||||
*/
|
||||
|
||||
Accepts.prototype.encoding =
|
||||
Accepts.prototype.encodings = function (encodings_) {
|
||||
var encodings = encodings_
|
||||
|
||||
// support flattened arguments
|
||||
if (encodings && !Array.isArray(encodings)) {
|
||||
encodings = new Array(arguments.length)
|
||||
for (var i = 0; i < encodings.length; i++) {
|
||||
encodings[i] = arguments[i]
|
||||
}
|
||||
}
|
||||
|
||||
// no encodings, return all requested encodings
|
||||
if (!encodings || encodings.length === 0) {
|
||||
return this.negotiator.encodings()
|
||||
}
|
||||
|
||||
return this.negotiator.encodings(encodings)[0] || false
|
||||
}
|
||||
|
||||
/**
|
||||
* Return accepted charsets or best fit based on `charsets`.
|
||||
*
|
||||
* Given `Accept-Charset: utf-8, iso-8859-1;q=0.2, utf-7;q=0.5`
|
||||
* an array sorted by quality is returned:
|
||||
*
|
||||
* ['utf-8', 'utf-7', 'iso-8859-1']
|
||||
*
|
||||
* @param {String|Array} charsets...
|
||||
* @return {String|Array}
|
||||
* @public
|
||||
*/
|
||||
|
||||
Accepts.prototype.charset =
|
||||
Accepts.prototype.charsets = function (charsets_) {
|
||||
var charsets = charsets_
|
||||
|
||||
// support flattened arguments
|
||||
if (charsets && !Array.isArray(charsets)) {
|
||||
charsets = new Array(arguments.length)
|
||||
for (var i = 0; i < charsets.length; i++) {
|
||||
charsets[i] = arguments[i]
|
||||
}
|
||||
}
|
||||
|
||||
// no charsets, return all requested charsets
|
||||
if (!charsets || charsets.length === 0) {
|
||||
return this.negotiator.charsets()
|
||||
}
|
||||
|
||||
return this.negotiator.charsets(charsets)[0] || false
|
||||
}
|
||||
|
||||
/**
|
||||
* Return accepted languages or best fit based on `langs`.
|
||||
*
|
||||
* Given `Accept-Language: en;q=0.8, es, pt`
|
||||
* an array sorted by quality is returned:
|
||||
*
|
||||
* ['es', 'pt', 'en']
|
||||
*
|
||||
* @param {String|Array} langs...
|
||||
* @return {Array|String}
|
||||
* @public
|
||||
*/
|
||||
|
||||
Accepts.prototype.lang =
|
||||
Accepts.prototype.langs =
|
||||
Accepts.prototype.language =
|
||||
Accepts.prototype.languages = function (languages_) {
|
||||
var languages = languages_
|
||||
|
||||
// support flattened arguments
|
||||
if (languages && !Array.isArray(languages)) {
|
||||
languages = new Array(arguments.length)
|
||||
for (var i = 0; i < languages.length; i++) {
|
||||
languages[i] = arguments[i]
|
||||
}
|
||||
}
|
||||
|
||||
// no languages, return all requested languages
|
||||
if (!languages || languages.length === 0) {
|
||||
return this.negotiator.languages()
|
||||
}
|
||||
|
||||
return this.negotiator.languages(languages)[0] || false
|
||||
}
|
||||
|
||||
/**
|
||||
* Convert extnames to mime.
|
||||
*
|
||||
* @param {String} type
|
||||
* @return {String}
|
||||
* @private
|
||||
*/
|
||||
|
||||
function extToMime(type) {
|
||||
return type.indexOf('/') === -1
|
||||
? mime.lookup(type)
|
||||
: type
|
||||
}
|
||||
|
||||
/**
|
||||
* Check if mime is valid.
|
||||
*
|
||||
* @param {String} type
|
||||
* @return {String}
|
||||
* @private
|
||||
*/
|
||||
|
||||
function validMime(type) {
|
||||
return typeof type === 'string';
|
||||
}
|
183
node_modules/express/node_modules/accepts/node_modules/mime-types/HISTORY.md
generated
vendored
Normal file
183
node_modules/express/node_modules/accepts/node_modules/mime-types/HISTORY.md
generated
vendored
Normal file
@ -0,0 +1,183 @@
|
||||
2.1.9 / 2016-01-06
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.21.0
|
||||
- Add new mime types
|
||||
|
||||
2.1.8 / 2015-11-30
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.20.0
|
||||
- Add new mime types
|
||||
|
||||
2.1.7 / 2015-09-20
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.19.0
|
||||
- Add new mime types
|
||||
|
||||
2.1.6 / 2015-09-03
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.18.0
|
||||
- Add new mime types
|
||||
|
||||
2.1.5 / 2015-08-20
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.17.0
|
||||
- Add new mime types
|
||||
|
||||
2.1.4 / 2015-07-30
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.16.0
|
||||
- Add new mime types
|
||||
|
||||
2.1.3 / 2015-07-13
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.15.0
|
||||
- Add new mime types
|
||||
|
||||
2.1.2 / 2015-06-25
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.14.0
|
||||
- Add new mime types
|
||||
|
||||
2.1.1 / 2015-06-08
|
||||
==================
|
||||
|
||||
* perf: fix deopt during mapping
|
||||
|
||||
2.1.0 / 2015-06-07
|
||||
==================
|
||||
|
||||
* Fix incorrectly treating extension-less file name as extension
|
||||
- i.e. `'path/to/json'` will no longer return `application/json`
|
||||
* Fix `.charset(type)` to accept parameters
|
||||
* Fix `.charset(type)` to match case-insensitive
|
||||
* Improve generation of extension to MIME mapping
|
||||
* Refactor internals for readability and no argument reassignment
|
||||
* Prefer `application/*` MIME types from the same source
|
||||
* Prefer any type over `application/octet-stream`
|
||||
* deps: mime-db@~1.13.0
|
||||
- Add nginx as a source
|
||||
- Add new mime types
|
||||
|
||||
2.0.14 / 2015-06-06
|
||||
===================
|
||||
|
||||
* deps: mime-db@~1.12.0
|
||||
- Add new mime types
|
||||
|
||||
2.0.13 / 2015-05-31
|
||||
===================
|
||||
|
||||
* deps: mime-db@~1.11.0
|
||||
- Add new mime types
|
||||
|
||||
2.0.12 / 2015-05-19
|
||||
===================
|
||||
|
||||
* deps: mime-db@~1.10.0
|
||||
- Add new mime types
|
||||
|
||||
2.0.11 / 2015-05-05
|
||||
===================
|
||||
|
||||
* deps: mime-db@~1.9.1
|
||||
- Add new mime types
|
||||
|
||||
2.0.10 / 2015-03-13
|
||||
===================
|
||||
|
||||
* deps: mime-db@~1.8.0
|
||||
- Add new mime types
|
||||
|
||||
2.0.9 / 2015-02-09
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.7.0
|
||||
- Add new mime types
|
||||
- Community extensions ownership transferred from `node-mime`
|
||||
|
||||
2.0.8 / 2015-01-29
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.6.0
|
||||
- Add new mime types
|
||||
|
||||
2.0.7 / 2014-12-30
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.5.0
|
||||
- Add new mime types
|
||||
- Fix various invalid MIME type entries
|
||||
|
||||
2.0.6 / 2014-12-30
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.4.0
|
||||
- Add new mime types
|
||||
- Fix various invalid MIME type entries
|
||||
- Remove example template MIME types
|
||||
|
||||
2.0.5 / 2014-12-29
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.3.1
|
||||
- Fix missing extensions
|
||||
|
||||
2.0.4 / 2014-12-10
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.3.0
|
||||
- Add new mime types
|
||||
|
||||
2.0.3 / 2014-11-09
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.2.0
|
||||
- Add new mime types
|
||||
|
||||
2.0.2 / 2014-09-28
|
||||
==================
|
||||
|
||||
* deps: mime-db@~1.1.0
|
||||
- Add new mime types
|
||||
- Add additional compressible
|
||||
- Update charsets
|
||||
|
||||
2.0.1 / 2014-09-07
|
||||
==================
|
||||
|
||||
* Support Node.js 0.6
|
||||
|
||||
2.0.0 / 2014-09-02
|
||||
==================
|
||||
|
||||
* Use `mime-db`
|
||||
* Remove `.define()`
|
||||
|
||||
1.0.2 / 2014-08-04
|
||||
==================
|
||||
|
||||
* Set charset=utf-8 for `text/javascript`
|
||||
|
||||
1.0.1 / 2014-06-24
|
||||
==================
|
||||
|
||||
* Add `text/jsx` type
|
||||
|
||||
1.0.0 / 2014-05-12
|
||||
==================
|
||||
|
||||
* Return `false` for unknown types
|
||||
* Set charset=utf-8 for `application/json`
|
||||
|
||||
0.1.0 / 2014-05-02
|
||||
==================
|
||||
|
||||
* Initial release
|
23
node_modules/express/node_modules/accepts/node_modules/mime-types/LICENSE
generated
vendored
Normal file
23
node_modules/express/node_modules/accepts/node_modules/mime-types/LICENSE
generated
vendored
Normal file
@ -0,0 +1,23 @@
|
||||
(The MIT License)
|
||||
|
||||
Copyright (c) 2014 Jonathan Ong <me@jongleberry.com>
|
||||
Copyright (c) 2015 Douglas Christopher Wilson <doug@somethingdoug.com>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of this software and associated documentation files (the
|
||||
'Software'), to deal in the Software without restriction, including
|
||||
without limitation the rights to use, copy, modify, merge, publish,
|
||||
distribute, sublicense, and/or sell copies of the Software, and to
|
||||
permit persons to whom the Software is furnished to do so, subject to
|
||||
the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
|
||||
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
|
||||
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
|
||||
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
|
||||
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
103
node_modules/express/node_modules/accepts/node_modules/mime-types/README.md
generated
vendored
Normal file
103
node_modules/express/node_modules/accepts/node_modules/mime-types/README.md
generated
vendored
Normal file
@ -0,0 +1,103 @@
|
||||
# mime-types
|
||||
|
||||
[![NPM Version][npm-image]][npm-url]
|
||||
[![NPM Downloads][downloads-image]][downloads-url]
|
||||
[![Node.js Version][node-version-image]][node-version-url]
|
||||
[![Build Status][travis-image]][travis-url]
|
||||
[![Test Coverage][coveralls-image]][coveralls-url]
|
||||
|
||||
The ultimate javascript content-type utility.
|
||||
|
||||
Similar to [node-mime](https://github.com/broofa/node-mime), except:
|
||||
|
||||
- __No fallbacks.__ Instead of naively returning the first available type, `mime-types` simply returns `false`,
|
||||
so do `var type = mime.lookup('unrecognized') || 'application/octet-stream'`.
|
||||
- No `new Mime()` business, so you could do `var lookup = require('mime-types').lookup`.
|
||||
- Additional mime types are added such as jade and stylus via [mime-db](https://github.com/jshttp/mime-db)
|
||||
- No `.define()` functionality
|
||||
|
||||
Otherwise, the API is compatible.
|
||||
|
||||
## Install
|
||||
|
||||
```sh
|
||||
$ npm install mime-types
|
||||
```
|
||||
|
||||
## Adding Types
|
||||
|
||||
All mime types are based on [mime-db](https://github.com/jshttp/mime-db),
|
||||
so open a PR there if you'd like to add mime types.
|
||||
|
||||
## API
|
||||
|
||||
```js
|
||||
var mime = require('mime-types')
|
||||
```
|
||||
|
||||
All functions return `false` if input is invalid or not found.
|
||||
|
||||
### mime.lookup(path)
|
||||
|
||||
Lookup the content-type associated with a file.
|
||||
|
||||
```js
|
||||
mime.lookup('json') // 'application/json'
|
||||
mime.lookup('.md') // 'text/x-markdown'
|
||||
mime.lookup('file.html') // 'text/html'
|
||||
mime.lookup('folder/file.js') // 'application/javascript'
|
||||
mime.lookup('folder/.htaccess') // false
|
||||
|
||||
mime.lookup('cats') // false
|
||||
```
|
||||
|
||||
### mime.contentType(type)
|
||||
|
||||
Create a full content-type header given a content-type or extension.
|
||||
|
||||
```js
|
||||
mime.contentType('markdown') // 'text/x-markdown; charset=utf-8'
|
||||
mime.contentType('file.json') // 'application/json; charset=utf-8'
|
||||
|
||||
// from a full path
|
||||
mime.contentType(path.extname('/path/to/file.json')) // 'application/json; charset=utf-8'
|
||||
```
|
||||
|
||||
### mime.extension(type)
|
||||
|
||||
Get the default extension for a content-type.
|
||||
|
||||
```js
|
||||
mime.extension('application/octet-stream') // 'bin'
|
||||
```
|
||||
|
||||
### mime.charset(type)
|
||||
|
||||
Lookup the implied default charset of a content-type.
|
||||
|
||||
```js
|
||||
mime.charset('text/x-markdown') // 'UTF-8'
|
||||
```
|
||||
|
||||
### var type = mime.types[extension]
|
||||
|
||||
A map of content-types by extension.
|
||||
|
||||
### [extensions...] = mime.extensions[type]
|
||||
|
||||
A map of extensions by content-type.
|
||||
|
||||
## License
|
||||
|
||||
[MIT](LICENSE)
|
||||
|
||||
[npm-image]: https://img.shields.io/npm/v/mime-types.svg
|
||||
[npm-url]: https://npmjs.org/package/mime-types
|
||||
[node-version-image]: https://img.shields.io/node/v/mime-types.svg
|
||||
[node-version-url]: http://nodejs.org/download/
|
||||
[travis-image]: https://img.shields.io/travis/jshttp/mime-types/master.svg
|
||||
[travis-url]: https://travis-ci.org/jshttp/mime-types
|
||||
[coveralls-image]: https://img.shields.io/coveralls/jshttp/mime-types/master.svg
|
||||
[coveralls-url]: https://coveralls.io/r/jshttp/mime-types
|
||||
[downloads-image]: https://img.shields.io/npm/dm/mime-types.svg
|
||||
[downloads-url]: https://npmjs.org/package/mime-types
|
188
node_modules/express/node_modules/accepts/node_modules/mime-types/index.js
generated
vendored
Normal file
188
node_modules/express/node_modules/accepts/node_modules/mime-types/index.js
generated
vendored
Normal file
@ -0,0 +1,188 @@
|
||||
/*!
|
||||
* mime-types
|
||||
* Copyright(c) 2014 Jonathan Ong
|
||||
* Copyright(c) 2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict'
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var db = require('mime-db')
|
||||
var extname = require('path').extname
|
||||
|
||||
/**
|
||||
* Module variables.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var extractTypeRegExp = /^\s*([^;\s]*)(?:;|\s|$)/
|
||||
var textTypeRegExp = /^text\//i
|
||||
|
||||
/**
|
||||
* Module exports.
|
||||
* @public
|
||||
*/
|
||||
|
||||
exports.charset = charset
|
||||
exports.charsets = { lookup: charset }
|
||||
exports.contentType = contentType
|
||||
exports.extension = extension
|
||||
exports.extensions = Object.create(null)
|
||||
exports.lookup = lookup
|
||||
exports.types = Object.create(null)
|
||||
|
||||
// Populate the extensions/types maps
|
||||
populateMaps(exports.extensions, exports.types)
|
||||
|
||||
/**
|
||||
* Get the default charset for a MIME type.
|
||||
*
|
||||
* @param {string} type
|
||||
* @return {boolean|string}
|
||||
*/
|
||||
|
||||
function charset(type) {
|
||||
if (!type || typeof type !== 'string') {
|
||||
return false
|
||||
}
|
||||
|
||||
// TODO: use media-typer
|
||||
var match = extractTypeRegExp.exec(type)
|
||||
var mime = match && db[match[1].toLowerCase()]
|
||||
|
||||
if (mime && mime.charset) {
|
||||
return mime.charset
|
||||
}
|
||||
|
||||
// default text/* to utf-8
|
||||
if (match && textTypeRegExp.test(match[1])) {
|
||||
return 'UTF-8'
|
||||
}
|
||||
|
||||
return false
|
||||
}
|
||||
|
||||
/**
|
||||
* Create a full Content-Type header given a MIME type or extension.
|
||||
*
|
||||
* @param {string} str
|
||||
* @return {boolean|string}
|
||||
*/
|
||||
|
||||
function contentType(str) {
|
||||
// TODO: should this even be in this module?
|
||||
if (!str || typeof str !== 'string') {
|
||||
return false
|
||||
}
|
||||
|
||||
var mime = str.indexOf('/') === -1
|
||||
? exports.lookup(str)
|
||||
: str
|
||||
|
||||
if (!mime) {
|
||||
return false
|
||||
}
|
||||
|
||||
// TODO: use content-type or other module
|
||||
if (mime.indexOf('charset') === -1) {
|
||||
var charset = exports.charset(mime)
|
||||
if (charset) mime += '; charset=' + charset.toLowerCase()
|
||||
}
|
||||
|
||||
return mime
|
||||
}
|
||||
|
||||
/**
|
||||
* Get the default extension for a MIME type.
|
||||
*
|
||||
* @param {string} type
|
||||
* @return {boolean|string}
|
||||
*/
|
||||
|
||||
function extension(type) {
|
||||
if (!type || typeof type !== 'string') {
|
||||
return false
|
||||
}
|
||||
|
||||
// TODO: use media-typer
|
||||
var match = extractTypeRegExp.exec(type)
|
||||
|
||||
// get extensions
|
||||
var exts = match && exports.extensions[match[1].toLowerCase()]
|
||||
|
||||
if (!exts || !exts.length) {
|
||||
return false
|
||||
}
|
||||
|
||||
return exts[0]
|
||||
}
|
||||
|
||||
/**
|
||||
* Lookup the MIME type for a file path/extension.
|
||||
*
|
||||
* @param {string} path
|
||||
* @return {boolean|string}
|
||||
*/
|
||||
|
||||
function lookup(path) {
|
||||
if (!path || typeof path !== 'string') {
|
||||
return false
|
||||
}
|
||||
|
||||
// get the extension ("ext" or ".ext" or full path)
|
||||
var extension = extname('x.' + path)
|
||||
.toLowerCase()
|
||||
.substr(1)
|
||||
|
||||
if (!extension) {
|
||||
return false
|
||||
}
|
||||
|
||||
return exports.types[extension] || false
|
||||
}
|
||||
|
||||
/**
|
||||
* Populate the extensions and types maps.
|
||||
* @private
|
||||
*/
|
||||
|
||||
function populateMaps(extensions, types) {
|
||||
// source preference (least -> most)
|
||||
var preference = ['nginx', 'apache', undefined, 'iana']
|
||||
|
||||
Object.keys(db).forEach(function forEachMimeType(type) {
|
||||
var mime = db[type]
|
||||
var exts = mime.extensions
|
||||
|
||||
if (!exts || !exts.length) {
|
||||
return
|
||||
}
|
||||
|
||||
// mime -> extensions
|
||||
extensions[type] = exts
|
||||
|
||||
// extension -> mime
|
||||
for (var i = 0; i < exts.length; i++) {
|
||||
var extension = exts[i]
|
||||
|
||||
if (types[extension]) {
|
||||
var from = preference.indexOf(db[types[extension]].source)
|
||||
var to = preference.indexOf(mime.source)
|
||||
|
||||
if (types[extension] !== 'application/octet-stream'
|
||||
&& from > to || (from === to && types[extension].substr(0, 12) === 'application/')) {
|
||||
// skip the remapping
|
||||
continue
|
||||
}
|
||||
}
|
||||
|
||||
// set the extension -> mime
|
||||
types[extension] = type
|
||||
}
|
||||
})
|
||||
}
|
306
node_modules/express/node_modules/accepts/node_modules/mime-types/node_modules/mime-db/HISTORY.md
generated
vendored
Normal file
306
node_modules/express/node_modules/accepts/node_modules/mime-types/node_modules/mime-db/HISTORY.md
generated
vendored
Normal file
@ -0,0 +1,306 @@
|
||||
1.21.0 / 2016-01-06
|
||||
===================
|
||||
|
||||
* Add `application/emergencycalldata.comment+xml`
|
||||
* Add `application/emergencycalldata.deviceinfo+xml`
|
||||
* Add `application/emergencycalldata.providerinfo+xml`
|
||||
* Add `application/emergencycalldata.serviceinfo+xml`
|
||||
* Add `application/emergencycalldata.subscriberinfo+xml`
|
||||
* Add `application/vnd.filmit.zfc`
|
||||
* Add `application/vnd.google-apps.document`
|
||||
* Add `application/vnd.google-apps.presentation`
|
||||
* Add `application/vnd.google-apps.spreadsheet`
|
||||
* Add `application/vnd.mapbox-vector-tile`
|
||||
* Add `application/vnd.ms-printdevicecapabilities+xml`
|
||||
* Add `application/vnd.ms-windows.devicepairing`
|
||||
* Add `application/vnd.ms-windows.nwprinting.oob`
|
||||
* Add `application/vnd.tml`
|
||||
* Add `audio/evs`
|
||||
|
||||
1.20.0 / 2015-11-10
|
||||
===================
|
||||
|
||||
* Add `application/cdni`
|
||||
* Add `application/csvm+json`
|
||||
* Add `application/rfc+xml`
|
||||
* Add `application/vnd.3gpp.access-transfer-events+xml`
|
||||
* Add `application/vnd.3gpp.srvcc-ext+xml`
|
||||
* Add `application/vnd.ms-windows.wsd.oob`
|
||||
* Add `application/vnd.oxli.countgraph`
|
||||
* Add `application/vnd.pagerduty+json`
|
||||
* Add `text/x-suse-ymp`
|
||||
|
||||
1.19.0 / 2015-09-17
|
||||
===================
|
||||
|
||||
* Add `application/vnd.3gpp-prose-pc3ch+xml`
|
||||
* Add `application/vnd.3gpp.srvcc-info+xml`
|
||||
* Add `application/vnd.apple.pkpass`
|
||||
* Add `application/vnd.drive+json`
|
||||
|
||||
1.18.0 / 2015-09-03
|
||||
===================
|
||||
|
||||
* Add `application/pkcs12`
|
||||
* Add `application/vnd.3gpp-prose+xml`
|
||||
* Add `application/vnd.3gpp.mid-call+xml`
|
||||
* Add `application/vnd.3gpp.state-and-event-info+xml`
|
||||
* Add `application/vnd.anki`
|
||||
* Add `application/vnd.firemonkeys.cloudcell`
|
||||
* Add `application/vnd.openblox.game+xml`
|
||||
* Add `application/vnd.openblox.game-binary`
|
||||
|
||||
1.17.0 / 2015-08-13
|
||||
===================
|
||||
|
||||
* Add `application/x-msdos-program`
|
||||
* Add `audio/g711-0`
|
||||
* Add `image/vnd.mozilla.apng`
|
||||
* Add extension `.exe` to `application/x-msdos-program`
|
||||
|
||||
1.16.0 / 2015-07-29
|
||||
===================
|
||||
|
||||
* Add `application/vnd.uri-map`
|
||||
|
||||
1.15.0 / 2015-07-13
|
||||
===================
|
||||
|
||||
* Add `application/x-httpd-php`
|
||||
|
||||
1.14.0 / 2015-06-25
|
||||
===================
|
||||
|
||||
* Add `application/scim+json`
|
||||
* Add `application/vnd.3gpp.ussd+xml`
|
||||
* Add `application/vnd.biopax.rdf+xml`
|
||||
* Add `text/x-processing`
|
||||
|
||||
1.13.0 / 2015-06-07
|
||||
===================
|
||||
|
||||
* Add nginx as a source
|
||||
* Add `application/x-cocoa`
|
||||
* Add `application/x-java-archive-diff`
|
||||
* Add `application/x-makeself`
|
||||
* Add `application/x-perl`
|
||||
* Add `application/x-pilot`
|
||||
* Add `application/x-redhat-package-manager`
|
||||
* Add `application/x-sea`
|
||||
* Add `audio/x-m4a`
|
||||
* Add `audio/x-realaudio`
|
||||
* Add `image/x-jng`
|
||||
* Add `text/mathml`
|
||||
|
||||
1.12.0 / 2015-06-05
|
||||
===================
|
||||
|
||||
* Add `application/bdoc`
|
||||
* Add `application/vnd.hyperdrive+json`
|
||||
* Add `application/x-bdoc`
|
||||
* Add extension `.rtf` to `text/rtf`
|
||||
|
||||
1.11.0 / 2015-05-31
|
||||
===================
|
||||
|
||||
* Add `audio/wav`
|
||||
* Add `audio/wave`
|
||||
* Add extension `.litcoffee` to `text/coffeescript`
|
||||
* Add extension `.sfd-hdstx` to `application/vnd.hydrostatix.sof-data`
|
||||
* Add extension `.n-gage` to `application/vnd.nokia.n-gage.symbian.install`
|
||||
|
||||
1.10.0 / 2015-05-19
|
||||
===================
|
||||
|
||||
* Add `application/vnd.balsamiq.bmpr`
|
||||
* Add `application/vnd.microsoft.portable-executable`
|
||||
* Add `application/x-ns-proxy-autoconfig`
|
||||
|
||||
1.9.1 / 2015-04-19
|
||||
==================
|
||||
|
||||
* Remove `.json` extension from `application/manifest+json`
|
||||
- This is causing bugs downstream
|
||||
|
||||
1.9.0 / 2015-04-19
|
||||
==================
|
||||
|
||||
* Add `application/manifest+json`
|
||||
* Add `application/vnd.micro+json`
|
||||
* Add `image/vnd.zbrush.pcx`
|
||||
* Add `image/x-ms-bmp`
|
||||
|
||||
1.8.0 / 2015-03-13
|
||||
==================
|
||||
|
||||
* Add `application/vnd.citationstyles.style+xml`
|
||||
* Add `application/vnd.fastcopy-disk-image`
|
||||
* Add `application/vnd.gov.sk.xmldatacontainer+xml`
|
||||
* Add extension `.jsonld` to `application/ld+json`
|
||||
|
||||
1.7.0 / 2015-02-08
|
||||
==================
|
||||
|
||||
* Add `application/vnd.gerber`
|
||||
* Add `application/vnd.msa-disk-image`
|
||||
|
||||
1.6.1 / 2015-02-05
|
||||
==================
|
||||
|
||||
* Community extensions ownership transferred from `node-mime`
|
||||
|
||||
1.6.0 / 2015-01-29
|
||||
==================
|
||||
|
||||
* Add `application/jose`
|
||||
* Add `application/jose+json`
|
||||
* Add `application/json-seq`
|
||||
* Add `application/jwk+json`
|
||||
* Add `application/jwk-set+json`
|
||||
* Add `application/jwt`
|
||||
* Add `application/rdap+json`
|
||||
* Add `application/vnd.gov.sk.e-form+xml`
|
||||
* Add `application/vnd.ims.imsccv1p3`
|
||||
|
||||
1.5.0 / 2014-12-30
|
||||
==================
|
||||
|
||||
* Add `application/vnd.oracle.resource+json`
|
||||
* Fix various invalid MIME type entries
|
||||
- `application/mbox+xml`
|
||||
- `application/oscp-response`
|
||||
- `application/vwg-multiplexed`
|
||||
- `audio/g721`
|
||||
|
||||
1.4.0 / 2014-12-21
|
||||
==================
|
||||
|
||||
* Add `application/vnd.ims.imsccv1p2`
|
||||
* Fix various invalid MIME type entries
|
||||
- `application/vnd-acucobol`
|
||||
- `application/vnd-curl`
|
||||
- `application/vnd-dart`
|
||||
- `application/vnd-dxr`
|
||||
- `application/vnd-fdf`
|
||||
- `application/vnd-mif`
|
||||
- `application/vnd-sema`
|
||||
- `application/vnd-wap-wmlc`
|
||||
- `application/vnd.adobe.flash-movie`
|
||||
- `application/vnd.dece-zip`
|
||||
- `application/vnd.dvb_service`
|
||||
- `application/vnd.micrografx-igx`
|
||||
- `application/vnd.sealed-doc`
|
||||
- `application/vnd.sealed-eml`
|
||||
- `application/vnd.sealed-mht`
|
||||
- `application/vnd.sealed-ppt`
|
||||
- `application/vnd.sealed-tiff`
|
||||
- `application/vnd.sealed-xls`
|
||||
- `application/vnd.sealedmedia.softseal-html`
|
||||
- `application/vnd.sealedmedia.softseal-pdf`
|
||||
- `application/vnd.wap-slc`
|
||||
- `application/vnd.wap-wbxml`
|
||||
- `audio/vnd.sealedmedia.softseal-mpeg`
|
||||
- `image/vnd-djvu`
|
||||
- `image/vnd-svf`
|
||||
- `image/vnd-wap-wbmp`
|
||||
- `image/vnd.sealed-png`
|
||||
- `image/vnd.sealedmedia.softseal-gif`
|
||||
- `image/vnd.sealedmedia.softseal-jpg`
|
||||
- `model/vnd-dwf`
|
||||
- `model/vnd.parasolid.transmit-binary`
|
||||
- `model/vnd.parasolid.transmit-text`
|
||||
- `text/vnd-a`
|
||||
- `text/vnd-curl`
|
||||
- `text/vnd.wap-wml`
|
||||
* Remove example template MIME types
|
||||
- `application/example`
|
||||
- `audio/example`
|
||||
- `image/example`
|
||||
- `message/example`
|
||||
- `model/example`
|
||||
- `multipart/example`
|
||||
- `text/example`
|
||||
- `video/example`
|
||||
|
||||
1.3.1 / 2014-12-16
|
||||
==================
|
||||
|
||||
* Fix missing extensions
|
||||
- `application/json5`
|
||||
- `text/hjson`
|
||||
|
||||
1.3.0 / 2014-12-07
|
||||
==================
|
||||
|
||||
* Add `application/a2l`
|
||||
* Add `application/aml`
|
||||
* Add `application/atfx`
|
||||
* Add `application/atxml`
|
||||
* Add `application/cdfx+xml`
|
||||
* Add `application/dii`
|
||||
* Add `application/json5`
|
||||
* Add `application/lxf`
|
||||
* Add `application/mf4`
|
||||
* Add `application/vnd.apache.thrift.compact`
|
||||
* Add `application/vnd.apache.thrift.json`
|
||||
* Add `application/vnd.coffeescript`
|
||||
* Add `application/vnd.enphase.envoy`
|
||||
* Add `application/vnd.ims.imsccv1p1`
|
||||
* Add `text/csv-schema`
|
||||
* Add `text/hjson`
|
||||
* Add `text/markdown`
|
||||
* Add `text/yaml`
|
||||
|
||||
1.2.0 / 2014-11-09
|
||||
==================
|
||||
|
||||
* Add `application/cea`
|
||||
* Add `application/dit`
|
||||
* Add `application/vnd.gov.sk.e-form+zip`
|
||||
* Add `application/vnd.tmd.mediaflex.api+xml`
|
||||
* Type `application/epub+zip` is now IANA-registered
|
||||
|
||||
1.1.2 / 2014-10-23
|
||||
==================
|
||||
|
||||
* Rebuild database for `application/x-www-form-urlencoded` change
|
||||
|
||||
1.1.1 / 2014-10-20
|
||||
==================
|
||||
|
||||
* Mark `application/x-www-form-urlencoded` as compressible.
|
||||
|
||||
1.1.0 / 2014-09-28
|
||||
==================
|
||||
|
||||
* Add `application/font-woff2`
|
||||
|
||||
1.0.3 / 2014-09-25
|
||||
==================
|
||||
|
||||
* Fix engine requirement in package
|
||||
|
||||
1.0.2 / 2014-09-25
|
||||
==================
|
||||
|
||||
* Add `application/coap-group+json`
|
||||
* Add `application/dcd`
|
||||
* Add `application/vnd.apache.thrift.binary`
|
||||
* Add `image/vnd.tencent.tap`
|
||||
* Mark all JSON-derived types as compressible
|
||||
* Update `text/vtt` data
|
||||
|
||||
1.0.1 / 2014-08-30
|
||||
==================
|
||||
|
||||
* Fix extension ordering
|
||||
|
||||
1.0.0 / 2014-08-30
|
||||
==================
|
||||
|
||||
* Add `application/atf`
|
||||
* Add `application/merge-patch+json`
|
||||
* Add `multipart/x-mixed-replace`
|
||||
* Add `source: 'apache'` metadata
|
||||
* Add `source: 'iana'` metadata
|
||||
* Remove badly-assumed charset data
|
22
node_modules/express/node_modules/accepts/node_modules/mime-types/node_modules/mime-db/LICENSE
generated
vendored
Normal file
22
node_modules/express/node_modules/accepts/node_modules/mime-types/node_modules/mime-db/LICENSE
generated
vendored
Normal file
@ -0,0 +1,22 @@
|
||||
|
||||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) 2014 Jonathan Ong me@jongleberry.com
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
82
node_modules/express/node_modules/accepts/node_modules/mime-types/node_modules/mime-db/README.md
generated
vendored
Normal file
82
node_modules/express/node_modules/accepts/node_modules/mime-types/node_modules/mime-db/README.md
generated
vendored
Normal file
@ -0,0 +1,82 @@
|
||||
# mime-db
|
||||
|
||||
[![NPM Version][npm-version-image]][npm-url]
|
||||
[![NPM Downloads][npm-downloads-image]][npm-url]
|
||||
[![Node.js Version][node-image]][node-url]
|
||||
[![Build Status][travis-image]][travis-url]
|
||||
[![Coverage Status][coveralls-image]][coveralls-url]
|
||||
|
||||
This is a database of all mime types.
|
||||
It consists of a single, public JSON file and does not include any logic,
|
||||
allowing it to remain as un-opinionated as possible with an API.
|
||||
It aggregates data from the following sources:
|
||||
|
||||
- http://www.iana.org/assignments/media-types/media-types.xhtml
|
||||
- http://svn.apache.org/repos/asf/httpd/httpd/trunk/docs/conf/mime.types
|
||||
- http://hg.nginx.org/nginx/raw-file/default/conf/mime.types
|
||||
|
||||
## Installation
|
||||
|
||||
```bash
|
||||
npm install mime-db
|
||||
```
|
||||
|
||||
### Database Download
|
||||
|
||||
If you're crazy enough to use this in the browser, you can just grab the
|
||||
JSON file using [RawGit](https://rawgit.com/). It is recommended to replace
|
||||
`master` with [a release tag](https://github.com/jshttp/mime-db/tags) as the
|
||||
JSON format may change in the future.
|
||||
|
||||
```
|
||||
https://cdn.rawgit.com/jshttp/mime-db/master/db.json
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
```js
|
||||
var db = require('mime-db');
|
||||
|
||||
// grab data on .js files
|
||||
var data = db['application/javascript'];
|
||||
```
|
||||
|
||||
## Data Structure
|
||||
|
||||
The JSON file is a map lookup for lowercased mime types.
|
||||
Each mime type has the following properties:
|
||||
|
||||
- `.source` - where the mime type is defined.
|
||||
If not set, it's probably a custom media type.
|
||||
- `apache` - [Apache common media types](http://svn.apache.org/repos/asf/httpd/httpd/trunk/docs/conf/mime.types)
|
||||
- `iana` - [IANA-defined media types](http://www.iana.org/assignments/media-types/media-types.xhtml)
|
||||
- `nginx` - [nginx media types](http://hg.nginx.org/nginx/raw-file/default/conf/mime.types)
|
||||
- `.extensions[]` - known extensions associated with this mime type.
|
||||
- `.compressible` - whether a file of this type can be gzipped.
|
||||
- `.charset` - the default charset associated with this type, if any.
|
||||
|
||||
If unknown, every property could be `undefined`.
|
||||
|
||||
## Contributing
|
||||
|
||||
To edit the database, only make PRs against `src/custom.json` or
|
||||
`src/custom-suffix.json`.
|
||||
|
||||
To update the build, run `npm run build`.
|
||||
|
||||
## Adding Custom Media Types
|
||||
|
||||
The best way to get new media types included in this library is to register
|
||||
them with the IANA. The community registration procedure is outlined in
|
||||
[RFC 6838 section 5](http://tools.ietf.org/html/rfc6838#section-5). Types
|
||||
registered with the IANA are automatically pulled into this library.
|
||||
|
||||
[npm-version-image]: https://img.shields.io/npm/v/mime-db.svg
|
||||
[npm-downloads-image]: https://img.shields.io/npm/dm/mime-db.svg
|
||||
[npm-url]: https://npmjs.org/package/mime-db
|
||||
[travis-image]: https://img.shields.io/travis/jshttp/mime-db/master.svg
|
||||
[travis-url]: https://travis-ci.org/jshttp/mime-db
|
||||
[coveralls-image]: https://img.shields.io/coveralls/jshttp/mime-db/master.svg
|
||||
[coveralls-url]: https://coveralls.io/r/jshttp/mime-db?branch=master
|
||||
[node-image]: https://img.shields.io/node/v/mime-db.svg
|
||||
[node-url]: http://nodejs.org/download/
|
6552
node_modules/express/node_modules/accepts/node_modules/mime-types/node_modules/mime-db/db.json
generated
vendored
Normal file
6552
node_modules/express/node_modules/accepts/node_modules/mime-types/node_modules/mime-db/db.json
generated
vendored
Normal file
File diff suppressed because it is too large
Load Diff
11
node_modules/express/node_modules/accepts/node_modules/mime-types/node_modules/mime-db/index.js
generated
vendored
Normal file
11
node_modules/express/node_modules/accepts/node_modules/mime-types/node_modules/mime-db/index.js
generated
vendored
Normal file
@ -0,0 +1,11 @@
|
||||
/*!
|
||||
* mime-db
|
||||
* Copyright(c) 2014 Jonathan Ong
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
/**
|
||||
* Module exports.
|
||||
*/
|
||||
|
||||
module.exports = require('./db.json')
|
93
node_modules/express/node_modules/accepts/node_modules/mime-types/node_modules/mime-db/package.json
generated
vendored
Normal file
93
node_modules/express/node_modules/accepts/node_modules/mime-types/node_modules/mime-db/package.json
generated
vendored
Normal file
@ -0,0 +1,93 @@
|
||||
{
|
||||
"name": "mime-db",
|
||||
"description": "Media Type Database",
|
||||
"version": "1.21.0",
|
||||
"contributors": [
|
||||
{
|
||||
"name": "Douglas Christopher Wilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
{
|
||||
"name": "Jonathan Ong",
|
||||
"email": "me@jongleberry.com",
|
||||
"url": "http://jongleberry.com"
|
||||
},
|
||||
{
|
||||
"name": "Robert Kieffer",
|
||||
"email": "robert@broofa.com",
|
||||
"url": "http://github.com/broofa"
|
||||
}
|
||||
],
|
||||
"license": "MIT",
|
||||
"keywords": [
|
||||
"mime",
|
||||
"db",
|
||||
"type",
|
||||
"types",
|
||||
"database",
|
||||
"charset",
|
||||
"charsets"
|
||||
],
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "https://github.com/jshttp/mime-db"
|
||||
},
|
||||
"devDependencies": {
|
||||
"bluebird": "3.1.1",
|
||||
"co": "4.6.0",
|
||||
"cogent": "1.0.1",
|
||||
"csv-parse": "1.0.1",
|
||||
"gnode": "0.1.1",
|
||||
"istanbul": "0.4.1",
|
||||
"mocha": "1.21.5",
|
||||
"raw-body": "2.1.5",
|
||||
"stream-to-array": "2.2.0"
|
||||
},
|
||||
"files": [
|
||||
"HISTORY.md",
|
||||
"LICENSE",
|
||||
"README.md",
|
||||
"db.json",
|
||||
"index.js"
|
||||
],
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
},
|
||||
"scripts": {
|
||||
"build": "node scripts/build",
|
||||
"fetch": "gnode scripts/fetch-apache && gnode scripts/fetch-iana && gnode scripts/fetch-nginx",
|
||||
"test": "mocha --reporter spec --bail --check-leaks test/",
|
||||
"test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot --check-leaks test/",
|
||||
"test-travis": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --check-leaks test/",
|
||||
"update": "npm run fetch && npm run build"
|
||||
},
|
||||
"gitHead": "9ab92f0a912a602408a64db5741dfef6f82c597f",
|
||||
"bugs": {
|
||||
"url": "https://github.com/jshttp/mime-db/issues"
|
||||
},
|
||||
"homepage": "https://github.com/jshttp/mime-db",
|
||||
"_id": "mime-db@1.21.0",
|
||||
"_shasum": "9b5239e3353cf6eb015a00d890261027c36d4bac",
|
||||
"_from": "mime-db@~1.21.0",
|
||||
"_npmVersion": "1.4.28",
|
||||
"_npmUser": {
|
||||
"name": "dougwilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
"maintainers": [
|
||||
{
|
||||
"name": "jongleberry",
|
||||
"email": "jonathanrichardong@gmail.com"
|
||||
},
|
||||
{
|
||||
"name": "dougwilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
}
|
||||
],
|
||||
"dist": {
|
||||
"shasum": "9b5239e3353cf6eb015a00d890261027c36d4bac",
|
||||
"tarball": "http://registry.npmjs.org/mime-db/-/mime-db-1.21.0.tgz"
|
||||
},
|
||||
"directories": {},
|
||||
"_resolved": "https://registry.npmjs.org/mime-db/-/mime-db-1.21.0.tgz"
|
||||
}
|
83
node_modules/express/node_modules/accepts/node_modules/mime-types/package.json
generated
vendored
Normal file
83
node_modules/express/node_modules/accepts/node_modules/mime-types/package.json
generated
vendored
Normal file
@ -0,0 +1,83 @@
|
||||
{
|
||||
"name": "mime-types",
|
||||
"description": "The ultimate javascript content-type utility.",
|
||||
"version": "2.1.9",
|
||||
"contributors": [
|
||||
{
|
||||
"name": "Douglas Christopher Wilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
{
|
||||
"name": "Jeremiah Senkpiel",
|
||||
"email": "fishrock123@rocketmail.com",
|
||||
"url": "https://searchbeam.jit.su"
|
||||
},
|
||||
{
|
||||
"name": "Jonathan Ong",
|
||||
"email": "me@jongleberry.com",
|
||||
"url": "http://jongleberry.com"
|
||||
}
|
||||
],
|
||||
"license": "MIT",
|
||||
"keywords": [
|
||||
"mime",
|
||||
"types"
|
||||
],
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "https://github.com/jshttp/mime-types"
|
||||
},
|
||||
"dependencies": {
|
||||
"mime-db": "~1.21.0"
|
||||
},
|
||||
"devDependencies": {
|
||||
"istanbul": "0.4.1",
|
||||
"mocha": "~1.21.5"
|
||||
},
|
||||
"files": [
|
||||
"HISTORY.md",
|
||||
"LICENSE",
|
||||
"index.js"
|
||||
],
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "mocha --reporter spec test/test.js",
|
||||
"test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot test/test.js",
|
||||
"test-travis": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter dot test/test.js"
|
||||
},
|
||||
"gitHead": "329f1c77e1a77c8fac59b15038e3808e9e314d96",
|
||||
"bugs": {
|
||||
"url": "https://github.com/jshttp/mime-types/issues"
|
||||
},
|
||||
"homepage": "https://github.com/jshttp/mime-types",
|
||||
"_id": "mime-types@2.1.9",
|
||||
"_shasum": "dfb396764b5fdf75be34b1f4104bc3687fb635f8",
|
||||
"_from": "mime-types@~2.1.6",
|
||||
"_npmVersion": "1.4.28",
|
||||
"_npmUser": {
|
||||
"name": "dougwilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
"maintainers": [
|
||||
{
|
||||
"name": "jongleberry",
|
||||
"email": "jonathanrichardong@gmail.com"
|
||||
},
|
||||
{
|
||||
"name": "fishrock123",
|
||||
"email": "fishrock123@rocketmail.com"
|
||||
},
|
||||
{
|
||||
"name": "dougwilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
}
|
||||
],
|
||||
"dist": {
|
||||
"shasum": "dfb396764b5fdf75be34b1f4104bc3687fb635f8",
|
||||
"tarball": "http://registry.npmjs.org/mime-types/-/mime-types-2.1.9.tgz"
|
||||
},
|
||||
"directories": {},
|
||||
"_resolved": "https://registry.npmjs.org/mime-types/-/mime-types-2.1.9.tgz"
|
||||
}
|
76
node_modules/express/node_modules/accepts/node_modules/negotiator/HISTORY.md
generated
vendored
Normal file
76
node_modules/express/node_modules/accepts/node_modules/negotiator/HISTORY.md
generated
vendored
Normal file
@ -0,0 +1,76 @@
|
||||
0.5.3 / 2015-05-10
|
||||
==================
|
||||
|
||||
* Fix media type parameter matching to be case-insensitive
|
||||
|
||||
0.5.2 / 2015-05-06
|
||||
==================
|
||||
|
||||
* Fix comparing media types with quoted values
|
||||
* Fix splitting media types with quoted commas
|
||||
|
||||
0.5.1 / 2015-02-14
|
||||
==================
|
||||
|
||||
* Fix preference sorting to be stable for long acceptable lists
|
||||
|
||||
0.5.0 / 2014-12-18
|
||||
==================
|
||||
|
||||
* Fix list return order when large accepted list
|
||||
* Fix missing identity encoding when q=0 exists
|
||||
* Remove dynamic building of Negotiator class
|
||||
|
||||
0.4.9 / 2014-10-14
|
||||
==================
|
||||
|
||||
* Fix error when media type has invalid parameter
|
||||
|
||||
0.4.8 / 2014-09-28
|
||||
==================
|
||||
|
||||
* Fix all negotiations to be case-insensitive
|
||||
* Stable sort preferences of same quality according to client order
|
||||
* Support Node.js 0.6
|
||||
|
||||
0.4.7 / 2014-06-24
|
||||
==================
|
||||
|
||||
* Handle invalid provided languages
|
||||
* Handle invalid provided media types
|
||||
|
||||
0.4.6 / 2014-06-11
|
||||
==================
|
||||
|
||||
* Order by specificity when quality is the same
|
||||
|
||||
0.4.5 / 2014-05-29
|
||||
==================
|
||||
|
||||
* Fix regression in empty header handling
|
||||
|
||||
0.4.4 / 2014-05-29
|
||||
==================
|
||||
|
||||
* Fix behaviors when headers are not present
|
||||
|
||||
0.4.3 / 2014-04-16
|
||||
==================
|
||||
|
||||
* Handle slashes on media params correctly
|
||||
|
||||
0.4.2 / 2014-02-28
|
||||
==================
|
||||
|
||||
* Fix media type sorting
|
||||
* Handle media types params strictly
|
||||
|
||||
0.4.1 / 2014-01-16
|
||||
==================
|
||||
|
||||
* Use most specific matches
|
||||
|
||||
0.4.0 / 2014-01-09
|
||||
==================
|
||||
|
||||
* Remove preferred prefix from methods
|
24
node_modules/express/node_modules/accepts/node_modules/negotiator/LICENSE
generated
vendored
Normal file
24
node_modules/express/node_modules/accepts/node_modules/negotiator/LICENSE
generated
vendored
Normal file
@ -0,0 +1,24 @@
|
||||
(The MIT License)
|
||||
|
||||
Copyright (c) 2012-2014 Federico Romero
|
||||
Copyright (c) 2012-2014 Isaac Z. Schlueter
|
||||
Copyright (c) 2014-2015 Douglas Christopher Wilson
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of this software and associated documentation files (the
|
||||
'Software'), to deal in the Software without restriction, including
|
||||
without limitation the rights to use, copy, modify, merge, publish,
|
||||
distribute, sublicense, and/or sell copies of the Software, and to
|
||||
permit persons to whom the Software is furnished to do so, subject to
|
||||
the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
|
||||
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
|
||||
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
|
||||
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
|
||||
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
203
node_modules/express/node_modules/accepts/node_modules/negotiator/README.md
generated
vendored
Normal file
203
node_modules/express/node_modules/accepts/node_modules/negotiator/README.md
generated
vendored
Normal file
@ -0,0 +1,203 @@
|
||||
# negotiator
|
||||
|
||||
[![NPM Version][npm-image]][npm-url]
|
||||
[![NPM Downloads][downloads-image]][downloads-url]
|
||||
[![Node.js Version][node-version-image]][node-version-url]
|
||||
[![Build Status][travis-image]][travis-url]
|
||||
[![Test Coverage][coveralls-image]][coveralls-url]
|
||||
|
||||
An HTTP content negotiator for Node.js
|
||||
|
||||
## Installation
|
||||
|
||||
```sh
|
||||
$ npm install negotiator
|
||||
```
|
||||
|
||||
## API
|
||||
|
||||
```js
|
||||
var Negotiator = require('negotiator')
|
||||
```
|
||||
|
||||
### Accept Negotiation
|
||||
|
||||
```js
|
||||
availableMediaTypes = ['text/html', 'text/plain', 'application/json']
|
||||
|
||||
// The negotiator constructor receives a request object
|
||||
negotiator = new Negotiator(request)
|
||||
|
||||
// Let's say Accept header is 'text/html, application/*;q=0.2, image/jpeg;q=0.8'
|
||||
|
||||
negotiator.mediaTypes()
|
||||
// -> ['text/html', 'image/jpeg', 'application/*']
|
||||
|
||||
negotiator.mediaTypes(availableMediaTypes)
|
||||
// -> ['text/html', 'application/json']
|
||||
|
||||
negotiator.mediaType(availableMediaTypes)
|
||||
// -> 'text/html'
|
||||
```
|
||||
|
||||
You can check a working example at `examples/accept.js`.
|
||||
|
||||
#### Methods
|
||||
|
||||
##### mediaType()
|
||||
|
||||
Returns the most preferred media type from the client.
|
||||
|
||||
##### mediaType(availableMediaType)
|
||||
|
||||
Returns the most preferred media type from a list of available media types.
|
||||
|
||||
##### mediaTypes()
|
||||
|
||||
Returns an array of preferred media types ordered by the client preference.
|
||||
|
||||
##### mediaTypes(availableMediaTypes)
|
||||
|
||||
Returns an array of preferred media types ordered by priority from a list of
|
||||
available media types.
|
||||
|
||||
### Accept-Language Negotiation
|
||||
|
||||
```js
|
||||
negotiator = new Negotiator(request)
|
||||
|
||||
availableLanguages = 'en', 'es', 'fr'
|
||||
|
||||
// Let's say Accept-Language header is 'en;q=0.8, es, pt'
|
||||
|
||||
negotiator.languages()
|
||||
// -> ['es', 'pt', 'en']
|
||||
|
||||
negotiator.languages(availableLanguages)
|
||||
// -> ['es', 'en']
|
||||
|
||||
language = negotiator.language(availableLanguages)
|
||||
// -> 'es'
|
||||
```
|
||||
|
||||
You can check a working example at `examples/language.js`.
|
||||
|
||||
#### Methods
|
||||
|
||||
##### language()
|
||||
|
||||
Returns the most preferred language from the client.
|
||||
|
||||
##### language(availableLanguages)
|
||||
|
||||
Returns the most preferred language from a list of available languages.
|
||||
|
||||
##### languages()
|
||||
|
||||
Returns an array of preferred languages ordered by the client preference.
|
||||
|
||||
##### languages(availableLanguages)
|
||||
|
||||
Returns an array of preferred languages ordered by priority from a list of
|
||||
available languages.
|
||||
|
||||
### Accept-Charset Negotiation
|
||||
|
||||
```js
|
||||
availableCharsets = ['utf-8', 'iso-8859-1', 'iso-8859-5']
|
||||
|
||||
negotiator = new Negotiator(request)
|
||||
|
||||
// Let's say Accept-Charset header is 'utf-8, iso-8859-1;q=0.8, utf-7;q=0.2'
|
||||
|
||||
negotiator.charsets()
|
||||
// -> ['utf-8', 'iso-8859-1', 'utf-7']
|
||||
|
||||
negotiator.charsets(availableCharsets)
|
||||
// -> ['utf-8', 'iso-8859-1']
|
||||
|
||||
negotiator.charset(availableCharsets)
|
||||
// -> 'utf-8'
|
||||
```
|
||||
|
||||
You can check a working example at `examples/charset.js`.
|
||||
|
||||
#### Methods
|
||||
|
||||
##### charset()
|
||||
|
||||
Returns the most preferred charset from the client.
|
||||
|
||||
##### charset(availableCharsets)
|
||||
|
||||
Returns the most preferred charset from a list of available charsets.
|
||||
|
||||
##### charsets()
|
||||
|
||||
Returns an array of preferred charsets ordered by the client preference.
|
||||
|
||||
##### charsets(availableCharsets)
|
||||
|
||||
Returns an array of preferred charsets ordered by priority from a list of
|
||||
available charsets.
|
||||
|
||||
### Accept-Encoding Negotiation
|
||||
|
||||
```js
|
||||
availableEncodings = ['identity', 'gzip']
|
||||
|
||||
negotiator = new Negotiator(request)
|
||||
|
||||
// Let's say Accept-Encoding header is 'gzip, compress;q=0.2, identity;q=0.5'
|
||||
|
||||
negotiator.encodings()
|
||||
// -> ['gzip', 'identity', 'compress']
|
||||
|
||||
negotiator.encodings(availableEncodings)
|
||||
// -> ['gzip', 'identity']
|
||||
|
||||
negotiator.encoding(availableEncodings)
|
||||
// -> 'gzip'
|
||||
```
|
||||
|
||||
You can check a working example at `examples/encoding.js`.
|
||||
|
||||
#### Methods
|
||||
|
||||
##### encoding()
|
||||
|
||||
Returns the most preferred encoding from the client.
|
||||
|
||||
##### encoding(availableEncodings)
|
||||
|
||||
Returns the most preferred encoding from a list of available encodings.
|
||||
|
||||
##### encodings()
|
||||
|
||||
Returns an array of preferred encodings ordered by the client preference.
|
||||
|
||||
##### encodings(availableEncodings)
|
||||
|
||||
Returns an array of preferred encodings ordered by priority from a list of
|
||||
available encodings.
|
||||
|
||||
## See Also
|
||||
|
||||
The [accepts](https://npmjs.org/package/accepts#readme) module builds on
|
||||
this module and provides an alternative interface, mime type validation,
|
||||
and more.
|
||||
|
||||
## License
|
||||
|
||||
[MIT](LICENSE)
|
||||
|
||||
[npm-image]: https://img.shields.io/npm/v/negotiator.svg
|
||||
[npm-url]: https://npmjs.org/package/negotiator
|
||||
[node-version-image]: https://img.shields.io/node/v/negotiator.svg
|
||||
[node-version-url]: http://nodejs.org/download/
|
||||
[travis-image]: https://img.shields.io/travis/jshttp/negotiator/master.svg
|
||||
[travis-url]: https://travis-ci.org/jshttp/negotiator
|
||||
[coveralls-image]: https://img.shields.io/coveralls/jshttp/negotiator/master.svg
|
||||
[coveralls-url]: https://coveralls.io/r/jshttp/negotiator?branch=master
|
||||
[downloads-image]: https://img.shields.io/npm/dm/negotiator.svg
|
||||
[downloads-url]: https://npmjs.org/package/negotiator
|
62
node_modules/express/node_modules/accepts/node_modules/negotiator/index.js
generated
vendored
Normal file
62
node_modules/express/node_modules/accepts/node_modules/negotiator/index.js
generated
vendored
Normal file
@ -0,0 +1,62 @@
|
||||
|
||||
var preferredCharsets = require('./lib/charset');
|
||||
var preferredEncodings = require('./lib/encoding');
|
||||
var preferredLanguages = require('./lib/language');
|
||||
var preferredMediaTypes = require('./lib/mediaType');
|
||||
|
||||
module.exports = Negotiator;
|
||||
Negotiator.Negotiator = Negotiator;
|
||||
|
||||
function Negotiator(request) {
|
||||
if (!(this instanceof Negotiator)) {
|
||||
return new Negotiator(request);
|
||||
}
|
||||
|
||||
this.request = request;
|
||||
}
|
||||
|
||||
Negotiator.prototype.charset = function charset(available) {
|
||||
var set = this.charsets(available);
|
||||
return set && set[0];
|
||||
};
|
||||
|
||||
Negotiator.prototype.charsets = function charsets(available) {
|
||||
return preferredCharsets(this.request.headers['accept-charset'], available);
|
||||
};
|
||||
|
||||
Negotiator.prototype.encoding = function encoding(available) {
|
||||
var set = this.encodings(available);
|
||||
return set && set[0];
|
||||
};
|
||||
|
||||
Negotiator.prototype.encodings = function encodings(available) {
|
||||
return preferredEncodings(this.request.headers['accept-encoding'], available);
|
||||
};
|
||||
|
||||
Negotiator.prototype.language = function language(available) {
|
||||
var set = this.languages(available);
|
||||
return set && set[0];
|
||||
};
|
||||
|
||||
Negotiator.prototype.languages = function languages(available) {
|
||||
return preferredLanguages(this.request.headers['accept-language'], available);
|
||||
};
|
||||
|
||||
Negotiator.prototype.mediaType = function mediaType(available) {
|
||||
var set = this.mediaTypes(available);
|
||||
return set && set[0];
|
||||
};
|
||||
|
||||
Negotiator.prototype.mediaTypes = function mediaTypes(available) {
|
||||
return preferredMediaTypes(this.request.headers.accept, available);
|
||||
};
|
||||
|
||||
// Backwards compatibility
|
||||
Negotiator.prototype.preferredCharset = Negotiator.prototype.charset;
|
||||
Negotiator.prototype.preferredCharsets = Negotiator.prototype.charsets;
|
||||
Negotiator.prototype.preferredEncoding = Negotiator.prototype.encoding;
|
||||
Negotiator.prototype.preferredEncodings = Negotiator.prototype.encodings;
|
||||
Negotiator.prototype.preferredLanguage = Negotiator.prototype.language;
|
||||
Negotiator.prototype.preferredLanguages = Negotiator.prototype.languages;
|
||||
Negotiator.prototype.preferredMediaType = Negotiator.prototype.mediaType;
|
||||
Negotiator.prototype.preferredMediaTypes = Negotiator.prototype.mediaTypes;
|
102
node_modules/express/node_modules/accepts/node_modules/negotiator/lib/charset.js
generated
vendored
Normal file
102
node_modules/express/node_modules/accepts/node_modules/negotiator/lib/charset.js
generated
vendored
Normal file
@ -0,0 +1,102 @@
|
||||
module.exports = preferredCharsets;
|
||||
preferredCharsets.preferredCharsets = preferredCharsets;
|
||||
|
||||
function parseAcceptCharset(accept) {
|
||||
var accepts = accept.split(',');
|
||||
|
||||
for (var i = 0, j = 0; i < accepts.length; i++) {
|
||||
var charset = parseCharset(accepts[i].trim(), i);
|
||||
|
||||
if (charset) {
|
||||
accepts[j++] = charset;
|
||||
}
|
||||
}
|
||||
|
||||
// trim accepts
|
||||
accepts.length = j;
|
||||
|
||||
return accepts;
|
||||
}
|
||||
|
||||
function parseCharset(s, i) {
|
||||
var match = s.match(/^\s*(\S+?)\s*(?:;(.*))?$/);
|
||||
if (!match) return null;
|
||||
|
||||
var charset = match[1];
|
||||
var q = 1;
|
||||
if (match[2]) {
|
||||
var params = match[2].split(';')
|
||||
for (var i = 0; i < params.length; i ++) {
|
||||
var p = params[i].trim().split('=');
|
||||
if (p[0] === 'q') {
|
||||
q = parseFloat(p[1]);
|
||||
break;
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
return {
|
||||
charset: charset,
|
||||
q: q,
|
||||
i: i
|
||||
};
|
||||
}
|
||||
|
||||
function getCharsetPriority(charset, accepted, index) {
|
||||
var priority = {o: -1, q: 0, s: 0};
|
||||
|
||||
for (var i = 0; i < accepted.length; i++) {
|
||||
var spec = specify(charset, accepted[i], index);
|
||||
|
||||
if (spec && (priority.s - spec.s || priority.q - spec.q || priority.o - spec.o) < 0) {
|
||||
priority = spec;
|
||||
}
|
||||
}
|
||||
|
||||
return priority;
|
||||
}
|
||||
|
||||
function specify(charset, spec, index) {
|
||||
var s = 0;
|
||||
if(spec.charset.toLowerCase() === charset.toLowerCase()){
|
||||
s |= 1;
|
||||
} else if (spec.charset !== '*' ) {
|
||||
return null
|
||||
}
|
||||
|
||||
return {
|
||||
i: index,
|
||||
o: spec.i,
|
||||
q: spec.q,
|
||||
s: s
|
||||
}
|
||||
}
|
||||
|
||||
function preferredCharsets(accept, provided) {
|
||||
// RFC 2616 sec 14.2: no header = *
|
||||
var accepts = parseAcceptCharset(accept === undefined ? '*' : accept || '');
|
||||
|
||||
if (!provided) {
|
||||
// sorted list of all charsets
|
||||
return accepts.filter(isQuality).sort(compareSpecs).map(function getCharset(spec) {
|
||||
return spec.charset;
|
||||
});
|
||||
}
|
||||
|
||||
var priorities = provided.map(function getPriority(type, index) {
|
||||
return getCharsetPriority(type, accepts, index);
|
||||
});
|
||||
|
||||
// sorted list of accepted charsets
|
||||
return priorities.filter(isQuality).sort(compareSpecs).map(function getCharset(priority) {
|
||||
return provided[priorities.indexOf(priority)];
|
||||
});
|
||||
}
|
||||
|
||||
function compareSpecs(a, b) {
|
||||
return (b.q - a.q) || (b.s - a.s) || (a.o - b.o) || (a.i - b.i) || 0;
|
||||
}
|
||||
|
||||
function isQuality(spec) {
|
||||
return spec.q > 0;
|
||||
}
|
118
node_modules/express/node_modules/accepts/node_modules/negotiator/lib/encoding.js
generated
vendored
Normal file
118
node_modules/express/node_modules/accepts/node_modules/negotiator/lib/encoding.js
generated
vendored
Normal file
@ -0,0 +1,118 @@
|
||||
module.exports = preferredEncodings;
|
||||
preferredEncodings.preferredEncodings = preferredEncodings;
|
||||
|
||||
function parseAcceptEncoding(accept) {
|
||||
var accepts = accept.split(',');
|
||||
var hasIdentity = false;
|
||||
var minQuality = 1;
|
||||
|
||||
for (var i = 0, j = 0; i < accepts.length; i++) {
|
||||
var encoding = parseEncoding(accepts[i].trim(), i);
|
||||
|
||||
if (encoding) {
|
||||
accepts[j++] = encoding;
|
||||
hasIdentity = hasIdentity || specify('identity', encoding);
|
||||
minQuality = Math.min(minQuality, encoding.q || 1);
|
||||
}
|
||||
}
|
||||
|
||||
if (!hasIdentity) {
|
||||
/*
|
||||
* If identity doesn't explicitly appear in the accept-encoding header,
|
||||
* it's added to the list of acceptable encoding with the lowest q
|
||||
*/
|
||||
accepts[j++] = {
|
||||
encoding: 'identity',
|
||||
q: minQuality,
|
||||
i: i
|
||||
};
|
||||
}
|
||||
|
||||
// trim accepts
|
||||
accepts.length = j;
|
||||
|
||||
return accepts;
|
||||
}
|
||||
|
||||
function parseEncoding(s, i) {
|
||||
var match = s.match(/^\s*(\S+?)\s*(?:;(.*))?$/);
|
||||
|
||||
if (!match) return null;
|
||||
|
||||
var encoding = match[1];
|
||||
var q = 1;
|
||||
if (match[2]) {
|
||||
var params = match[2].split(';');
|
||||
for (var i = 0; i < params.length; i ++) {
|
||||
var p = params[i].trim().split('=');
|
||||
if (p[0] === 'q') {
|
||||
q = parseFloat(p[1]);
|
||||
break;
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
return {
|
||||
encoding: encoding,
|
||||
q: q,
|
||||
i: i
|
||||
};
|
||||
}
|
||||
|
||||
function getEncodingPriority(encoding, accepted, index) {
|
||||
var priority = {o: -1, q: 0, s: 0};
|
||||
|
||||
for (var i = 0; i < accepted.length; i++) {
|
||||
var spec = specify(encoding, accepted[i], index);
|
||||
|
||||
if (spec && (priority.s - spec.s || priority.q - spec.q || priority.o - spec.o) < 0) {
|
||||
priority = spec;
|
||||
}
|
||||
}
|
||||
|
||||
return priority;
|
||||
}
|
||||
|
||||
function specify(encoding, spec, index) {
|
||||
var s = 0;
|
||||
if(spec.encoding.toLowerCase() === encoding.toLowerCase()){
|
||||
s |= 1;
|
||||
} else if (spec.encoding !== '*' ) {
|
||||
return null
|
||||
}
|
||||
|
||||
return {
|
||||
i: index,
|
||||
o: spec.i,
|
||||
q: spec.q,
|
||||
s: s
|
||||
}
|
||||
};
|
||||
|
||||
function preferredEncodings(accept, provided) {
|
||||
var accepts = parseAcceptEncoding(accept || '');
|
||||
|
||||
if (!provided) {
|
||||
// sorted list of all encodings
|
||||
return accepts.filter(isQuality).sort(compareSpecs).map(function getEncoding(spec) {
|
||||
return spec.encoding;
|
||||
});
|
||||
}
|
||||
|
||||
var priorities = provided.map(function getPriority(type, index) {
|
||||
return getEncodingPriority(type, accepts, index);
|
||||
});
|
||||
|
||||
// sorted list of accepted encodings
|
||||
return priorities.filter(isQuality).sort(compareSpecs).map(function getEncoding(priority) {
|
||||
return provided[priorities.indexOf(priority)];
|
||||
});
|
||||
}
|
||||
|
||||
function compareSpecs(a, b) {
|
||||
return (b.q - a.q) || (b.s - a.s) || (a.o - b.o) || (a.i - b.i) || 0;
|
||||
}
|
||||
|
||||
function isQuality(spec) {
|
||||
return spec.q > 0;
|
||||
}
|
112
node_modules/express/node_modules/accepts/node_modules/negotiator/lib/language.js
generated
vendored
Normal file
112
node_modules/express/node_modules/accepts/node_modules/negotiator/lib/language.js
generated
vendored
Normal file
@ -0,0 +1,112 @@
|
||||
module.exports = preferredLanguages;
|
||||
preferredLanguages.preferredLanguages = preferredLanguages;
|
||||
|
||||
function parseAcceptLanguage(accept) {
|
||||
var accepts = accept.split(',');
|
||||
|
||||
for (var i = 0, j = 0; i < accepts.length; i++) {
|
||||
var langauge = parseLanguage(accepts[i].trim(), i);
|
||||
|
||||
if (langauge) {
|
||||
accepts[j++] = langauge;
|
||||
}
|
||||
}
|
||||
|
||||
// trim accepts
|
||||
accepts.length = j;
|
||||
|
||||
return accepts;
|
||||
}
|
||||
|
||||
function parseLanguage(s, i) {
|
||||
var match = s.match(/^\s*(\S+?)(?:-(\S+?))?\s*(?:;(.*))?$/);
|
||||
if (!match) return null;
|
||||
|
||||
var prefix = match[1],
|
||||
suffix = match[2],
|
||||
full = prefix;
|
||||
|
||||
if (suffix) full += "-" + suffix;
|
||||
|
||||
var q = 1;
|
||||
if (match[3]) {
|
||||
var params = match[3].split(';')
|
||||
for (var i = 0; i < params.length; i ++) {
|
||||
var p = params[i].split('=');
|
||||
if (p[0] === 'q') q = parseFloat(p[1]);
|
||||
}
|
||||
}
|
||||
|
||||
return {
|
||||
prefix: prefix,
|
||||
suffix: suffix,
|
||||
q: q,
|
||||
i: i,
|
||||
full: full
|
||||
};
|
||||
}
|
||||
|
||||
function getLanguagePriority(language, accepted, index) {
|
||||
var priority = {o: -1, q: 0, s: 0};
|
||||
|
||||
for (var i = 0; i < accepted.length; i++) {
|
||||
var spec = specify(language, accepted[i], index);
|
||||
|
||||
if (spec && (priority.s - spec.s || priority.q - spec.q || priority.o - spec.o) < 0) {
|
||||
priority = spec;
|
||||
}
|
||||
}
|
||||
|
||||
return priority;
|
||||
}
|
||||
|
||||
function specify(language, spec, index) {
|
||||
var p = parseLanguage(language)
|
||||
if (!p) return null;
|
||||
var s = 0;
|
||||
if(spec.full.toLowerCase() === p.full.toLowerCase()){
|
||||
s |= 4;
|
||||
} else if (spec.prefix.toLowerCase() === p.full.toLowerCase()) {
|
||||
s |= 2;
|
||||
} else if (spec.full.toLowerCase() === p.prefix.toLowerCase()) {
|
||||
s |= 1;
|
||||
} else if (spec.full !== '*' ) {
|
||||
return null
|
||||
}
|
||||
|
||||
return {
|
||||
i: index,
|
||||
o: spec.i,
|
||||
q: spec.q,
|
||||
s: s
|
||||
}
|
||||
};
|
||||
|
||||
function preferredLanguages(accept, provided) {
|
||||
// RFC 2616 sec 14.4: no header = *
|
||||
var accepts = parseAcceptLanguage(accept === undefined ? '*' : accept || '');
|
||||
|
||||
if (!provided) {
|
||||
// sorted list of all languages
|
||||
return accepts.filter(isQuality).sort(compareSpecs).map(function getLanguage(spec) {
|
||||
return spec.full;
|
||||
});
|
||||
}
|
||||
|
||||
var priorities = provided.map(function getPriority(type, index) {
|
||||
return getLanguagePriority(type, accepts, index);
|
||||
});
|
||||
|
||||
// sorted list of accepted languages
|
||||
return priorities.filter(isQuality).sort(compareSpecs).map(function getLanguage(priority) {
|
||||
return provided[priorities.indexOf(priority)];
|
||||
});
|
||||
}
|
||||
|
||||
function compareSpecs(a, b) {
|
||||
return (b.q - a.q) || (b.s - a.s) || (a.o - b.o) || (a.i - b.i) || 0;
|
||||
}
|
||||
|
||||
function isQuality(spec) {
|
||||
return spec.q > 0;
|
||||
}
|
179
node_modules/express/node_modules/accepts/node_modules/negotiator/lib/mediaType.js
generated
vendored
Normal file
179
node_modules/express/node_modules/accepts/node_modules/negotiator/lib/mediaType.js
generated
vendored
Normal file
@ -0,0 +1,179 @@
|
||||
/**
|
||||
* negotiator
|
||||
* Copyright(c) 2012 Isaac Z. Schlueter
|
||||
* Copyright(c) 2014 Federico Romero
|
||||
* Copyright(c) 2014-2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
module.exports = preferredMediaTypes;
|
||||
preferredMediaTypes.preferredMediaTypes = preferredMediaTypes;
|
||||
|
||||
function parseAccept(accept) {
|
||||
var accepts = splitMediaTypes(accept);
|
||||
|
||||
for (var i = 0, j = 0; i < accepts.length; i++) {
|
||||
var mediaType = parseMediaType(accepts[i].trim(), i);
|
||||
|
||||
if (mediaType) {
|
||||
accepts[j++] = mediaType;
|
||||
}
|
||||
}
|
||||
|
||||
// trim accepts
|
||||
accepts.length = j;
|
||||
|
||||
return accepts;
|
||||
};
|
||||
|
||||
function parseMediaType(s, i) {
|
||||
var match = s.match(/\s*(\S+?)\/([^;\s]+)\s*(?:;(.*))?/);
|
||||
if (!match) return null;
|
||||
|
||||
var type = match[1],
|
||||
subtype = match[2],
|
||||
full = "" + type + "/" + subtype,
|
||||
params = {},
|
||||
q = 1;
|
||||
|
||||
if (match[3]) {
|
||||
params = match[3].split(';').map(function(s) {
|
||||
return s.trim().split('=');
|
||||
}).reduce(function (set, p) {
|
||||
var name = p[0].toLowerCase();
|
||||
var value = p[1];
|
||||
|
||||
set[name] = value && value[0] === '"' && value[value.length - 1] === '"'
|
||||
? value.substr(1, value.length - 2)
|
||||
: value;
|
||||
|
||||
return set;
|
||||
}, params);
|
||||
|
||||
if (params.q != null) {
|
||||
q = parseFloat(params.q);
|
||||
delete params.q;
|
||||
}
|
||||
}
|
||||
|
||||
return {
|
||||
type: type,
|
||||
subtype: subtype,
|
||||
params: params,
|
||||
q: q,
|
||||
i: i,
|
||||
full: full
|
||||
};
|
||||
}
|
||||
|
||||
function getMediaTypePriority(type, accepted, index) {
|
||||
var priority = {o: -1, q: 0, s: 0};
|
||||
|
||||
for (var i = 0; i < accepted.length; i++) {
|
||||
var spec = specify(type, accepted[i], index);
|
||||
|
||||
if (spec && (priority.s - spec.s || priority.q - spec.q || priority.o - spec.o) < 0) {
|
||||
priority = spec;
|
||||
}
|
||||
}
|
||||
|
||||
return priority;
|
||||
}
|
||||
|
||||
function specify(type, spec, index) {
|
||||
var p = parseMediaType(type);
|
||||
var s = 0;
|
||||
|
||||
if (!p) {
|
||||
return null;
|
||||
}
|
||||
|
||||
if(spec.type.toLowerCase() == p.type.toLowerCase()) {
|
||||
s |= 4
|
||||
} else if(spec.type != '*') {
|
||||
return null;
|
||||
}
|
||||
|
||||
if(spec.subtype.toLowerCase() == p.subtype.toLowerCase()) {
|
||||
s |= 2
|
||||
} else if(spec.subtype != '*') {
|
||||
return null;
|
||||
}
|
||||
|
||||
var keys = Object.keys(spec.params);
|
||||
if (keys.length > 0) {
|
||||
if (keys.every(function (k) {
|
||||
return spec.params[k] == '*' || (spec.params[k] || '').toLowerCase() == (p.params[k] || '').toLowerCase();
|
||||
})) {
|
||||
s |= 1
|
||||
} else {
|
||||
return null
|
||||
}
|
||||
}
|
||||
|
||||
return {
|
||||
i: index,
|
||||
o: spec.i,
|
||||
q: spec.q,
|
||||
s: s,
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
function preferredMediaTypes(accept, provided) {
|
||||
// RFC 2616 sec 14.2: no header = */*
|
||||
var accepts = parseAccept(accept === undefined ? '*/*' : accept || '');
|
||||
|
||||
if (!provided) {
|
||||
// sorted list of all types
|
||||
return accepts.filter(isQuality).sort(compareSpecs).map(function getType(spec) {
|
||||
return spec.full;
|
||||
});
|
||||
}
|
||||
|
||||
var priorities = provided.map(function getPriority(type, index) {
|
||||
return getMediaTypePriority(type, accepts, index);
|
||||
});
|
||||
|
||||
// sorted list of accepted types
|
||||
return priorities.filter(isQuality).sort(compareSpecs).map(function getType(priority) {
|
||||
return provided[priorities.indexOf(priority)];
|
||||
});
|
||||
}
|
||||
|
||||
function compareSpecs(a, b) {
|
||||
return (b.q - a.q) || (b.s - a.s) || (a.o - b.o) || (a.i - b.i) || 0;
|
||||
}
|
||||
|
||||
function isQuality(spec) {
|
||||
return spec.q > 0;
|
||||
}
|
||||
|
||||
function quoteCount(string) {
|
||||
var count = 0;
|
||||
var index = 0;
|
||||
|
||||
while ((index = string.indexOf('"', index)) !== -1) {
|
||||
count++;
|
||||
index++;
|
||||
}
|
||||
|
||||
return count;
|
||||
}
|
||||
|
||||
function splitMediaTypes(accept) {
|
||||
var accepts = accept.split(',');
|
||||
|
||||
for (var i = 1, j = 0; i < accepts.length; i++) {
|
||||
if (quoteCount(accepts[j]) % 2 == 0) {
|
||||
accepts[++j] = accepts[i];
|
||||
} else {
|
||||
accepts[j] += ',' + accepts[i];
|
||||
}
|
||||
}
|
||||
|
||||
// trim accepts
|
||||
accepts.length = j + 1;
|
||||
|
||||
return accepts;
|
||||
}
|
85
node_modules/express/node_modules/accepts/node_modules/negotiator/package.json
generated
vendored
Normal file
85
node_modules/express/node_modules/accepts/node_modules/negotiator/package.json
generated
vendored
Normal file
@ -0,0 +1,85 @@
|
||||
{
|
||||
"name": "negotiator",
|
||||
"description": "HTTP content negotiation",
|
||||
"version": "0.5.3",
|
||||
"contributors": [
|
||||
{
|
||||
"name": "Douglas Christopher Wilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
{
|
||||
"name": "Federico Romero",
|
||||
"email": "federico.romero@outboxlabs.com"
|
||||
},
|
||||
{
|
||||
"name": "Isaac Z. Schlueter",
|
||||
"email": "i@izs.me",
|
||||
"url": "http://blog.izs.me/"
|
||||
}
|
||||
],
|
||||
"license": "MIT",
|
||||
"keywords": [
|
||||
"http",
|
||||
"content negotiation",
|
||||
"accept",
|
||||
"accept-language",
|
||||
"accept-encoding",
|
||||
"accept-charset"
|
||||
],
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "https://github.com/jshttp/negotiator"
|
||||
},
|
||||
"devDependencies": {
|
||||
"istanbul": "0.3.9",
|
||||
"mocha": "~1.21.5"
|
||||
},
|
||||
"files": [
|
||||
"lib/",
|
||||
"HISTORY.md",
|
||||
"LICENSE",
|
||||
"index.js",
|
||||
"README.md"
|
||||
],
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "mocha --reporter spec --check-leaks --bail test/",
|
||||
"test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot --check-leaks test/",
|
||||
"test-travis": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --check-leaks test/"
|
||||
},
|
||||
"gitHead": "cbb717b3f164f25820f90b160cda6d0166b9d922",
|
||||
"bugs": {
|
||||
"url": "https://github.com/jshttp/negotiator/issues"
|
||||
},
|
||||
"homepage": "https://github.com/jshttp/negotiator",
|
||||
"_id": "negotiator@0.5.3",
|
||||
"_shasum": "269d5c476810ec92edbe7b6c2f28316384f9a7e8",
|
||||
"_from": "negotiator@0.5.3",
|
||||
"_npmVersion": "1.4.28",
|
||||
"_npmUser": {
|
||||
"name": "dougwilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
"maintainers": [
|
||||
{
|
||||
"name": "federomero",
|
||||
"email": "federomero@gmail.com"
|
||||
},
|
||||
{
|
||||
"name": "dougwilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
{
|
||||
"name": "jongleberry",
|
||||
"email": "jonathanrichardong@gmail.com"
|
||||
}
|
||||
],
|
||||
"dist": {
|
||||
"shasum": "269d5c476810ec92edbe7b6c2f28316384f9a7e8",
|
||||
"tarball": "http://registry.npmjs.org/negotiator/-/negotiator-0.5.3.tgz"
|
||||
},
|
||||
"directories": {},
|
||||
"_resolved": "https://registry.npmjs.org/negotiator/-/negotiator-0.5.3.tgz"
|
||||
}
|
97
node_modules/express/node_modules/accepts/package.json
generated
vendored
Normal file
97
node_modules/express/node_modules/accepts/package.json
generated
vendored
Normal file
@ -0,0 +1,97 @@
|
||||
{
|
||||
"name": "accepts",
|
||||
"description": "Higher-level content negotiation",
|
||||
"version": "1.2.13",
|
||||
"contributors": [
|
||||
{
|
||||
"name": "Douglas Christopher Wilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
{
|
||||
"name": "Jonathan Ong",
|
||||
"email": "me@jongleberry.com",
|
||||
"url": "http://jongleberry.com"
|
||||
}
|
||||
],
|
||||
"license": "MIT",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "https://github.com/jshttp/accepts"
|
||||
},
|
||||
"dependencies": {
|
||||
"mime-types": "~2.1.6",
|
||||
"negotiator": "0.5.3"
|
||||
},
|
||||
"devDependencies": {
|
||||
"istanbul": "0.3.19",
|
||||
"mocha": "~1.21.5"
|
||||
},
|
||||
"files": [
|
||||
"LICENSE",
|
||||
"HISTORY.md",
|
||||
"index.js"
|
||||
],
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "mocha --reporter spec --check-leaks --bail test/",
|
||||
"test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot --check-leaks test/",
|
||||
"test-travis": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --check-leaks test/"
|
||||
},
|
||||
"keywords": [
|
||||
"content",
|
||||
"negotiation",
|
||||
"accept",
|
||||
"accepts"
|
||||
],
|
||||
"gitHead": "b7e15ecb25dacc0b2133ed0553d64f8a79537e01",
|
||||
"bugs": {
|
||||
"url": "https://github.com/jshttp/accepts/issues"
|
||||
},
|
||||
"homepage": "https://github.com/jshttp/accepts",
|
||||
"_id": "accepts@1.2.13",
|
||||
"_shasum": "e5f1f3928c6d95fd96558c36ec3d9d0de4a6ecea",
|
||||
"_from": "accepts@~1.2.12",
|
||||
"_npmVersion": "1.4.28",
|
||||
"_npmUser": {
|
||||
"name": "dougwilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
"maintainers": [
|
||||
{
|
||||
"name": "jongleberry",
|
||||
"email": "jonathanrichardong@gmail.com"
|
||||
},
|
||||
{
|
||||
"name": "federomero",
|
||||
"email": "federomero@gmail.com"
|
||||
},
|
||||
{
|
||||
"name": "dougwilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
{
|
||||
"name": "fishrock123",
|
||||
"email": "fishrock123@rocketmail.com"
|
||||
},
|
||||
{
|
||||
"name": "tjholowaychuk",
|
||||
"email": "tj@vision-media.ca"
|
||||
},
|
||||
{
|
||||
"name": "mscdex",
|
||||
"email": "mscdex@mscdex.net"
|
||||
},
|
||||
{
|
||||
"name": "defunctzombie",
|
||||
"email": "shtylman@gmail.com"
|
||||
}
|
||||
],
|
||||
"dist": {
|
||||
"shasum": "e5f1f3928c6d95fd96558c36ec3d9d0de4a6ecea",
|
||||
"tarball": "http://registry.npmjs.org/accepts/-/accepts-1.2.13.tgz"
|
||||
},
|
||||
"directories": {},
|
||||
"_resolved": "https://registry.npmjs.org/accepts/-/accepts-1.2.13.tgz"
|
||||
}
|
21
node_modules/express/node_modules/array-flatten/LICENSE
generated
vendored
Normal file
21
node_modules/express/node_modules/array-flatten/LICENSE
generated
vendored
Normal file
@ -0,0 +1,21 @@
|
||||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) 2014 Blake Embrey (hello@blakeembrey.com)
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
43
node_modules/express/node_modules/array-flatten/README.md
generated
vendored
Normal file
43
node_modules/express/node_modules/array-flatten/README.md
generated
vendored
Normal file
@ -0,0 +1,43 @@
|
||||
# Array Flatten
|
||||
|
||||
[![NPM version][npm-image]][npm-url]
|
||||
[![NPM downloads][downloads-image]][downloads-url]
|
||||
[![Build status][travis-image]][travis-url]
|
||||
[![Test coverage][coveralls-image]][coveralls-url]
|
||||
|
||||
> Flatten an array of nested arrays into a single flat array. Accepts an optional depth.
|
||||
|
||||
## Installation
|
||||
|
||||
```
|
||||
npm install array-flatten --save
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
```javascript
|
||||
var flatten = require('array-flatten')
|
||||
|
||||
flatten([1, [2, [3, [4, [5], 6], 7], 8], 9])
|
||||
//=> [1, 2, 3, 4, 5, 6, 7, 8, 9]
|
||||
|
||||
flatten([1, [2, [3, [4, [5], 6], 7], 8], 9], 2)
|
||||
//=> [1, 2, 3, [4, [5], 6], 7, 8, 9]
|
||||
|
||||
(function () {
|
||||
flatten(arguments) //=> [1, 2, 3]
|
||||
})(1, [2, 3])
|
||||
```
|
||||
|
||||
## License
|
||||
|
||||
MIT
|
||||
|
||||
[npm-image]: https://img.shields.io/npm/v/array-flatten.svg?style=flat
|
||||
[npm-url]: https://npmjs.org/package/array-flatten
|
||||
[downloads-image]: https://img.shields.io/npm/dm/array-flatten.svg?style=flat
|
||||
[downloads-url]: https://npmjs.org/package/array-flatten
|
||||
[travis-image]: https://img.shields.io/travis/blakeembrey/array-flatten.svg?style=flat
|
||||
[travis-url]: https://travis-ci.org/blakeembrey/array-flatten
|
||||
[coveralls-image]: https://img.shields.io/coveralls/blakeembrey/array-flatten.svg?style=flat
|
||||
[coveralls-url]: https://coveralls.io/r/blakeembrey/array-flatten?branch=master
|
64
node_modules/express/node_modules/array-flatten/array-flatten.js
generated
vendored
Normal file
64
node_modules/express/node_modules/array-flatten/array-flatten.js
generated
vendored
Normal file
@ -0,0 +1,64 @@
|
||||
'use strict'
|
||||
|
||||
/**
|
||||
* Expose `arrayFlatten`.
|
||||
*/
|
||||
module.exports = arrayFlatten
|
||||
|
||||
/**
|
||||
* Recursive flatten function with depth.
|
||||
*
|
||||
* @param {Array} array
|
||||
* @param {Array} result
|
||||
* @param {Number} depth
|
||||
* @return {Array}
|
||||
*/
|
||||
function flattenWithDepth (array, result, depth) {
|
||||
for (var i = 0; i < array.length; i++) {
|
||||
var value = array[i]
|
||||
|
||||
if (depth > 0 && Array.isArray(value)) {
|
||||
flattenWithDepth(value, result, depth - 1)
|
||||
} else {
|
||||
result.push(value)
|
||||
}
|
||||
}
|
||||
|
||||
return result
|
||||
}
|
||||
|
||||
/**
|
||||
* Recursive flatten function. Omitting depth is slightly faster.
|
||||
*
|
||||
* @param {Array} array
|
||||
* @param {Array} result
|
||||
* @return {Array}
|
||||
*/
|
||||
function flattenForever (array, result) {
|
||||
for (var i = 0; i < array.length; i++) {
|
||||
var value = array[i]
|
||||
|
||||
if (Array.isArray(value)) {
|
||||
flattenForever(value, result)
|
||||
} else {
|
||||
result.push(value)
|
||||
}
|
||||
}
|
||||
|
||||
return result
|
||||
}
|
||||
|
||||
/**
|
||||
* Flatten an array, with the ability to define a depth.
|
||||
*
|
||||
* @param {Array} array
|
||||
* @param {Number} depth
|
||||
* @return {Array}
|
||||
*/
|
||||
function arrayFlatten (array, depth) {
|
||||
if (depth == null) {
|
||||
return flattenForever(array, [])
|
||||
}
|
||||
|
||||
return flattenWithDepth(array, [], depth)
|
||||
}
|
61
node_modules/express/node_modules/array-flatten/package.json
generated
vendored
Normal file
61
node_modules/express/node_modules/array-flatten/package.json
generated
vendored
Normal file
@ -0,0 +1,61 @@
|
||||
{
|
||||
"name": "array-flatten",
|
||||
"version": "1.1.1",
|
||||
"description": "Flatten an array of nested arrays into a single flat array",
|
||||
"main": "array-flatten.js",
|
||||
"files": [
|
||||
"array-flatten.js",
|
||||
"LICENSE"
|
||||
],
|
||||
"scripts": {
|
||||
"test": "istanbul cover _mocha -- -R spec"
|
||||
},
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git://github.com/blakeembrey/array-flatten.git"
|
||||
},
|
||||
"keywords": [
|
||||
"array",
|
||||
"flatten",
|
||||
"arguments",
|
||||
"depth"
|
||||
],
|
||||
"author": {
|
||||
"name": "Blake Embrey",
|
||||
"email": "hello@blakeembrey.com",
|
||||
"url": "http://blakeembrey.me"
|
||||
},
|
||||
"license": "MIT",
|
||||
"bugs": {
|
||||
"url": "https://github.com/blakeembrey/array-flatten/issues"
|
||||
},
|
||||
"homepage": "https://github.com/blakeembrey/array-flatten",
|
||||
"devDependencies": {
|
||||
"istanbul": "^0.3.13",
|
||||
"mocha": "^2.2.4",
|
||||
"pre-commit": "^1.0.7",
|
||||
"standard": "^3.7.3"
|
||||
},
|
||||
"gitHead": "1963a9189229d408e1e8f585a00c8be9edbd1803",
|
||||
"_id": "array-flatten@1.1.1",
|
||||
"_shasum": "9a5f699051b1e7073328f2a008968b64ea2955d2",
|
||||
"_from": "array-flatten@1.1.1",
|
||||
"_npmVersion": "2.11.3",
|
||||
"_nodeVersion": "2.3.3",
|
||||
"_npmUser": {
|
||||
"name": "blakeembrey",
|
||||
"email": "hello@blakeembrey.com"
|
||||
},
|
||||
"maintainers": [
|
||||
{
|
||||
"name": "blakeembrey",
|
||||
"email": "hello@blakeembrey.com"
|
||||
}
|
||||
],
|
||||
"dist": {
|
||||
"shasum": "9a5f699051b1e7073328f2a008968b64ea2955d2",
|
||||
"tarball": "http://registry.npmjs.org/array-flatten/-/array-flatten-1.1.1.tgz"
|
||||
},
|
||||
"directories": {},
|
||||
"_resolved": "https://registry.npmjs.org/array-flatten/-/array-flatten-1.1.1.tgz"
|
||||
}
|
45
node_modules/express/node_modules/content-disposition/HISTORY.md
generated
vendored
Normal file
45
node_modules/express/node_modules/content-disposition/HISTORY.md
generated
vendored
Normal file
@ -0,0 +1,45 @@
|
||||
0.5.1 / 2016-01-17
|
||||
==================
|
||||
|
||||
* perf: enable strict mode
|
||||
|
||||
0.5.0 / 2014-10-11
|
||||
==================
|
||||
|
||||
* Add `parse` function
|
||||
|
||||
0.4.0 / 2014-09-21
|
||||
==================
|
||||
|
||||
* Expand non-Unicode `filename` to the full ISO-8859-1 charset
|
||||
|
||||
0.3.0 / 2014-09-20
|
||||
==================
|
||||
|
||||
* Add `fallback` option
|
||||
* Add `type` option
|
||||
|
||||
0.2.0 / 2014-09-19
|
||||
==================
|
||||
|
||||
* Reduce ambiguity of file names with hex escape in buggy browsers
|
||||
|
||||
0.1.2 / 2014-09-19
|
||||
==================
|
||||
|
||||
* Fix periodic invalid Unicode filename header
|
||||
|
||||
0.1.1 / 2014-09-19
|
||||
==================
|
||||
|
||||
* Fix invalid characters appearing in `filename*` parameter
|
||||
|
||||
0.1.0 / 2014-09-18
|
||||
==================
|
||||
|
||||
* Make the `filename` argument optional
|
||||
|
||||
0.0.0 / 2014-09-18
|
||||
==================
|
||||
|
||||
* Initial release
|
22
node_modules/express/node_modules/content-disposition/LICENSE
generated
vendored
Normal file
22
node_modules/express/node_modules/content-disposition/LICENSE
generated
vendored
Normal file
@ -0,0 +1,22 @@
|
||||
(The MIT License)
|
||||
|
||||
Copyright (c) 2014 Douglas Christopher Wilson
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of this software and associated documentation files (the
|
||||
'Software'), to deal in the Software without restriction, including
|
||||
without limitation the rights to use, copy, modify, merge, publish,
|
||||
distribute, sublicense, and/or sell copies of the Software, and to
|
||||
permit persons to whom the Software is furnished to do so, subject to
|
||||
the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
|
||||
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
|
||||
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
|
||||
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
|
||||
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
141
node_modules/express/node_modules/content-disposition/README.md
generated
vendored
Normal file
141
node_modules/express/node_modules/content-disposition/README.md
generated
vendored
Normal file
@ -0,0 +1,141 @@
|
||||
# content-disposition
|
||||
|
||||
[![NPM Version][npm-image]][npm-url]
|
||||
[![NPM Downloads][downloads-image]][downloads-url]
|
||||
[![Node.js Version][node-version-image]][node-version-url]
|
||||
[![Build Status][travis-image]][travis-url]
|
||||
[![Test Coverage][coveralls-image]][coveralls-url]
|
||||
|
||||
Create and parse HTTP `Content-Disposition` header
|
||||
|
||||
## Installation
|
||||
|
||||
```sh
|
||||
$ npm install content-disposition
|
||||
```
|
||||
|
||||
## API
|
||||
|
||||
```js
|
||||
var contentDisposition = require('content-disposition')
|
||||
```
|
||||
|
||||
### contentDisposition(filename, options)
|
||||
|
||||
Create an attachment `Content-Disposition` header value using the given file name,
|
||||
if supplied. The `filename` is optional and if no file name is desired, but you
|
||||
want to specify `options`, set `filename` to `undefined`.
|
||||
|
||||
```js
|
||||
res.setHeader('Content-Disposition', contentDisposition('∫ maths.pdf'))
|
||||
```
|
||||
|
||||
**note** HTTP headers are of the ISO-8859-1 character set. If you are writing this
|
||||
header through a means different from `setHeader` in Node.js, you'll want to specify
|
||||
the `'binary'` encoding in Node.js.
|
||||
|
||||
#### Options
|
||||
|
||||
`contentDisposition` accepts these properties in the options object.
|
||||
|
||||
##### fallback
|
||||
|
||||
If the `filename` option is outside ISO-8859-1, then the file name is actually
|
||||
stored in a supplemental field for clients that support Unicode file names and
|
||||
a ISO-8859-1 version of the file name is automatically generated.
|
||||
|
||||
This specifies the ISO-8859-1 file name to override the automatic generation or
|
||||
disables the generation all together, defaults to `true`.
|
||||
|
||||
- A string will specify the ISO-8859-1 file name to use in place of automatic
|
||||
generation.
|
||||
- `false` will disable including a ISO-8859-1 file name and only include the
|
||||
Unicode version (unless the file name is already ISO-8859-1).
|
||||
- `true` will enable automatic generation if the file name is outside ISO-8859-1.
|
||||
|
||||
If the `filename` option is ISO-8859-1 and this option is specified and has a
|
||||
different value, then the `filename` option is encoded in the extended field
|
||||
and this set as the fallback field, even though they are both ISO-8859-1.
|
||||
|
||||
##### type
|
||||
|
||||
Specifies the disposition type, defaults to `"attachment"`. This can also be
|
||||
`"inline"`, or any other value (all values except inline are treated like
|
||||
`attachment`, but can convey additional information if both parties agree to
|
||||
it). The type is normalized to lower-case.
|
||||
|
||||
### contentDisposition.parse(string)
|
||||
|
||||
```js
|
||||
var disposition = contentDisposition.parse('attachment; filename="EURO rates.txt"; filename*=UTF-8\'\'%e2%82%ac%20rates.txt');
|
||||
```
|
||||
|
||||
Parse a `Content-Disposition` header string. This automatically handles extended
|
||||
("Unicode") parameters by decoding them and providing them under the standard
|
||||
parameter name. This will return an object with the following properties (examples
|
||||
are shown for the string `'attachment; filename="EURO rates.txt"; filename*=UTF-8\'\'%e2%82%ac%20rates.txt'`):
|
||||
|
||||
- `type`: The disposition type (always lower case). Example: `'attachment'`
|
||||
|
||||
- `parameters`: An object of the parameters in the disposition (name of parameter
|
||||
always lower case and extended versions replace non-extended versions). Example:
|
||||
`{filename: "€ rates.txt"}`
|
||||
|
||||
## Examples
|
||||
|
||||
### Send a file for download
|
||||
|
||||
```js
|
||||
var contentDisposition = require('content-disposition')
|
||||
var destroy = require('destroy')
|
||||
var http = require('http')
|
||||
var onFinished = require('on-finished')
|
||||
|
||||
var filePath = '/path/to/public/plans.pdf'
|
||||
|
||||
http.createServer(function onRequest(req, res) {
|
||||
// set headers
|
||||
res.setHeader('Content-Type', 'application/pdf')
|
||||
res.setHeader('Content-Disposition', contentDisposition(filePath))
|
||||
|
||||
// send file
|
||||
var stream = fs.createReadStream(filePath)
|
||||
stream.pipe(res)
|
||||
onFinished(res, function (err) {
|
||||
destroy(stream)
|
||||
})
|
||||
})
|
||||
```
|
||||
|
||||
## Testing
|
||||
|
||||
```sh
|
||||
$ npm test
|
||||
```
|
||||
|
||||
## References
|
||||
|
||||
- [RFC 2616: Hypertext Transfer Protocol -- HTTP/1.1][rfc-2616]
|
||||
- [RFC 5987: Character Set and Language Encoding for Hypertext Transfer Protocol (HTTP) Header Field Parameters][rfc-5987]
|
||||
- [RFC 6266: Use of the Content-Disposition Header Field in the Hypertext Transfer Protocol (HTTP)][rfc-6266]
|
||||
- [Test Cases for HTTP Content-Disposition header field (RFC 6266) and the Encodings defined in RFCs 2047, 2231 and 5987][tc-2231]
|
||||
|
||||
[rfc-2616]: https://tools.ietf.org/html/rfc2616
|
||||
[rfc-5987]: https://tools.ietf.org/html/rfc5987
|
||||
[rfc-6266]: https://tools.ietf.org/html/rfc6266
|
||||
[tc-2231]: http://greenbytes.de/tech/tc2231/
|
||||
|
||||
## License
|
||||
|
||||
[MIT](LICENSE)
|
||||
|
||||
[npm-image]: https://img.shields.io/npm/v/content-disposition.svg?style=flat
|
||||
[npm-url]: https://npmjs.org/package/content-disposition
|
||||
[node-version-image]: https://img.shields.io/node/v/content-disposition.svg?style=flat
|
||||
[node-version-url]: http://nodejs.org/download/
|
||||
[travis-image]: https://img.shields.io/travis/jshttp/content-disposition.svg?style=flat
|
||||
[travis-url]: https://travis-ci.org/jshttp/content-disposition
|
||||
[coveralls-image]: https://img.shields.io/coveralls/jshttp/content-disposition.svg?style=flat
|
||||
[coveralls-url]: https://coveralls.io/r/jshttp/content-disposition?branch=master
|
||||
[downloads-image]: https://img.shields.io/npm/dm/content-disposition.svg?style=flat
|
||||
[downloads-url]: https://npmjs.org/package/content-disposition
|
445
node_modules/express/node_modules/content-disposition/index.js
generated
vendored
Normal file
445
node_modules/express/node_modules/content-disposition/index.js
generated
vendored
Normal file
@ -0,0 +1,445 @@
|
||||
/*!
|
||||
* content-disposition
|
||||
* Copyright(c) 2014 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict'
|
||||
|
||||
/**
|
||||
* Module exports.
|
||||
*/
|
||||
|
||||
module.exports = contentDisposition
|
||||
module.exports.parse = parse
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
*/
|
||||
|
||||
var basename = require('path').basename
|
||||
|
||||
/**
|
||||
* RegExp to match non attr-char, *after* encodeURIComponent (i.e. not including "%")
|
||||
*/
|
||||
|
||||
var encodeUriAttrCharRegExp = /[\x00-\x20"'\(\)*,\/:;<=>?@\[\\\]\{\}\x7f]/g
|
||||
|
||||
/**
|
||||
* RegExp to match percent encoding escape.
|
||||
*/
|
||||
|
||||
var hexEscapeRegExp = /%[0-9A-Fa-f]{2}/
|
||||
var hexEscapeReplaceRegExp = /%([0-9A-Fa-f]{2})/g
|
||||
|
||||
/**
|
||||
* RegExp to match non-latin1 characters.
|
||||
*/
|
||||
|
||||
var nonLatin1RegExp = /[^\x20-\x7e\xa0-\xff]/g
|
||||
|
||||
/**
|
||||
* RegExp to match quoted-pair in RFC 2616
|
||||
*
|
||||
* quoted-pair = "\" CHAR
|
||||
* CHAR = <any US-ASCII character (octets 0 - 127)>
|
||||
*/
|
||||
|
||||
var qescRegExp = /\\([\u0000-\u007f])/g;
|
||||
|
||||
/**
|
||||
* RegExp to match chars that must be quoted-pair in RFC 2616
|
||||
*/
|
||||
|
||||
var quoteRegExp = /([\\"])/g
|
||||
|
||||
/**
|
||||
* RegExp for various RFC 2616 grammar
|
||||
*
|
||||
* parameter = token "=" ( token | quoted-string )
|
||||
* token = 1*<any CHAR except CTLs or separators>
|
||||
* separators = "(" | ")" | "<" | ">" | "@"
|
||||
* | "," | ";" | ":" | "\" | <">
|
||||
* | "/" | "[" | "]" | "?" | "="
|
||||
* | "{" | "}" | SP | HT
|
||||
* quoted-string = ( <"> *(qdtext | quoted-pair ) <"> )
|
||||
* qdtext = <any TEXT except <">>
|
||||
* quoted-pair = "\" CHAR
|
||||
* CHAR = <any US-ASCII character (octets 0 - 127)>
|
||||
* TEXT = <any OCTET except CTLs, but including LWS>
|
||||
* LWS = [CRLF] 1*( SP | HT )
|
||||
* CRLF = CR LF
|
||||
* CR = <US-ASCII CR, carriage return (13)>
|
||||
* LF = <US-ASCII LF, linefeed (10)>
|
||||
* SP = <US-ASCII SP, space (32)>
|
||||
* HT = <US-ASCII HT, horizontal-tab (9)>
|
||||
* CTL = <any US-ASCII control character (octets 0 - 31) and DEL (127)>
|
||||
* OCTET = <any 8-bit sequence of data>
|
||||
*/
|
||||
|
||||
var paramRegExp = /; *([!#$%&'\*\+\-\.0-9A-Z\^_`a-z\|~]+) *= *("(?:[ !\x23-\x5b\x5d-\x7e\x80-\xff]|\\[\x20-\x7e])*"|[!#$%&'\*\+\-\.0-9A-Z\^_`a-z\|~]+) */g
|
||||
var textRegExp = /^[\x20-\x7e\x80-\xff]+$/
|
||||
var tokenRegExp = /^[!#$%&'\*\+\-\.0-9A-Z\^_`a-z\|~]+$/
|
||||
|
||||
/**
|
||||
* RegExp for various RFC 5987 grammar
|
||||
*
|
||||
* ext-value = charset "'" [ language ] "'" value-chars
|
||||
* charset = "UTF-8" / "ISO-8859-1" / mime-charset
|
||||
* mime-charset = 1*mime-charsetc
|
||||
* mime-charsetc = ALPHA / DIGIT
|
||||
* / "!" / "#" / "$" / "%" / "&"
|
||||
* / "+" / "-" / "^" / "_" / "`"
|
||||
* / "{" / "}" / "~"
|
||||
* language = ( 2*3ALPHA [ extlang ] )
|
||||
* / 4ALPHA
|
||||
* / 5*8ALPHA
|
||||
* extlang = *3( "-" 3ALPHA )
|
||||
* value-chars = *( pct-encoded / attr-char )
|
||||
* pct-encoded = "%" HEXDIG HEXDIG
|
||||
* attr-char = ALPHA / DIGIT
|
||||
* / "!" / "#" / "$" / "&" / "+" / "-" / "."
|
||||
* / "^" / "_" / "`" / "|" / "~"
|
||||
*/
|
||||
|
||||
var extValueRegExp = /^([A-Za-z0-9!#$%&+\-^_`{}~]+)'(?:[A-Za-z]{2,3}(?:-[A-Za-z]{3}){0,3}|[A-Za-z]{4,8}|)'((?:%[0-9A-Fa-f]{2}|[A-Za-z0-9!#$&+\-\.^_`|~])+)$/
|
||||
|
||||
/**
|
||||
* RegExp for various RFC 6266 grammar
|
||||
*
|
||||
* disposition-type = "inline" | "attachment" | disp-ext-type
|
||||
* disp-ext-type = token
|
||||
* disposition-parm = filename-parm | disp-ext-parm
|
||||
* filename-parm = "filename" "=" value
|
||||
* | "filename*" "=" ext-value
|
||||
* disp-ext-parm = token "=" value
|
||||
* | ext-token "=" ext-value
|
||||
* ext-token = <the characters in token, followed by "*">
|
||||
*/
|
||||
|
||||
var dispositionTypeRegExp = /^([!#$%&'\*\+\-\.0-9A-Z\^_`a-z\|~]+) *(?:$|;)/
|
||||
|
||||
/**
|
||||
* Create an attachment Content-Disposition header.
|
||||
*
|
||||
* @param {string} [filename]
|
||||
* @param {object} [options]
|
||||
* @param {string} [options.type=attachment]
|
||||
* @param {string|boolean} [options.fallback=true]
|
||||
* @return {string}
|
||||
* @api public
|
||||
*/
|
||||
|
||||
function contentDisposition(filename, options) {
|
||||
var opts = options || {}
|
||||
|
||||
// get type
|
||||
var type = opts.type || 'attachment'
|
||||
|
||||
// get parameters
|
||||
var params = createparams(filename, opts.fallback)
|
||||
|
||||
// format into string
|
||||
return format(new ContentDisposition(type, params))
|
||||
}
|
||||
|
||||
/**
|
||||
* Create parameters object from filename and fallback.
|
||||
*
|
||||
* @param {string} [filename]
|
||||
* @param {string|boolean} [fallback=true]
|
||||
* @return {object}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function createparams(filename, fallback) {
|
||||
if (filename === undefined) {
|
||||
return
|
||||
}
|
||||
|
||||
var params = {}
|
||||
|
||||
if (typeof filename !== 'string') {
|
||||
throw new TypeError('filename must be a string')
|
||||
}
|
||||
|
||||
// fallback defaults to true
|
||||
if (fallback === undefined) {
|
||||
fallback = true
|
||||
}
|
||||
|
||||
if (typeof fallback !== 'string' && typeof fallback !== 'boolean') {
|
||||
throw new TypeError('fallback must be a string or boolean')
|
||||
}
|
||||
|
||||
if (typeof fallback === 'string' && nonLatin1RegExp.test(fallback)) {
|
||||
throw new TypeError('fallback must be ISO-8859-1 string')
|
||||
}
|
||||
|
||||
// restrict to file base name
|
||||
var name = basename(filename)
|
||||
|
||||
// determine if name is suitable for quoted string
|
||||
var isQuotedString = textRegExp.test(name)
|
||||
|
||||
// generate fallback name
|
||||
var fallbackName = typeof fallback !== 'string'
|
||||
? fallback && getlatin1(name)
|
||||
: basename(fallback)
|
||||
var hasFallback = typeof fallbackName === 'string' && fallbackName !== name
|
||||
|
||||
// set extended filename parameter
|
||||
if (hasFallback || !isQuotedString || hexEscapeRegExp.test(name)) {
|
||||
params['filename*'] = name
|
||||
}
|
||||
|
||||
// set filename parameter
|
||||
if (isQuotedString || hasFallback) {
|
||||
params.filename = hasFallback
|
||||
? fallbackName
|
||||
: name
|
||||
}
|
||||
|
||||
return params
|
||||
}
|
||||
|
||||
/**
|
||||
* Format object to Content-Disposition header.
|
||||
*
|
||||
* @param {object} obj
|
||||
* @param {string} obj.type
|
||||
* @param {object} [obj.parameters]
|
||||
* @return {string}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function format(obj) {
|
||||
var parameters = obj.parameters
|
||||
var type = obj.type
|
||||
|
||||
if (!type || typeof type !== 'string' || !tokenRegExp.test(type)) {
|
||||
throw new TypeError('invalid type')
|
||||
}
|
||||
|
||||
// start with normalized type
|
||||
var string = String(type).toLowerCase()
|
||||
|
||||
// append parameters
|
||||
if (parameters && typeof parameters === 'object') {
|
||||
var param
|
||||
var params = Object.keys(parameters).sort()
|
||||
|
||||
for (var i = 0; i < params.length; i++) {
|
||||
param = params[i]
|
||||
|
||||
var val = param.substr(-1) === '*'
|
||||
? ustring(parameters[param])
|
||||
: qstring(parameters[param])
|
||||
|
||||
string += '; ' + param + '=' + val
|
||||
}
|
||||
}
|
||||
|
||||
return string
|
||||
}
|
||||
|
||||
/**
|
||||
* Decode a RFC 6987 field value (gracefully).
|
||||
*
|
||||
* @param {string} str
|
||||
* @return {string}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function decodefield(str) {
|
||||
var match = extValueRegExp.exec(str)
|
||||
|
||||
if (!match) {
|
||||
throw new TypeError('invalid extended field value')
|
||||
}
|
||||
|
||||
var charset = match[1].toLowerCase()
|
||||
var encoded = match[2]
|
||||
var value
|
||||
|
||||
// to binary string
|
||||
var binary = encoded.replace(hexEscapeReplaceRegExp, pdecode)
|
||||
|
||||
switch (charset) {
|
||||
case 'iso-8859-1':
|
||||
value = getlatin1(binary)
|
||||
break
|
||||
case 'utf-8':
|
||||
value = new Buffer(binary, 'binary').toString('utf8')
|
||||
break
|
||||
default:
|
||||
throw new TypeError('unsupported charset in extended field')
|
||||
}
|
||||
|
||||
return value
|
||||
}
|
||||
|
||||
/**
|
||||
* Get ISO-8859-1 version of string.
|
||||
*
|
||||
* @param {string} val
|
||||
* @return {string}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function getlatin1(val) {
|
||||
// simple Unicode -> ISO-8859-1 transformation
|
||||
return String(val).replace(nonLatin1RegExp, '?')
|
||||
}
|
||||
|
||||
/**
|
||||
* Parse Content-Disposition header string.
|
||||
*
|
||||
* @param {string} string
|
||||
* @return {object}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function parse(string) {
|
||||
if (!string || typeof string !== 'string') {
|
||||
throw new TypeError('argument string is required')
|
||||
}
|
||||
|
||||
var match = dispositionTypeRegExp.exec(string)
|
||||
|
||||
if (!match) {
|
||||
throw new TypeError('invalid type format')
|
||||
}
|
||||
|
||||
// normalize type
|
||||
var index = match[0].length
|
||||
var type = match[1].toLowerCase()
|
||||
|
||||
var key
|
||||
var names = []
|
||||
var params = {}
|
||||
var value
|
||||
|
||||
// calculate index to start at
|
||||
index = paramRegExp.lastIndex = match[0].substr(-1) === ';'
|
||||
? index - 1
|
||||
: index
|
||||
|
||||
// match parameters
|
||||
while (match = paramRegExp.exec(string)) {
|
||||
if (match.index !== index) {
|
||||
throw new TypeError('invalid parameter format')
|
||||
}
|
||||
|
||||
index += match[0].length
|
||||
key = match[1].toLowerCase()
|
||||
value = match[2]
|
||||
|
||||
if (names.indexOf(key) !== -1) {
|
||||
throw new TypeError('invalid duplicate parameter')
|
||||
}
|
||||
|
||||
names.push(key)
|
||||
|
||||
if (key.indexOf('*') + 1 === key.length) {
|
||||
// decode extended value
|
||||
key = key.slice(0, -1)
|
||||
value = decodefield(value)
|
||||
|
||||
// overwrite existing value
|
||||
params[key] = value
|
||||
continue
|
||||
}
|
||||
|
||||
if (typeof params[key] === 'string') {
|
||||
continue
|
||||
}
|
||||
|
||||
if (value[0] === '"') {
|
||||
// remove quotes and escapes
|
||||
value = value
|
||||
.substr(1, value.length - 2)
|
||||
.replace(qescRegExp, '$1')
|
||||
}
|
||||
|
||||
params[key] = value
|
||||
}
|
||||
|
||||
if (index !== -1 && index !== string.length) {
|
||||
throw new TypeError('invalid parameter format')
|
||||
}
|
||||
|
||||
return new ContentDisposition(type, params)
|
||||
}
|
||||
|
||||
/**
|
||||
* Percent decode a single character.
|
||||
*
|
||||
* @param {string} str
|
||||
* @param {string} hex
|
||||
* @return {string}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function pdecode(str, hex) {
|
||||
return String.fromCharCode(parseInt(hex, 16))
|
||||
}
|
||||
|
||||
/**
|
||||
* Percent encode a single character.
|
||||
*
|
||||
* @param {string} char
|
||||
* @return {string}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function pencode(char) {
|
||||
var hex = String(char)
|
||||
.charCodeAt(0)
|
||||
.toString(16)
|
||||
.toUpperCase()
|
||||
return hex.length === 1
|
||||
? '%0' + hex
|
||||
: '%' + hex
|
||||
}
|
||||
|
||||
/**
|
||||
* Quote a string for HTTP.
|
||||
*
|
||||
* @param {string} val
|
||||
* @return {string}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function qstring(val) {
|
||||
var str = String(val)
|
||||
|
||||
return '"' + str.replace(quoteRegExp, '\\$1') + '"'
|
||||
}
|
||||
|
||||
/**
|
||||
* Encode a Unicode string for HTTP (RFC 5987).
|
||||
*
|
||||
* @param {string} val
|
||||
* @return {string}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function ustring(val) {
|
||||
var str = String(val)
|
||||
|
||||
// percent encode as UTF-8
|
||||
var encoded = encodeURIComponent(str)
|
||||
.replace(encodeUriAttrCharRegExp, pencode)
|
||||
|
||||
return 'UTF-8\'\'' + encoded
|
||||
}
|
||||
|
||||
/**
|
||||
* Class for parsed Content-Disposition header for v8 optimization
|
||||
*/
|
||||
|
||||
function ContentDisposition(type, parameters) {
|
||||
this.type = type
|
||||
this.parameters = parameters
|
||||
}
|
65
node_modules/express/node_modules/content-disposition/package.json
generated
vendored
Normal file
65
node_modules/express/node_modules/content-disposition/package.json
generated
vendored
Normal file
@ -0,0 +1,65 @@
|
||||
{
|
||||
"name": "content-disposition",
|
||||
"description": "Create and parse Content-Disposition header",
|
||||
"version": "0.5.1",
|
||||
"contributors": [
|
||||
{
|
||||
"name": "Douglas Christopher Wilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
}
|
||||
],
|
||||
"license": "MIT",
|
||||
"keywords": [
|
||||
"content-disposition",
|
||||
"http",
|
||||
"rfc6266",
|
||||
"res"
|
||||
],
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "https://github.com/jshttp/content-disposition"
|
||||
},
|
||||
"devDependencies": {
|
||||
"istanbul": "0.4.2",
|
||||
"mocha": "1.21.5"
|
||||
},
|
||||
"files": [
|
||||
"LICENSE",
|
||||
"HISTORY.md",
|
||||
"README.md",
|
||||
"index.js"
|
||||
],
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "mocha --reporter spec --bail --check-leaks test/",
|
||||
"test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot --check-leaks test/",
|
||||
"test-travis": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --check-leaks test/"
|
||||
},
|
||||
"gitHead": "7b391db3af5629d4c698f1de21802940bb9f22a5",
|
||||
"bugs": {
|
||||
"url": "https://github.com/jshttp/content-disposition/issues"
|
||||
},
|
||||
"homepage": "https://github.com/jshttp/content-disposition",
|
||||
"_id": "content-disposition@0.5.1",
|
||||
"_shasum": "87476c6a67c8daa87e32e87616df883ba7fb071b",
|
||||
"_from": "content-disposition@0.5.1",
|
||||
"_npmVersion": "1.4.28",
|
||||
"_npmUser": {
|
||||
"name": "dougwilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
"maintainers": [
|
||||
{
|
||||
"name": "dougwilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
}
|
||||
],
|
||||
"dist": {
|
||||
"shasum": "87476c6a67c8daa87e32e87616df883ba7fb071b",
|
||||
"tarball": "http://registry.npmjs.org/content-disposition/-/content-disposition-0.5.1.tgz"
|
||||
},
|
||||
"directories": {},
|
||||
"_resolved": "https://registry.npmjs.org/content-disposition/-/content-disposition-0.5.1.tgz"
|
||||
}
|
9
node_modules/express/node_modules/content-type/HISTORY.md
generated
vendored
Normal file
9
node_modules/express/node_modules/content-type/HISTORY.md
generated
vendored
Normal file
@ -0,0 +1,9 @@
|
||||
1.0.1 / 2015-02-13
|
||||
==================
|
||||
|
||||
* Improve missing `Content-Type` header error message
|
||||
|
||||
1.0.0 / 2015-02-01
|
||||
==================
|
||||
|
||||
* Initial implementation, derived from `media-typer@0.3.0`
|
22
node_modules/express/node_modules/content-type/LICENSE
generated
vendored
Normal file
22
node_modules/express/node_modules/content-type/LICENSE
generated
vendored
Normal file
@ -0,0 +1,22 @@
|
||||
(The MIT License)
|
||||
|
||||
Copyright (c) 2015 Douglas Christopher Wilson
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of this software and associated documentation files (the
|
||||
'Software'), to deal in the Software without restriction, including
|
||||
without limitation the rights to use, copy, modify, merge, publish,
|
||||
distribute, sublicense, and/or sell copies of the Software, and to
|
||||
permit persons to whom the Software is furnished to do so, subject to
|
||||
the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
|
||||
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
|
||||
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
|
||||
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
|
||||
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
92
node_modules/express/node_modules/content-type/README.md
generated
vendored
Normal file
92
node_modules/express/node_modules/content-type/README.md
generated
vendored
Normal file
@ -0,0 +1,92 @@
|
||||
# content-type
|
||||
|
||||
[![NPM Version][npm-image]][npm-url]
|
||||
[![NPM Downloads][downloads-image]][downloads-url]
|
||||
[![Node.js Version][node-version-image]][node-version-url]
|
||||
[![Build Status][travis-image]][travis-url]
|
||||
[![Test Coverage][coveralls-image]][coveralls-url]
|
||||
|
||||
Create and parse HTTP Content-Type header according to RFC 7231
|
||||
|
||||
## Installation
|
||||
|
||||
```sh
|
||||
$ npm install content-type
|
||||
```
|
||||
|
||||
## API
|
||||
|
||||
```js
|
||||
var contentType = require('content-type')
|
||||
```
|
||||
|
||||
### contentType.parse(string)
|
||||
|
||||
```js
|
||||
var obj = contentType.parse('image/svg+xml; charset=utf-8')
|
||||
```
|
||||
|
||||
Parse a content type string. This will return an object with the following
|
||||
properties (examples are shown for the string `'image/svg+xml; charset=utf-8'`):
|
||||
|
||||
- `type`: The media type (the type and subtype, always lower case).
|
||||
Example: `'image/svg+xml'`
|
||||
|
||||
- `parameters`: An object of the parameters in the media type (name of parameter
|
||||
always lower case). Example: `{charset: 'utf-8'}`
|
||||
|
||||
Throws a `TypeError` if the string is missing or invalid.
|
||||
|
||||
### contentType.parse(req)
|
||||
|
||||
```js
|
||||
var obj = contentType.parse(req)
|
||||
```
|
||||
|
||||
Parse the `content-type` header from the given `req`. Short-cut for
|
||||
`contentType.parse(req.headers['content-type'])`.
|
||||
|
||||
Throws a `TypeError` if the `Content-Type` header is missing or invalid.
|
||||
|
||||
### contentType.parse(res)
|
||||
|
||||
```js
|
||||
var obj = contentType.parse(res)
|
||||
```
|
||||
|
||||
Parse the `content-type` header set on the given `res`. Short-cut for
|
||||
`contentType.parse(res.getHeader('content-type'))`.
|
||||
|
||||
Throws a `TypeError` if the `Content-Type` header is missing or invalid.
|
||||
|
||||
### contentType.format(obj)
|
||||
|
||||
```js
|
||||
var str = contentType.format({type: 'image/svg+xml'})
|
||||
```
|
||||
|
||||
Format an object into a content type string. This will return a string of the
|
||||
content type for the given object with the following properties (examples are
|
||||
shown that produce the string `'image/svg+xml; charset=utf-8'`):
|
||||
|
||||
- `type`: The media type (will be lower-cased). Example: `'image/svg+xml'`
|
||||
|
||||
- `parameters`: An object of the parameters in the media type (name of the
|
||||
parameter will be lower-cased). Example: `{charset: 'utf-8'}`
|
||||
|
||||
Throws a `TypeError` if the object contains an invalid type or parameter names.
|
||||
|
||||
## License
|
||||
|
||||
[MIT](LICENSE)
|
||||
|
||||
[npm-image]: https://img.shields.io/npm/v/content-type.svg
|
||||
[npm-url]: https://npmjs.org/package/content-type
|
||||
[node-version-image]: https://img.shields.io/node/v/content-type.svg
|
||||
[node-version-url]: http://nodejs.org/download/
|
||||
[travis-image]: https://img.shields.io/travis/jshttp/content-type/master.svg
|
||||
[travis-url]: https://travis-ci.org/jshttp/content-type
|
||||
[coveralls-image]: https://img.shields.io/coveralls/jshttp/content-type/master.svg
|
||||
[coveralls-url]: https://coveralls.io/r/jshttp/content-type
|
||||
[downloads-image]: https://img.shields.io/npm/dm/content-type.svg
|
||||
[downloads-url]: https://npmjs.org/package/content-type
|
214
node_modules/express/node_modules/content-type/index.js
generated
vendored
Normal file
214
node_modules/express/node_modules/content-type/index.js
generated
vendored
Normal file
@ -0,0 +1,214 @@
|
||||
/*!
|
||||
* content-type
|
||||
* Copyright(c) 2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
/**
|
||||
* RegExp to match *( ";" parameter ) in RFC 7231 sec 3.1.1.1
|
||||
*
|
||||
* parameter = token "=" ( token / quoted-string )
|
||||
* token = 1*tchar
|
||||
* tchar = "!" / "#" / "$" / "%" / "&" / "'" / "*"
|
||||
* / "+" / "-" / "." / "^" / "_" / "`" / "|" / "~"
|
||||
* / DIGIT / ALPHA
|
||||
* ; any VCHAR, except delimiters
|
||||
* quoted-string = DQUOTE *( qdtext / quoted-pair ) DQUOTE
|
||||
* qdtext = HTAB / SP / %x21 / %x23-5B / %x5D-7E / obs-text
|
||||
* obs-text = %x80-FF
|
||||
* quoted-pair = "\" ( HTAB / SP / VCHAR / obs-text )
|
||||
*/
|
||||
var paramRegExp = /; *([!#$%&'\*\+\-\.\^_`\|~0-9A-Za-z]+) *= *("(?:[\u000b\u0020\u0021\u0023-\u005b\u005d-\u007e\u0080-\u00ff]|\\[\u000b\u0020-\u00ff])*"|[!#$%&'\*\+\-\.\^_`\|~0-9A-Za-z]+) */g
|
||||
var textRegExp = /^[\u000b\u0020-\u007e\u0080-\u00ff]+$/
|
||||
var tokenRegExp = /^[!#$%&'\*\+\-\.\^_`\|~0-9A-Za-z]+$/
|
||||
|
||||
/**
|
||||
* RegExp to match quoted-pair in RFC 7230 sec 3.2.6
|
||||
*
|
||||
* quoted-pair = "\" ( HTAB / SP / VCHAR / obs-text )
|
||||
* obs-text = %x80-FF
|
||||
*/
|
||||
var qescRegExp = /\\([\u000b\u0020-\u00ff])/g
|
||||
|
||||
/**
|
||||
* RegExp to match chars that must be quoted-pair in RFC 7230 sec 3.2.6
|
||||
*/
|
||||
var quoteRegExp = /([\\"])/g
|
||||
|
||||
/**
|
||||
* RegExp to match type in RFC 6838
|
||||
*
|
||||
* media-type = type "/" subtype
|
||||
* type = token
|
||||
* subtype = token
|
||||
*/
|
||||
var typeRegExp = /^[!#$%&'\*\+\-\.\^_`\|~0-9A-Za-z]+\/[!#$%&'\*\+\-\.\^_`\|~0-9A-Za-z]+$/
|
||||
|
||||
/**
|
||||
* Module exports.
|
||||
* @public
|
||||
*/
|
||||
|
||||
exports.format = format
|
||||
exports.parse = parse
|
||||
|
||||
/**
|
||||
* Format object to media type.
|
||||
*
|
||||
* @param {object} obj
|
||||
* @return {string}
|
||||
* @public
|
||||
*/
|
||||
|
||||
function format(obj) {
|
||||
if (!obj || typeof obj !== 'object') {
|
||||
throw new TypeError('argument obj is required')
|
||||
}
|
||||
|
||||
var parameters = obj.parameters
|
||||
var type = obj.type
|
||||
|
||||
if (!type || !typeRegExp.test(type)) {
|
||||
throw new TypeError('invalid type')
|
||||
}
|
||||
|
||||
var string = type
|
||||
|
||||
// append parameters
|
||||
if (parameters && typeof parameters === 'object') {
|
||||
var param
|
||||
var params = Object.keys(parameters).sort()
|
||||
|
||||
for (var i = 0; i < params.length; i++) {
|
||||
param = params[i]
|
||||
|
||||
if (!tokenRegExp.test(param)) {
|
||||
throw new TypeError('invalid parameter name')
|
||||
}
|
||||
|
||||
string += '; ' + param + '=' + qstring(parameters[param])
|
||||
}
|
||||
}
|
||||
|
||||
return string
|
||||
}
|
||||
|
||||
/**
|
||||
* Parse media type to object.
|
||||
*
|
||||
* @param {string|object} string
|
||||
* @return {Object}
|
||||
* @public
|
||||
*/
|
||||
|
||||
function parse(string) {
|
||||
if (!string) {
|
||||
throw new TypeError('argument string is required')
|
||||
}
|
||||
|
||||
if (typeof string === 'object') {
|
||||
// support req/res-like objects as argument
|
||||
string = getcontenttype(string)
|
||||
|
||||
if (typeof string !== 'string') {
|
||||
throw new TypeError('content-type header is missing from object');
|
||||
}
|
||||
}
|
||||
|
||||
if (typeof string !== 'string') {
|
||||
throw new TypeError('argument string is required to be a string')
|
||||
}
|
||||
|
||||
var index = string.indexOf(';')
|
||||
var type = index !== -1
|
||||
? string.substr(0, index).trim()
|
||||
: string.trim()
|
||||
|
||||
if (!typeRegExp.test(type)) {
|
||||
throw new TypeError('invalid media type')
|
||||
}
|
||||
|
||||
var key
|
||||
var match
|
||||
var obj = new ContentType(type.toLowerCase())
|
||||
var value
|
||||
|
||||
paramRegExp.lastIndex = index
|
||||
|
||||
while (match = paramRegExp.exec(string)) {
|
||||
if (match.index !== index) {
|
||||
throw new TypeError('invalid parameter format')
|
||||
}
|
||||
|
||||
index += match[0].length
|
||||
key = match[1].toLowerCase()
|
||||
value = match[2]
|
||||
|
||||
if (value[0] === '"') {
|
||||
// remove quotes and escapes
|
||||
value = value
|
||||
.substr(1, value.length - 2)
|
||||
.replace(qescRegExp, '$1')
|
||||
}
|
||||
|
||||
obj.parameters[key] = value
|
||||
}
|
||||
|
||||
if (index !== -1 && index !== string.length) {
|
||||
throw new TypeError('invalid parameter format')
|
||||
}
|
||||
|
||||
return obj
|
||||
}
|
||||
|
||||
/**
|
||||
* Get content-type from req/res objects.
|
||||
*
|
||||
* @param {object}
|
||||
* @return {Object}
|
||||
* @private
|
||||
*/
|
||||
|
||||
function getcontenttype(obj) {
|
||||
if (typeof obj.getHeader === 'function') {
|
||||
// res-like
|
||||
return obj.getHeader('content-type')
|
||||
}
|
||||
|
||||
if (typeof obj.headers === 'object') {
|
||||
// req-like
|
||||
return obj.headers && obj.headers['content-type']
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Quote a string if necessary.
|
||||
*
|
||||
* @param {string} val
|
||||
* @return {string}
|
||||
* @private
|
||||
*/
|
||||
|
||||
function qstring(val) {
|
||||
var str = String(val)
|
||||
|
||||
// no need to quote tokens
|
||||
if (tokenRegExp.test(str)) {
|
||||
return str
|
||||
}
|
||||
|
||||
if (str.length > 0 && !textRegExp.test(str)) {
|
||||
throw new TypeError('invalid parameter value')
|
||||
}
|
||||
|
||||
return '"' + str.replace(quoteRegExp, '\\$1') + '"'
|
||||
}
|
||||
|
||||
/**
|
||||
* Class to represent a content type.
|
||||
* @private
|
||||
*/
|
||||
function ContentType(type) {
|
||||
this.parameters = Object.create(null)
|
||||
this.type = type
|
||||
}
|
64
node_modules/express/node_modules/content-type/package.json
generated
vendored
Normal file
64
node_modules/express/node_modules/content-type/package.json
generated
vendored
Normal file
@ -0,0 +1,64 @@
|
||||
{
|
||||
"name": "content-type",
|
||||
"description": "Create and parse HTTP Content-Type header",
|
||||
"version": "1.0.1",
|
||||
"author": {
|
||||
"name": "Douglas Christopher Wilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
"license": "MIT",
|
||||
"keywords": [
|
||||
"content-type",
|
||||
"http",
|
||||
"req",
|
||||
"res",
|
||||
"rfc7231"
|
||||
],
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "https://github.com/jshttp/content-type"
|
||||
},
|
||||
"devDependencies": {
|
||||
"istanbul": "0.3.5",
|
||||
"mocha": "~1.21.5"
|
||||
},
|
||||
"files": [
|
||||
"LICENSE",
|
||||
"HISTORY.md",
|
||||
"README.md",
|
||||
"index.js"
|
||||
],
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "mocha --reporter spec --check-leaks --bail test/",
|
||||
"test-ci": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --check-leaks test/",
|
||||
"test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot --check-leaks test/"
|
||||
},
|
||||
"gitHead": "3aa58f9c5a358a3634b8601602177888b4a477d8",
|
||||
"bugs": {
|
||||
"url": "https://github.com/jshttp/content-type/issues"
|
||||
},
|
||||
"homepage": "https://github.com/jshttp/content-type",
|
||||
"_id": "content-type@1.0.1",
|
||||
"_shasum": "a19d2247327dc038050ce622b7a154ec59c5e600",
|
||||
"_from": "content-type@~1.0.1",
|
||||
"_npmVersion": "1.4.28",
|
||||
"_npmUser": {
|
||||
"name": "dougwilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
"maintainers": [
|
||||
{
|
||||
"name": "dougwilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
}
|
||||
],
|
||||
"dist": {
|
||||
"shasum": "a19d2247327dc038050ce622b7a154ec59c5e600",
|
||||
"tarball": "http://registry.npmjs.org/content-type/-/content-type-1.0.1.tgz"
|
||||
},
|
||||
"directories": {},
|
||||
"_resolved": "https://registry.npmjs.org/content-type/-/content-type-1.0.1.tgz"
|
||||
}
|
4
node_modules/express/node_modules/cookie-signature/.npmignore
generated
vendored
Normal file
4
node_modules/express/node_modules/cookie-signature/.npmignore
generated
vendored
Normal file
@ -0,0 +1,4 @@
|
||||
support
|
||||
test
|
||||
examples
|
||||
*.sock
|
38
node_modules/express/node_modules/cookie-signature/History.md
generated
vendored
Normal file
38
node_modules/express/node_modules/cookie-signature/History.md
generated
vendored
Normal file
@ -0,0 +1,38 @@
|
||||
1.0.6 / 2015-02-03
|
||||
==================
|
||||
|
||||
* use `npm test` instead of `make test` to run tests
|
||||
* clearer assertion messages when checking input
|
||||
|
||||
|
||||
1.0.5 / 2014-09-05
|
||||
==================
|
||||
|
||||
* add license to package.json
|
||||
|
||||
1.0.4 / 2014-06-25
|
||||
==================
|
||||
|
||||
* corrected avoidance of timing attacks (thanks @tenbits!)
|
||||
|
||||
1.0.3 / 2014-01-28
|
||||
==================
|
||||
|
||||
* [incorrect] fix for timing attacks
|
||||
|
||||
1.0.2 / 2014-01-28
|
||||
==================
|
||||
|
||||
* fix missing repository warning
|
||||
* fix typo in test
|
||||
|
||||
1.0.1 / 2013-04-15
|
||||
==================
|
||||
|
||||
* Revert "Changed underlying HMAC algo. to sha512."
|
||||
* Revert "Fix for timing attacks on MAC verification."
|
||||
|
||||
0.0.1 / 2010-01-03
|
||||
==================
|
||||
|
||||
* Initial release
|
42
node_modules/express/node_modules/cookie-signature/Readme.md
generated
vendored
Normal file
42
node_modules/express/node_modules/cookie-signature/Readme.md
generated
vendored
Normal file
@ -0,0 +1,42 @@
|
||||
|
||||
# cookie-signature
|
||||
|
||||
Sign and unsign cookies.
|
||||
|
||||
## Example
|
||||
|
||||
```js
|
||||
var cookie = require('cookie-signature');
|
||||
|
||||
var val = cookie.sign('hello', 'tobiiscool');
|
||||
val.should.equal('hello.DGDUkGlIkCzPz+C0B064FNgHdEjox7ch8tOBGslZ5QI');
|
||||
|
||||
var val = cookie.sign('hello', 'tobiiscool');
|
||||
cookie.unsign(val, 'tobiiscool').should.equal('hello');
|
||||
cookie.unsign(val, 'luna').should.be.false;
|
||||
```
|
||||
|
||||
## License
|
||||
|
||||
(The MIT License)
|
||||
|
||||
Copyright (c) 2012 LearnBoost <tj@learnboost.com>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of this software and associated documentation files (the
|
||||
'Software'), to deal in the Software without restriction, including
|
||||
without limitation the rights to use, copy, modify, merge, publish,
|
||||
distribute, sublicense, and/or sell copies of the Software, and to
|
||||
permit persons to whom the Software is furnished to do so, subject to
|
||||
the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
|
||||
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
|
||||
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
|
||||
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
|
||||
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
51
node_modules/express/node_modules/cookie-signature/index.js
generated
vendored
Normal file
51
node_modules/express/node_modules/cookie-signature/index.js
generated
vendored
Normal file
@ -0,0 +1,51 @@
|
||||
/**
|
||||
* Module dependencies.
|
||||
*/
|
||||
|
||||
var crypto = require('crypto');
|
||||
|
||||
/**
|
||||
* Sign the given `val` with `secret`.
|
||||
*
|
||||
* @param {String} val
|
||||
* @param {String} secret
|
||||
* @return {String}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
exports.sign = function(val, secret){
|
||||
if ('string' != typeof val) throw new TypeError("Cookie value must be provided as a string.");
|
||||
if ('string' != typeof secret) throw new TypeError("Secret string must be provided.");
|
||||
return val + '.' + crypto
|
||||
.createHmac('sha256', secret)
|
||||
.update(val)
|
||||
.digest('base64')
|
||||
.replace(/\=+$/, '');
|
||||
};
|
||||
|
||||
/**
|
||||
* Unsign and decode the given `val` with `secret`,
|
||||
* returning `false` if the signature is invalid.
|
||||
*
|
||||
* @param {String} val
|
||||
* @param {String} secret
|
||||
* @return {String|Boolean}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
exports.unsign = function(val, secret){
|
||||
if ('string' != typeof val) throw new TypeError("Signed cookie string must be provided.");
|
||||
if ('string' != typeof secret) throw new TypeError("Secret string must be provided.");
|
||||
var str = val.slice(0, val.lastIndexOf('.'))
|
||||
, mac = exports.sign(str, secret);
|
||||
|
||||
return sha1(mac) == sha1(val) ? str : false;
|
||||
};
|
||||
|
||||
/**
|
||||
* Private
|
||||
*/
|
||||
|
||||
function sha1(str){
|
||||
return crypto.createHash('sha1').update(str).digest('hex');
|
||||
}
|
58
node_modules/express/node_modules/cookie-signature/package.json
generated
vendored
Normal file
58
node_modules/express/node_modules/cookie-signature/package.json
generated
vendored
Normal file
@ -0,0 +1,58 @@
|
||||
{
|
||||
"name": "cookie-signature",
|
||||
"version": "1.0.6",
|
||||
"description": "Sign and unsign cookies",
|
||||
"keywords": [
|
||||
"cookie",
|
||||
"sign",
|
||||
"unsign"
|
||||
],
|
||||
"author": {
|
||||
"name": "TJ Holowaychuk",
|
||||
"email": "tj@learnboost.com"
|
||||
},
|
||||
"license": "MIT",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "https://github.com/visionmedia/node-cookie-signature.git"
|
||||
},
|
||||
"dependencies": {},
|
||||
"devDependencies": {
|
||||
"mocha": "*",
|
||||
"should": "*"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "mocha --require should --reporter spec"
|
||||
},
|
||||
"main": "index",
|
||||
"gitHead": "391b56cf44d88c493491b7e3fc53208cfb976d2a",
|
||||
"bugs": {
|
||||
"url": "https://github.com/visionmedia/node-cookie-signature/issues"
|
||||
},
|
||||
"homepage": "https://github.com/visionmedia/node-cookie-signature",
|
||||
"_id": "cookie-signature@1.0.6",
|
||||
"_shasum": "e303a882b342cc3ee8ca513a79999734dab3ae2c",
|
||||
"_from": "cookie-signature@1.0.6",
|
||||
"_npmVersion": "2.3.0",
|
||||
"_nodeVersion": "0.10.36",
|
||||
"_npmUser": {
|
||||
"name": "natevw",
|
||||
"email": "natevw@yahoo.com"
|
||||
},
|
||||
"maintainers": [
|
||||
{
|
||||
"name": "tjholowaychuk",
|
||||
"email": "tj@vision-media.ca"
|
||||
},
|
||||
{
|
||||
"name": "natevw",
|
||||
"email": "natevw@yahoo.com"
|
||||
}
|
||||
],
|
||||
"dist": {
|
||||
"shasum": "e303a882b342cc3ee8ca513a79999734dab3ae2c",
|
||||
"tarball": "http://registry.npmjs.org/cookie-signature/-/cookie-signature-1.0.6.tgz"
|
||||
},
|
||||
"directories": {},
|
||||
"_resolved": "https://registry.npmjs.org/cookie-signature/-/cookie-signature-1.0.6.tgz"
|
||||
}
|
72
node_modules/express/node_modules/cookie/HISTORY.md
generated
vendored
Normal file
72
node_modules/express/node_modules/cookie/HISTORY.md
generated
vendored
Normal file
@ -0,0 +1,72 @@
|
||||
0.1.5 / 2015-09-17
|
||||
==================
|
||||
|
||||
* Fix regression when setting empty cookie value
|
||||
- Ease the new restriction, which is just basic header-level validation
|
||||
* Fix typo in invalid value errors
|
||||
|
||||
0.1.4 / 2015-09-17
|
||||
==================
|
||||
|
||||
* Throw better error for invalid argument to parse
|
||||
* Throw on invalid values provided to `serialize`
|
||||
- Ensures the resulting string is a valid HTTP header value
|
||||
|
||||
0.1.3 / 2015-05-19
|
||||
==================
|
||||
|
||||
* Reduce the scope of try-catch deopt
|
||||
* Remove argument reassignments
|
||||
|
||||
0.1.2 / 2014-04-16
|
||||
==================
|
||||
|
||||
* Remove unnecessary files from npm package
|
||||
|
||||
0.1.1 / 2014-02-23
|
||||
==================
|
||||
|
||||
* Fix bad parse when cookie value contained a comma
|
||||
* Fix support for `maxAge` of `0`
|
||||
|
||||
0.1.0 / 2013-05-01
|
||||
==================
|
||||
|
||||
* Add `decode` option
|
||||
* Add `encode` option
|
||||
|
||||
0.0.6 / 2013-04-08
|
||||
==================
|
||||
|
||||
* Ignore cookie parts missing `=`
|
||||
|
||||
0.0.5 / 2012-10-29
|
||||
==================
|
||||
|
||||
* Return raw cookie value if value unescape errors
|
||||
|
||||
0.0.4 / 2012-06-21
|
||||
==================
|
||||
|
||||
* Use encode/decodeURIComponent for cookie encoding/decoding
|
||||
- Improve server/client interoperability
|
||||
|
||||
0.0.3 / 2012-06-06
|
||||
==================
|
||||
|
||||
* Only escape special characters per the cookie RFC
|
||||
|
||||
0.0.2 / 2012-06-01
|
||||
==================
|
||||
|
||||
* Fix `maxAge` option to not throw error
|
||||
|
||||
0.0.1 / 2012-05-28
|
||||
==================
|
||||
|
||||
* Add more tests
|
||||
|
||||
0.0.0 / 2012-05-28
|
||||
==================
|
||||
|
||||
* Initial release
|
24
node_modules/express/node_modules/cookie/LICENSE
generated
vendored
Normal file
24
node_modules/express/node_modules/cookie/LICENSE
generated
vendored
Normal file
@ -0,0 +1,24 @@
|
||||
(The MIT License)
|
||||
|
||||
Copyright (c) 2012-2014 Roman Shtylman <shtylman@gmail.com>
|
||||
Copyright (c) 2015 Douglas Christopher Wilson <doug@somethingdoug.com>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of this software and associated documentation files (the
|
||||
'Software'), to deal in the Software without restriction, including
|
||||
without limitation the rights to use, copy, modify, merge, publish,
|
||||
distribute, sublicense, and/or sell copies of the Software, and to
|
||||
permit persons to whom the Software is furnished to do so, subject to
|
||||
the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
|
||||
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
|
||||
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
|
||||
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
|
||||
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
|
64
node_modules/express/node_modules/cookie/README.md
generated
vendored
Normal file
64
node_modules/express/node_modules/cookie/README.md
generated
vendored
Normal file
@ -0,0 +1,64 @@
|
||||
# cookie
|
||||
|
||||
[![NPM Version][npm-image]][npm-url]
|
||||
[![NPM Downloads][downloads-image]][downloads-url]
|
||||
[![Node.js Version][node-version-image]][node-version-url]
|
||||
[![Build Status][travis-image]][travis-url]
|
||||
[![Test Coverage][coveralls-image]][coveralls-url]
|
||||
|
||||
cookie is a basic cookie parser and serializer. It doesn't make assumptions about how you are going to deal with your cookies. It basically just provides a way to read and write the HTTP cookie headers.
|
||||
|
||||
See [RFC6265](http://tools.ietf.org/html/rfc6265) for details about the http header for cookies.
|
||||
|
||||
## how?
|
||||
|
||||
```
|
||||
npm install cookie
|
||||
```
|
||||
|
||||
```javascript
|
||||
var cookie = require('cookie');
|
||||
|
||||
var hdr = cookie.serialize('foo', 'bar');
|
||||
// hdr = 'foo=bar';
|
||||
|
||||
var cookies = cookie.parse('foo=bar; cat=meow; dog=ruff');
|
||||
// cookies = { foo: 'bar', cat: 'meow', dog: 'ruff' };
|
||||
```
|
||||
|
||||
## more
|
||||
|
||||
The serialize function takes a third parameter, an object, to set cookie options. See the RFC for valid values.
|
||||
|
||||
### path
|
||||
> cookie path
|
||||
|
||||
### expires
|
||||
> absolute expiration date for the cookie (Date object)
|
||||
|
||||
### maxAge
|
||||
> relative max age of the cookie from when the client receives it (seconds)
|
||||
|
||||
### domain
|
||||
> domain for the cookie
|
||||
|
||||
### secure
|
||||
> true or false
|
||||
|
||||
### httpOnly
|
||||
> true or false
|
||||
|
||||
## License
|
||||
|
||||
[MIT](LICENSE)
|
||||
|
||||
[npm-image]: https://img.shields.io/npm/v/cookie.svg
|
||||
[npm-url]: https://npmjs.org/package/cookie
|
||||
[node-version-image]: https://img.shields.io/node/v/cookie.svg
|
||||
[node-version-url]: http://nodejs.org/download/
|
||||
[travis-image]: https://img.shields.io/travis/jshttp/cookie/master.svg
|
||||
[travis-url]: https://travis-ci.org/jshttp/cookie
|
||||
[coveralls-image]: https://img.shields.io/coveralls/jshttp/cookie/master.svg
|
||||
[coveralls-url]: https://coveralls.io/r/jshttp/cookie?branch=master
|
||||
[downloads-image]: https://img.shields.io/npm/dm/cookie.svg
|
||||
[downloads-url]: https://npmjs.org/package/cookie
|
156
node_modules/express/node_modules/cookie/index.js
generated
vendored
Normal file
156
node_modules/express/node_modules/cookie/index.js
generated
vendored
Normal file
@ -0,0 +1,156 @@
|
||||
/*!
|
||||
* cookie
|
||||
* Copyright(c) 2012-2014 Roman Shtylman
|
||||
* Copyright(c) 2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
/**
|
||||
* Module exports.
|
||||
* @public
|
||||
*/
|
||||
|
||||
exports.parse = parse;
|
||||
exports.serialize = serialize;
|
||||
|
||||
/**
|
||||
* Module variables.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var decode = decodeURIComponent;
|
||||
var encode = encodeURIComponent;
|
||||
|
||||
/**
|
||||
* RegExp to match field-content in RFC 7230 sec 3.2
|
||||
*
|
||||
* field-content = field-vchar [ 1*( SP / HTAB ) field-vchar ]
|
||||
* field-vchar = VCHAR / obs-text
|
||||
* obs-text = %x80-FF
|
||||
*/
|
||||
|
||||
var fieldContentRegExp = /^[\u0009\u0020-\u007e\u0080-\u00ff]+$/;
|
||||
|
||||
/**
|
||||
* Parse a cookie header.
|
||||
*
|
||||
* Parse the given cookie header string into an object
|
||||
* The object has the various cookies as keys(names) => values
|
||||
*
|
||||
* @param {string} str
|
||||
* @param {object} [options]
|
||||
* @return {object}
|
||||
* @public
|
||||
*/
|
||||
|
||||
function parse(str, options) {
|
||||
if (typeof str !== 'string') {
|
||||
throw new TypeError('argument str must be a string');
|
||||
}
|
||||
|
||||
var obj = {}
|
||||
var opt = options || {};
|
||||
var pairs = str.split(/; */);
|
||||
var dec = opt.decode || decode;
|
||||
|
||||
pairs.forEach(function(pair) {
|
||||
var eq_idx = pair.indexOf('=')
|
||||
|
||||
// skip things that don't look like key=value
|
||||
if (eq_idx < 0) {
|
||||
return;
|
||||
}
|
||||
|
||||
var key = pair.substr(0, eq_idx).trim()
|
||||
var val = pair.substr(++eq_idx, pair.length).trim();
|
||||
|
||||
// quoted values
|
||||
if ('"' == val[0]) {
|
||||
val = val.slice(1, -1);
|
||||
}
|
||||
|
||||
// only assign once
|
||||
if (undefined == obj[key]) {
|
||||
obj[key] = tryDecode(val, dec);
|
||||
}
|
||||
});
|
||||
|
||||
return obj;
|
||||
}
|
||||
|
||||
/**
|
||||
* Serialize data into a cookie header.
|
||||
*
|
||||
* Serialize the a name value pair into a cookie string suitable for
|
||||
* http headers. An optional options object specified cookie parameters.
|
||||
*
|
||||
* serialize('foo', 'bar', { httpOnly: true })
|
||||
* => "foo=bar; httpOnly"
|
||||
*
|
||||
* @param {string} name
|
||||
* @param {string} val
|
||||
* @param {object} [options]
|
||||
* @return {string}
|
||||
* @public
|
||||
*/
|
||||
|
||||
function serialize(name, val, options) {
|
||||
var opt = options || {};
|
||||
var enc = opt.encode || encode;
|
||||
|
||||
if (!fieldContentRegExp.test(name)) {
|
||||
throw new TypeError('argument name is invalid');
|
||||
}
|
||||
|
||||
var value = enc(val);
|
||||
|
||||
if (value && !fieldContentRegExp.test(value)) {
|
||||
throw new TypeError('argument val is invalid');
|
||||
}
|
||||
|
||||
var pairs = [name + '=' + value];
|
||||
|
||||
if (null != opt.maxAge) {
|
||||
var maxAge = opt.maxAge - 0;
|
||||
if (isNaN(maxAge)) throw new Error('maxAge should be a Number');
|
||||
pairs.push('Max-Age=' + maxAge);
|
||||
}
|
||||
|
||||
if (opt.domain) {
|
||||
if (!fieldContentRegExp.test(opt.domain)) {
|
||||
throw new TypeError('option domain is invalid');
|
||||
}
|
||||
|
||||
pairs.push('Domain=' + opt.domain);
|
||||
}
|
||||
|
||||
if (opt.path) {
|
||||
if (!fieldContentRegExp.test(opt.path)) {
|
||||
throw new TypeError('option path is invalid');
|
||||
}
|
||||
|
||||
pairs.push('Path=' + opt.path);
|
||||
}
|
||||
|
||||
if (opt.expires) pairs.push('Expires=' + opt.expires.toUTCString());
|
||||
if (opt.httpOnly) pairs.push('HttpOnly');
|
||||
if (opt.secure) pairs.push('Secure');
|
||||
|
||||
return pairs.join('; ');
|
||||
}
|
||||
|
||||
/**
|
||||
* Try decoding a string using a decoding function.
|
||||
*
|
||||
* @param {string} str
|
||||
* @param {function} decode
|
||||
* @private
|
||||
*/
|
||||
|
||||
function tryDecode(str, decode) {
|
||||
try {
|
||||
return decode(str);
|
||||
} catch (e) {
|
||||
return str;
|
||||
}
|
||||
}
|
75
node_modules/express/node_modules/cookie/package.json
generated
vendored
Normal file
75
node_modules/express/node_modules/cookie/package.json
generated
vendored
Normal file
@ -0,0 +1,75 @@
|
||||
{
|
||||
"name": "cookie",
|
||||
"description": "cookie parsing and serialization",
|
||||
"version": "0.1.5",
|
||||
"author": {
|
||||
"name": "Roman Shtylman",
|
||||
"email": "shtylman@gmail.com"
|
||||
},
|
||||
"contributors": [
|
||||
{
|
||||
"name": "Douglas Christopher Wilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
}
|
||||
],
|
||||
"license": "MIT",
|
||||
"keywords": [
|
||||
"cookie",
|
||||
"cookies"
|
||||
],
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "https://github.com/jshttp/cookie"
|
||||
},
|
||||
"devDependencies": {
|
||||
"istanbul": "0.3.20",
|
||||
"mocha": "1.21.5"
|
||||
},
|
||||
"files": [
|
||||
"HISTORY.md",
|
||||
"LICENSE",
|
||||
"README.md",
|
||||
"index.js"
|
||||
],
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
},
|
||||
"scripts": {
|
||||
"test": "mocha --reporter spec --bail --check-leaks test/",
|
||||
"test-ci": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --check-leaks test/",
|
||||
"test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot --check-leaks test/"
|
||||
},
|
||||
"gitHead": "0dfc4876575cef2609cdc1082fccf832743822c2",
|
||||
"bugs": {
|
||||
"url": "https://github.com/jshttp/cookie/issues"
|
||||
},
|
||||
"homepage": "https://github.com/jshttp/cookie",
|
||||
"_id": "cookie@0.1.5",
|
||||
"_shasum": "6ab9948a4b1ae21952cd2588530a4722d4044d7c",
|
||||
"_from": "cookie@0.1.5",
|
||||
"_npmVersion": "1.4.28",
|
||||
"_npmUser": {
|
||||
"name": "dougwilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
"maintainers": [
|
||||
{
|
||||
"name": "defunctzombie",
|
||||
"email": "shtylman@gmail.com"
|
||||
},
|
||||
{
|
||||
"name": "dougwilson",
|
||||
"email": "doug@somethingdoug.com"
|
||||
},
|
||||
{
|
||||
"name": "jongleberry",
|
||||
"email": "jonathanrichardong@gmail.com"
|
||||
}
|
||||
],
|
||||
"dist": {
|
||||
"shasum": "6ab9948a4b1ae21952cd2588530a4722d4044d7c",
|
||||
"tarball": "http://registry.npmjs.org/cookie/-/cookie-0.1.5.tgz"
|
||||
},
|
||||
"directories": {},
|
||||
"_resolved": "https://registry.npmjs.org/cookie/-/cookie-0.1.5.tgz"
|
||||
}
|
3
node_modules/express/node_modules/debug/.jshintrc
generated
vendored
Normal file
3
node_modules/express/node_modules/debug/.jshintrc
generated
vendored
Normal file
@ -0,0 +1,3 @@
|
||||
{
|
||||
"laxbreak": true
|
||||
}
|
6
node_modules/express/node_modules/debug/.npmignore
generated
vendored
Normal file
6
node_modules/express/node_modules/debug/.npmignore
generated
vendored
Normal file
@ -0,0 +1,6 @@
|
||||
support
|
||||
test
|
||||
examples
|
||||
example
|
||||
*.sock
|
||||
dist
|
195
node_modules/express/node_modules/debug/History.md
generated
vendored
Normal file
195
node_modules/express/node_modules/debug/History.md
generated
vendored
Normal file
@ -0,0 +1,195 @@
|
||||
|
||||
2.2.0 / 2015-05-09
|
||||
==================
|
||||
|
||||
* package: update "ms" to v0.7.1 (#202, @dougwilson)
|
||||
* README: add logging to file example (#193, @DanielOchoa)
|
||||
* README: fixed a typo (#191, @amir-s)
|
||||
* browser: expose `storage` (#190, @stephenmathieson)
|
||||
* Makefile: add a `distclean` target (#189, @stephenmathieson)
|
||||
|
||||
2.1.3 / 2015-03-13
|
||||
==================
|
||||
|
||||
* Updated stdout/stderr example (#186)
|
||||
* Updated example/stdout.js to match debug current behaviour
|
||||
* Renamed example/stderr.js to stdout.js
|
||||
* Update Readme.md (#184)
|
||||
* replace high intensity foreground color for bold (#182, #183)
|
||||
|
||||
2.1.2 / 2015-03-01
|
||||
==================
|
||||
|
||||
* dist: recompile
|
||||
* update "ms" to v0.7.0
|
||||
* package: update "browserify" to v9.0.3
|
||||
* component: fix "ms.js" repo location
|
||||
* changed bower package name
|
||||
* updated documentation about using debug in a browser
|
||||
* fix: security error on safari (#167, #168, @yields)
|
||||
|
||||
2.1.1 / 2014-12-29
|
||||
==================
|
||||
|
||||
* browser: use `typeof` to check for `console` existence
|
||||
* browser: check for `console.log` truthiness (fix IE 8/9)
|
||||
* browser: add support for Chrome apps
|
||||
* Readme: added Windows usage remarks
|
||||
* Add `bower.json` to properly support bower install
|
||||
|
||||
2.1.0 / 2014-10-15
|
||||
==================
|
||||
|
||||
* node: implement `DEBUG_FD` env variable support
|
||||
* package: update "browserify" to v6.1.0
|
||||
* package: add "license" field to package.json (#135, @panuhorsmalahti)
|
||||
|
||||
2.0.0 / 2014-09-01
|
||||
==================
|
||||
|
||||
* package: update "browserify" to v5.11.0
|
||||
* node: use stderr rather than stdout for logging (#29, @stephenmathieson)
|
||||
|
||||
1.0.4 / 2014-07-15
|
||||
==================
|
||||
|
||||
* dist: recompile
|
||||
* example: remove `console.info()` log usage
|
||||
* example: add "Content-Type" UTF-8 header to browser example
|
||||
* browser: place %c marker after the space character
|
||||
* browser: reset the "content" color via `color: inherit`
|
||||
* browser: add colors support for Firefox >= v31
|
||||
* debug: prefer an instance `log()` function over the global one (#119)
|
||||
* Readme: update documentation about styled console logs for FF v31 (#116, @wryk)
|
||||
|
||||
1.0.3 / 2014-07-09
|
||||
==================
|
||||
|
||||
* Add support for multiple wildcards in namespaces (#122, @seegno)
|
||||
* browser: fix lint
|
||||
|
||||
1.0.2 / 2014-06-10
|
||||
==================
|
||||
|
||||
* browser: update color palette (#113, @gscottolson)
|
||||
* common: make console logging function configurable (#108, @timoxley)
|
||||
* node: fix %o colors on old node <= 0.8.x
|
||||
* Makefile: find node path using shell/which (#109, @timoxley)
|
||||
|
||||
1.0.1 / 2014-06-06
|
||||
==================
|
||||
|
||||
* browser: use `removeItem()` to clear localStorage
|
||||
* browser, node: don't set DEBUG if namespaces is undefined (#107, @leedm777)
|
||||
* package: add "contributors" section
|
||||
* node: fix comment typo
|
||||
* README: list authors
|
||||
|
||||
1.0.0 / 2014-06-04
|
||||
==================
|
||||
|
||||
* make ms diff be global, not be scope
|
||||
* debug: ignore empty strings in enable()
|
||||
* node: make DEBUG_COLORS able to disable coloring
|
||||
* *: export the `colors` array
|
||||
* npmignore: don't publish the `dist` dir
|
||||
* Makefile: refactor to use browserify
|
||||
* package: add "browserify" as a dev dependency
|
||||
* Readme: add Web Inspector Colors section
|
||||
* node: reset terminal color for the debug content
|
||||
* node: map "%o" to `util.inspect()`
|
||||
* browser: map "%j" to `JSON.stringify()`
|
||||
* debug: add custom "formatters"
|
||||
* debug: use "ms" module for humanizing the diff
|
||||
* Readme: add "bash" syntax highlighting
|
||||
* browser: add Firebug color support
|
||||
* browser: add colors for WebKit browsers
|
||||
* node: apply log to `console`
|
||||
* rewrite: abstract common logic for Node & browsers
|
||||
* add .jshintrc file
|
||||
|
||||
0.8.1 / 2014-04-14
|
||||
==================
|
||||
|
||||
* package: re-add the "component" section
|
||||
|
||||
0.8.0 / 2014-03-30
|
||||
==================
|
||||
|
||||
* add `enable()` method for nodejs. Closes #27
|
||||
* change from stderr to stdout
|
||||
* remove unnecessary index.js file
|
||||
|
||||
0.7.4 / 2013-11-13
|
||||
==================
|
||||
|
||||
* remove "browserify" key from package.json (fixes something in browserify)
|
||||
|
||||
0.7.3 / 2013-10-30
|
||||
==================
|
||||
|
||||
* fix: catch localStorage security error when cookies are blocked (Chrome)
|
||||
* add debug(err) support. Closes #46
|
||||
* add .browser prop to package.json. Closes #42
|
||||
|
||||
0.7.2 / 2013-02-06
|
||||
==================
|
||||
|
||||
* fix package.json
|
||||
* fix: Mobile Safari (private mode) is broken with debug
|
||||
* fix: Use unicode to send escape character to shell instead of octal to work with strict mode javascript
|
||||
|
||||
0.7.1 / 2013-02-05
|
||||
==================
|
||||
|
||||
* add repository URL to package.json
|
||||
* add DEBUG_COLORED to force colored output
|
||||
* add browserify support
|
||||
* fix component. Closes #24
|
||||
|
||||
0.7.0 / 2012-05-04
|
||||
==================
|
||||
|
||||
* Added .component to package.json
|
||||
* Added debug.component.js build
|
||||
|
||||
0.6.0 / 2012-03-16
|
||||
==================
|
||||
|
||||
* Added support for "-" prefix in DEBUG [Vinay Pulim]
|
||||
* Added `.enabled` flag to the node version [TooTallNate]
|
||||
|
||||
0.5.0 / 2012-02-02
|
||||
==================
|
||||
|
||||
* Added: humanize diffs. Closes #8
|
||||
* Added `debug.disable()` to the CS variant
|
||||
* Removed padding. Closes #10
|
||||
* Fixed: persist client-side variant again. Closes #9
|
||||
|
||||
0.4.0 / 2012-02-01
|
||||
==================
|
||||
|
||||
* Added browser variant support for older browsers [TooTallNate]
|
||||
* Added `debug.enable('project:*')` to browser variant [TooTallNate]
|
||||
* Added padding to diff (moved it to the right)
|
||||
|
||||
0.3.0 / 2012-01-26
|
||||
==================
|
||||
|
||||
* Added millisecond diff when isatty, otherwise UTC string
|
||||
|
||||
0.2.0 / 2012-01-22
|
||||
==================
|
||||
|
||||
* Added wildcard support
|
||||
|
||||
0.1.0 / 2011-12-02
|
||||
==================
|
||||
|
||||
* Added: remove colors unless stderr isatty [TooTallNate]
|
||||
|
||||
0.0.1 / 2010-01-03
|
||||
==================
|
||||
|
||||
* Initial release
|
36
node_modules/express/node_modules/debug/Makefile
generated
vendored
Normal file
36
node_modules/express/node_modules/debug/Makefile
generated
vendored
Normal file
@ -0,0 +1,36 @@
|
||||
|
||||
# get Makefile directory name: http://stackoverflow.com/a/5982798/376773
|
||||
THIS_MAKEFILE_PATH:=$(word $(words $(MAKEFILE_LIST)),$(MAKEFILE_LIST))
|
||||
THIS_DIR:=$(shell cd $(dir $(THIS_MAKEFILE_PATH));pwd)
|
||||
|
||||
# BIN directory
|
||||
BIN := $(THIS_DIR)/node_modules/.bin
|
||||
|
||||
# applications
|
||||
NODE ?= $(shell which node)
|
||||
NPM ?= $(NODE) $(shell which npm)
|
||||
BROWSERIFY ?= $(NODE) $(BIN)/browserify
|
||||
|
||||
all: dist/debug.js
|
||||
|
||||
install: node_modules
|
||||
|
||||
clean:
|
||||
@rm -rf dist
|
||||
|
||||
dist:
|
||||
@mkdir -p $@
|
||||
|
||||
dist/debug.js: node_modules browser.js debug.js dist
|
||||
@$(BROWSERIFY) \
|
||||
--standalone debug \
|
||||
. > $@
|
||||
|
||||
distclean: clean
|
||||
@rm -rf node_modules
|
||||
|
||||
node_modules: package.json
|
||||
@NODE_ENV= $(NPM) install
|
||||
@touch node_modules
|
||||
|
||||
.PHONY: all install clean distclean
|
188
node_modules/express/node_modules/debug/Readme.md
generated
vendored
Normal file
188
node_modules/express/node_modules/debug/Readme.md
generated
vendored
Normal file
@ -0,0 +1,188 @@
|
||||
# debug
|
||||
|
||||
tiny node.js debugging utility modelled after node core's debugging technique.
|
||||
|
||||
## Installation
|
||||
|
||||
```bash
|
||||
$ npm install debug
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
With `debug` you simply invoke the exported function to generate your debug function, passing it a name which will determine if a noop function is returned, or a decorated `console.error`, so all of the `console` format string goodies you're used to work fine. A unique color is selected per-function for visibility.
|
||||
|
||||
Example _app.js_:
|
||||
|
||||
```js
|
||||
var debug = require('debug')('http')
|
||||
, http = require('http')
|
||||
, name = 'My App';
|
||||
|
||||
// fake app
|
||||
|
||||
debug('booting %s', name);
|
||||
|
||||
http.createServer(function(req, res){
|
||||
debug(req.method + ' ' + req.url);
|
||||
res.end('hello\n');
|
||||
}).listen(3000, function(){
|
||||
debug('listening');
|
||||
});
|
||||
|
||||
// fake worker of some kind
|
||||
|
||||
require('./worker');
|
||||
```
|
||||
|
||||
Example _worker.js_:
|
||||
|
||||
```js
|
||||
var debug = require('debug')('worker');
|
||||
|
||||
setInterval(function(){
|
||||
debug('doing some work');
|
||||
}, 1000);
|
||||
```
|
||||
|
||||
The __DEBUG__ environment variable is then used to enable these based on space or comma-delimited names. Here are some examples:
|
||||
|
||||

|
||||
|
||||

|
||||
|
||||
#### Windows note
|
||||
|
||||
On Windows the environment variable is set using the `set` command.
|
||||
|
||||
```cmd
|
||||
set DEBUG=*,-not_this
|
||||
```
|
||||
|
||||
Then, run the program to be debugged as usual.
|
||||
|
||||
## Millisecond diff
|
||||
|
||||
When actively developing an application it can be useful to see when the time spent between one `debug()` call and the next. Suppose for example you invoke `debug()` before requesting a resource, and after as well, the "+NNNms" will show you how much time was spent between calls.
|
||||
|
||||

|
||||
|
||||
When stdout is not a TTY, `Date#toUTCString()` is used, making it more useful for logging the debug information as shown below:
|
||||
|
||||

|
||||
|
||||
## Conventions
|
||||
|
||||
If you're using this in one or more of your libraries, you _should_ use the name of your library so that developers may toggle debugging as desired without guessing names. If you have more than one debuggers you _should_ prefix them with your library name and use ":" to separate features. For example "bodyParser" from Connect would then be "connect:bodyParser".
|
||||
|
||||
## Wildcards
|
||||
|
||||
The `*` character may be used as a wildcard. Suppose for example your library has debuggers named "connect:bodyParser", "connect:compress", "connect:session", instead of listing all three with `DEBUG=connect:bodyParser,connect:compress,connect:session`, you may simply do `DEBUG=connect:*`, or to run everything using this module simply use `DEBUG=*`.
|
||||
|
||||
You can also exclude specific debuggers by prefixing them with a "-" character. For example, `DEBUG=*,-connect:*` would include all debuggers except those starting with "connect:".
|
||||
|
||||
## Browser support
|
||||
|
||||
Debug works in the browser as well, currently persisted by `localStorage`. Consider the situation shown below where you have `worker:a` and `worker:b`, and wish to debug both. Somewhere in the code on your page, include:
|
||||
|
||||
```js
|
||||
window.myDebug = require("debug");
|
||||
```
|
||||
|
||||
("debug" is a global object in the browser so we give this object a different name.) When your page is open in the browser, type the following in the console:
|
||||
|
||||
```js
|
||||
myDebug.enable("worker:*")
|
||||
```
|
||||
|
||||
Refresh the page. Debug output will continue to be sent to the console until it is disabled by typing `myDebug.disable()` in the console.
|
||||
|
||||
```js
|
||||
a = debug('worker:a');
|
||||
b = debug('worker:b');
|
||||
|
||||
setInterval(function(){
|
||||
a('doing some work');
|
||||
}, 1000);
|
||||
|
||||
setInterval(function(){
|
||||
b('doing some work');
|
||||
}, 1200);
|
||||
```
|
||||
|
||||
#### Web Inspector Colors
|
||||
|
||||
Colors are also enabled on "Web Inspectors" that understand the `%c` formatting
|
||||
option. These are WebKit web inspectors, Firefox ([since version
|
||||
31](https://hacks.mozilla.org/2014/05/editable-box-model-multiple-selection-sublime-text-keys-much-more-firefox-developer-tools-episode-31/))
|
||||
and the Firebug plugin for Firefox (any version).
|
||||
|
||||
Colored output looks something like:
|
||||
|
||||

|
||||
|
||||
### stderr vs stdout
|
||||
|
||||
You can set an alternative logging method per-namespace by overriding the `log` method on a per-namespace or globally:
|
||||
|
||||
Example _stdout.js_:
|
||||
|
||||
```js
|
||||
var debug = require('debug');
|
||||
var error = debug('app:error');
|
||||
|
||||
// by default stderr is used
|
||||
error('goes to stderr!');
|
||||
|
||||
var log = debug('app:log');
|
||||
// set this namespace to log via console.log
|
||||
log.log = console.log.bind(console); // don't forget to bind to console!
|
||||
log('goes to stdout');
|
||||
error('still goes to stderr!');
|
||||
|
||||
// set all output to go via console.info
|
||||
// overrides all per-namespace log settings
|
||||
debug.log = console.info.bind(console);
|
||||
error('now goes to stdout via console.info');
|
||||
log('still goes to stdout, but via console.info now');
|
||||
```
|
||||
|
||||
### Save debug output to a file
|
||||
|
||||
You can save all debug statements to a file by piping them.
|
||||
|
||||
Example:
|
||||
|
||||
```bash
|
||||
$ DEBUG_FD=3 node your-app.js 3> whatever.log
|
||||
```
|
||||
|
||||
## Authors
|
||||
|
||||
- TJ Holowaychuk
|
||||
- Nathan Rajlich
|
||||
|
||||
## License
|
||||
|
||||
(The MIT License)
|
||||
|
||||
Copyright (c) 2014 TJ Holowaychuk <tj@vision-media.ca>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of this software and associated documentation files (the
|
||||
'Software'), to deal in the Software without restriction, including
|
||||
without limitation the rights to use, copy, modify, merge, publish,
|
||||
distribute, sublicense, and/or sell copies of the Software, and to
|
||||
permit persons to whom the Software is furnished to do so, subject to
|
||||
the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
|
||||
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
|
||||
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
|
||||
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
|
||||
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
28
node_modules/express/node_modules/debug/bower.json
generated
vendored
Normal file
28
node_modules/express/node_modules/debug/bower.json
generated
vendored
Normal file
@ -0,0 +1,28 @@
|
||||
{
|
||||
"name": "visionmedia-debug",
|
||||
"main": "dist/debug.js",
|
||||
"version": "2.2.0",
|
||||
"homepage": "https://github.com/visionmedia/debug",
|
||||
"authors": [
|
||||
"TJ Holowaychuk <tj@vision-media.ca>"
|
||||
],
|
||||
"description": "visionmedia-debug",
|
||||
"moduleType": [
|
||||
"amd",
|
||||
"es6",
|
||||
"globals",
|
||||
"node"
|
||||
],
|
||||
"keywords": [
|
||||
"visionmedia",
|
||||
"debug"
|
||||
],
|
||||
"license": "MIT",
|
||||
"ignore": [
|
||||
"**/.*",
|
||||
"node_modules",
|
||||
"bower_components",
|
||||
"test",
|
||||
"tests"
|
||||
]
|
||||
}
|
168
node_modules/express/node_modules/debug/browser.js
generated
vendored
Normal file
168
node_modules/express/node_modules/debug/browser.js
generated
vendored
Normal file
@ -0,0 +1,168 @@
|
||||
|
||||
/**
|
||||
* This is the web browser implementation of `debug()`.
|
||||
*
|
||||
* Expose `debug()` as the module.
|
||||
*/
|
||||
|
||||
exports = module.exports = require('./debug');
|
||||
exports.log = log;
|
||||
exports.formatArgs = formatArgs;
|
||||
exports.save = save;
|
||||
exports.load = load;
|
||||
exports.useColors = useColors;
|
||||
exports.storage = 'undefined' != typeof chrome
|
||||
&& 'undefined' != typeof chrome.storage
|
||||
? chrome.storage.local
|
||||
: localstorage();
|
||||
|
||||
/**
|
||||
* Colors.
|
||||
*/
|
||||
|
||||
exports.colors = [
|
||||
'lightseagreen',
|
||||
'forestgreen',
|
||||
'goldenrod',
|
||||
'dodgerblue',
|
||||
'darkorchid',
|
||||
'crimson'
|
||||
];
|
||||
|
||||
/**
|
||||
* Currently only WebKit-based Web Inspectors, Firefox >= v31,
|
||||
* and the Firebug extension (any Firefox version) are known
|
||||
* to support "%c" CSS customizations.
|
||||
*
|
||||
* TODO: add a `localStorage` variable to explicitly enable/disable colors
|
||||
*/
|
||||
|
||||
function useColors() {
|
||||
// is webkit? http://stackoverflow.com/a/16459606/376773
|
||||
return ('WebkitAppearance' in document.documentElement.style) ||
|
||||
// is firebug? http://stackoverflow.com/a/398120/376773
|
||||
(window.console && (console.firebug || (console.exception && console.table))) ||
|
||||
// is firefox >= v31?
|
||||
// https://developer.mozilla.org/en-US/docs/Tools/Web_Console#Styling_messages
|
||||
(navigator.userAgent.toLowerCase().match(/firefox\/(\d+)/) && parseInt(RegExp.$1, 10) >= 31);
|
||||
}
|
||||
|
||||
/**
|
||||
* Map %j to `JSON.stringify()`, since no Web Inspectors do that by default.
|
||||
*/
|
||||
|
||||
exports.formatters.j = function(v) {
|
||||
return JSON.stringify(v);
|
||||
};
|
||||
|
||||
|
||||
/**
|
||||
* Colorize log arguments if enabled.
|
||||
*
|
||||
* @api public
|
||||
*/
|
||||
|
||||
function formatArgs() {
|
||||
var args = arguments;
|
||||
var useColors = this.useColors;
|
||||
|
||||
args[0] = (useColors ? '%c' : '')
|
||||
+ this.namespace
|
||||
+ (useColors ? ' %c' : ' ')
|
||||
+ args[0]
|
||||
+ (useColors ? '%c ' : ' ')
|
||||
+ '+' + exports.humanize(this.diff);
|
||||
|
||||
if (!useColors) return args;
|
||||
|
||||
var c = 'color: ' + this.color;
|
||||
args = [args[0], c, 'color: inherit'].concat(Array.prototype.slice.call(args, 1));
|
||||
|
||||
// the final "%c" is somewhat tricky, because there could be other
|
||||
// arguments passed either before or after the %c, so we need to
|
||||
// figure out the correct index to insert the CSS into
|
||||
var index = 0;
|
||||
var lastC = 0;
|
||||
args[0].replace(/%[a-z%]/g, function(match) {
|
||||
if ('%%' === match) return;
|
||||
index++;
|
||||
if ('%c' === match) {
|
||||
// we only are interested in the *last* %c
|
||||
// (the user may have provided their own)
|
||||
lastC = index;
|
||||
}
|
||||
});
|
||||
|
||||
args.splice(lastC, 0, c);
|
||||
return args;
|
||||
}
|
||||
|
||||
/**
|
||||
* Invokes `console.log()` when available.
|
||||
* No-op when `console.log` is not a "function".
|
||||
*
|
||||
* @api public
|
||||
*/
|
||||
|
||||
function log() {
|
||||
// this hackery is required for IE8/9, where
|
||||
// the `console.log` function doesn't have 'apply'
|
||||
return 'object' === typeof console
|
||||
&& console.log
|
||||
&& Function.prototype.apply.call(console.log, console, arguments);
|
||||
}
|
||||
|
||||
/**
|
||||
* Save `namespaces`.
|
||||
*
|
||||
* @param {String} namespaces
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function save(namespaces) {
|
||||
try {
|
||||
if (null == namespaces) {
|
||||
exports.storage.removeItem('debug');
|
||||
} else {
|
||||
exports.storage.debug = namespaces;
|
||||
}
|
||||
} catch(e) {}
|
||||
}
|
||||
|
||||
/**
|
||||
* Load `namespaces`.
|
||||
*
|
||||
* @return {String} returns the previously persisted debug modes
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function load() {
|
||||
var r;
|
||||
try {
|
||||
r = exports.storage.debug;
|
||||
} catch(e) {}
|
||||
return r;
|
||||
}
|
||||
|
||||
/**
|
||||
* Enable namespaces listed in `localStorage.debug` initially.
|
||||
*/
|
||||
|
||||
exports.enable(load());
|
||||
|
||||
/**
|
||||
* Localstorage attempts to return the localstorage.
|
||||
*
|
||||
* This is necessary because safari throws
|
||||
* when a user disables cookies/localstorage
|
||||
* and you attempt to access it.
|
||||
*
|
||||
* @return {LocalStorage}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function localstorage(){
|
||||
try {
|
||||
return window.localStorage;
|
||||
} catch (e) {}
|
||||
}
|
19
node_modules/express/node_modules/debug/component.json
generated
vendored
Normal file
19
node_modules/express/node_modules/debug/component.json
generated
vendored
Normal file
@ -0,0 +1,19 @@
|
||||
{
|
||||
"name": "debug",
|
||||
"repo": "visionmedia/debug",
|
||||
"description": "small debugging utility",
|
||||
"version": "2.2.0",
|
||||
"keywords": [
|
||||
"debug",
|
||||
"log",
|
||||
"debugger"
|
||||
],
|
||||
"main": "browser.js",
|
||||
"scripts": [
|
||||
"browser.js",
|
||||
"debug.js"
|
||||
],
|
||||
"dependencies": {
|
||||
"rauchg/ms.js": "0.7.1"
|
||||
}
|
||||
}
|
197
node_modules/express/node_modules/debug/debug.js
generated
vendored
Normal file
197
node_modules/express/node_modules/debug/debug.js
generated
vendored
Normal file
@ -0,0 +1,197 @@
|
||||
|
||||
/**
|
||||
* This is the common logic for both the Node.js and web browser
|
||||
* implementations of `debug()`.
|
||||
*
|
||||
* Expose `debug()` as the module.
|
||||
*/
|
||||
|
||||
exports = module.exports = debug;
|
||||
exports.coerce = coerce;
|
||||
exports.disable = disable;
|
||||
exports.enable = enable;
|
||||
exports.enabled = enabled;
|
||||
exports.humanize = require('ms');
|
||||
|
||||
/**
|
||||
* The currently active debug mode names, and names to skip.
|
||||
*/
|
||||
|
||||
exports.names = [];
|
||||
exports.skips = [];
|
||||
|
||||
/**
|
||||
* Map of special "%n" handling functions, for the debug "format" argument.
|
||||
*
|
||||
* Valid key names are a single, lowercased letter, i.e. "n".
|
||||
*/
|
||||
|
||||
exports.formatters = {};
|
||||
|
||||
/**
|
||||
* Previously assigned color.
|
||||
*/
|
||||
|
||||
var prevColor = 0;
|
||||
|
||||
/**
|
||||
* Previous log timestamp.
|
||||
*/
|
||||
|
||||
var prevTime;
|
||||
|
||||
/**
|
||||
* Select a color.
|
||||
*
|
||||
* @return {Number}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function selectColor() {
|
||||
return exports.colors[prevColor++ % exports.colors.length];
|
||||
}
|
||||
|
||||
/**
|
||||
* Create a debugger with the given `namespace`.
|
||||
*
|
||||
* @param {String} namespace
|
||||
* @return {Function}
|
||||
* @api public
|
||||
*/
|
||||
|
||||
function debug(namespace) {
|
||||
|
||||
// define the `disabled` version
|
||||
function disabled() {
|
||||
}
|
||||
disabled.enabled = false;
|
||||
|
||||
// define the `enabled` version
|
||||
function enabled() {
|
||||
|
||||
var self = enabled;
|
||||
|
||||
// set `diff` timestamp
|
||||
var curr = +new Date();
|
||||
var ms = curr - (prevTime || curr);
|
||||
self.diff = ms;
|
||||
self.prev = prevTime;
|
||||
self.curr = curr;
|
||||
prevTime = curr;
|
||||
|
||||
// add the `color` if not set
|
||||
if (null == self.useColors) self.useColors = exports.useColors();
|
||||
if (null == self.color && self.useColors) self.color = selectColor();
|
||||
|
||||
var args = Array.prototype.slice.call(arguments);
|
||||
|
||||
args[0] = exports.coerce(args[0]);
|
||||
|
||||
if ('string' !== typeof args[0]) {
|
||||
// anything else let's inspect with %o
|
||||
args = ['%o'].concat(args);
|
||||
}
|
||||
|
||||
// apply any `formatters` transformations
|
||||
var index = 0;
|
||||
args[0] = args[0].replace(/%([a-z%])/g, function(match, format) {
|
||||
// if we encounter an escaped % then don't increase the array index
|
||||
if (match === '%%') return match;
|
||||
index++;
|
||||
var formatter = exports.formatters[format];
|
||||
if ('function' === typeof formatter) {
|
||||
var val = args[index];
|
||||
match = formatter.call(self, val);
|
||||
|
||||
// now we need to remove `args[index]` since it's inlined in the `format`
|
||||
args.splice(index, 1);
|
||||
index--;
|
||||
}
|
||||
return match;
|
||||
});
|
||||
|
||||
if ('function' === typeof exports.formatArgs) {
|
||||
args = exports.formatArgs.apply(self, args);
|
||||
}
|
||||
var logFn = enabled.log || exports.log || console.log.bind(console);
|
||||
logFn.apply(self, args);
|
||||
}
|
||||
enabled.enabled = true;
|
||||
|
||||
var fn = exports.enabled(namespace) ? enabled : disabled;
|
||||
|
||||
fn.namespace = namespace;
|
||||
|
||||
return fn;
|
||||
}
|
||||
|
||||
/**
|
||||
* Enables a debug mode by namespaces. This can include modes
|
||||
* separated by a colon and wildcards.
|
||||
*
|
||||
* @param {String} namespaces
|
||||
* @api public
|
||||
*/
|
||||
|
||||
function enable(namespaces) {
|
||||
exports.save(namespaces);
|
||||
|
||||
var split = (namespaces || '').split(/[\s,]+/);
|
||||
var len = split.length;
|
||||
|
||||
for (var i = 0; i < len; i++) {
|
||||
if (!split[i]) continue; // ignore empty strings
|
||||
namespaces = split[i].replace(/\*/g, '.*?');
|
||||
if (namespaces[0] === '-') {
|
||||
exports.skips.push(new RegExp('^' + namespaces.substr(1) + '$'));
|
||||
} else {
|
||||
exports.names.push(new RegExp('^' + namespaces + '$'));
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Disable debug output.
|
||||
*
|
||||
* @api public
|
||||
*/
|
||||
|
||||
function disable() {
|
||||
exports.enable('');
|
||||
}
|
||||
|
||||
/**
|
||||
* Returns true if the given mode name is enabled, false otherwise.
|
||||
*
|
||||
* @param {String} name
|
||||
* @return {Boolean}
|
||||
* @api public
|
||||
*/
|
||||
|
||||
function enabled(name) {
|
||||
var i, len;
|
||||
for (i = 0, len = exports.skips.length; i < len; i++) {
|
||||
if (exports.skips[i].test(name)) {
|
||||
return false;
|
||||
}
|
||||
}
|
||||
for (i = 0, len = exports.names.length; i < len; i++) {
|
||||
if (exports.names[i].test(name)) {
|
||||
return true;
|
||||
}
|
||||
}
|
||||
return false;
|
||||
}
|
||||
|
||||
/**
|
||||
* Coerce `val`.
|
||||
*
|
||||
* @param {Mixed} val
|
||||
* @return {Mixed}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function coerce(val) {
|
||||
if (val instanceof Error) return val.stack || val.message;
|
||||
return val;
|
||||
}
|
209
node_modules/express/node_modules/debug/node.js
generated
vendored
Normal file
209
node_modules/express/node_modules/debug/node.js
generated
vendored
Normal file
@ -0,0 +1,209 @@
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
*/
|
||||
|
||||
var tty = require('tty');
|
||||
var util = require('util');
|
||||
|
||||
/**
|
||||
* This is the Node.js implementation of `debug()`.
|
||||
*
|
||||
* Expose `debug()` as the module.
|
||||
*/
|
||||
|
||||
exports = module.exports = require('./debug');
|
||||
exports.log = log;
|
||||
exports.formatArgs = formatArgs;
|
||||
exports.save = save;
|
||||
exports.load = load;
|
||||
exports.useColors = useColors;
|
||||
|
||||
/**
|
||||
* Colors.
|
||||
*/
|
||||
|
||||
exports.colors = [6, 2, 3, 4, 5, 1];
|
||||
|
||||
/**
|
||||
* The file descriptor to write the `debug()` calls to.
|
||||
* Set the `DEBUG_FD` env variable to override with another value. i.e.:
|
||||
*
|
||||
* $ DEBUG_FD=3 node script.js 3>debug.log
|
||||
*/
|
||||
|
||||
var fd = parseInt(process.env.DEBUG_FD, 10) || 2;
|
||||
var stream = 1 === fd ? process.stdout :
|
||||
2 === fd ? process.stderr :
|
||||
createWritableStdioStream(fd);
|
||||
|
||||
/**
|
||||
* Is stdout a TTY? Colored output is enabled when `true`.
|
||||
*/
|
||||
|
||||
function useColors() {
|
||||
var debugColors = (process.env.DEBUG_COLORS || '').trim().toLowerCase();
|
||||
if (0 === debugColors.length) {
|
||||
return tty.isatty(fd);
|
||||
} else {
|
||||
return '0' !== debugColors
|
||||
&& 'no' !== debugColors
|
||||
&& 'false' !== debugColors
|
||||
&& 'disabled' !== debugColors;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Map %o to `util.inspect()`, since Node doesn't do that out of the box.
|
||||
*/
|
||||
|
||||
var inspect = (4 === util.inspect.length ?
|
||||
// node <= 0.8.x
|
||||
function (v, colors) {
|
||||
return util.inspect(v, void 0, void 0, colors);
|
||||
} :
|
||||
// node > 0.8.x
|
||||
function (v, colors) {
|
||||
return util.inspect(v, { colors: colors });
|
||||
}
|
||||
);
|
||||
|
||||
exports.formatters.o = function(v) {
|
||||
return inspect(v, this.useColors)
|
||||
.replace(/\s*\n\s*/g, ' ');
|
||||
};
|
||||
|
||||
/**
|
||||
* Adds ANSI color escape codes if enabled.
|
||||
*
|
||||
* @api public
|
||||
*/
|
||||
|
||||
function formatArgs() {
|
||||
var args = arguments;
|
||||
var useColors = this.useColors;
|
||||
var name = this.namespace;
|
||||
|
||||
if (useColors) {
|
||||
var c = this.color;
|
||||
|
||||
args[0] = ' \u001b[3' + c + ';1m' + name + ' '
|
||||
+ '\u001b[0m'
|
||||
+ args[0] + '\u001b[3' + c + 'm'
|
||||
+ ' +' + exports.humanize(this.diff) + '\u001b[0m';
|
||||
} else {
|
||||
args[0] = new Date().toUTCString()
|
||||
+ ' ' + name + ' ' + args[0];
|
||||
}
|
||||
return args;
|
||||
}
|
||||
|
||||
/**
|
||||
* Invokes `console.error()` with the specified arguments.
|
||||
*/
|
||||
|
||||
function log() {
|
||||
return stream.write(util.format.apply(this, arguments) + '\n');
|
||||
}
|
||||
|
||||
/**
|
||||
* Save `namespaces`.
|
||||
*
|
||||
* @param {String} namespaces
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function save(namespaces) {
|
||||
if (null == namespaces) {
|
||||
// If you set a process.env field to null or undefined, it gets cast to the
|
||||
// string 'null' or 'undefined'. Just delete instead.
|
||||
delete process.env.DEBUG;
|
||||
} else {
|
||||
process.env.DEBUG = namespaces;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Load `namespaces`.
|
||||
*
|
||||
* @return {String} returns the previously persisted debug modes
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function load() {
|
||||
return process.env.DEBUG;
|
||||
}
|
||||
|
||||
/**
|
||||
* Copied from `node/src/node.js`.
|
||||
*
|
||||
* XXX: It's lame that node doesn't expose this API out-of-the-box. It also
|
||||
* relies on the undocumented `tty_wrap.guessHandleType()` which is also lame.
|
||||
*/
|
||||
|
||||
function createWritableStdioStream (fd) {
|
||||
var stream;
|
||||
var tty_wrap = process.binding('tty_wrap');
|
||||
|
||||
// Note stream._type is used for test-module-load-list.js
|
||||
|
||||
switch (tty_wrap.guessHandleType(fd)) {
|
||||
case 'TTY':
|
||||
stream = new tty.WriteStream(fd);
|
||||
stream._type = 'tty';
|
||||
|
||||
// Hack to have stream not keep the event loop alive.
|
||||
// See https://github.com/joyent/node/issues/1726
|
||||
if (stream._handle && stream._handle.unref) {
|
||||
stream._handle.unref();
|
||||
}
|
||||
break;
|
||||
|
||||
case 'FILE':
|
||||
var fs = require('fs');
|
||||
stream = new fs.SyncWriteStream(fd, { autoClose: false });
|
||||
stream._type = 'fs';
|
||||
break;
|
||||
|
||||
case 'PIPE':
|
||||
case 'TCP':
|
||||
var net = require('net');
|
||||
stream = new net.Socket({
|
||||
fd: fd,
|
||||
readable: false,
|
||||
writable: true
|
||||
});
|
||||
|
||||
// FIXME Should probably have an option in net.Socket to create a
|
||||
// stream from an existing fd which is writable only. But for now
|
||||
// we'll just add this hack and set the `readable` member to false.
|
||||
// Test: ./node test/fixtures/echo.js < /etc/passwd
|
||||
stream.readable = false;
|
||||
stream.read = null;
|
||||
stream._type = 'pipe';
|
||||
|
||||
// FIXME Hack to have stream not keep the event loop alive.
|
||||
// See https://github.com/joyent/node/issues/1726
|
||||
if (stream._handle && stream._handle.unref) {
|
||||
stream._handle.unref();
|
||||
}
|
||||
break;
|
||||
|
||||
default:
|
||||
// Probably an error on in uv_guess_handle()
|
||||
throw new Error('Implement me. Unknown stream file type!');
|
||||
}
|
||||
|
||||
// For supporting legacy API we put the FD here.
|
||||
stream.fd = fd;
|
||||
|
||||
stream._isStdio = true;
|
||||
|
||||
return stream;
|
||||
}
|
||||
|
||||
/**
|
||||
* Enable namespaces listed in `process.env.DEBUG` initially.
|
||||
*/
|
||||
|
||||
exports.enable(load());
|
5
node_modules/express/node_modules/debug/node_modules/ms/.npmignore
generated
vendored
Normal file
5
node_modules/express/node_modules/debug/node_modules/ms/.npmignore
generated
vendored
Normal file
@ -0,0 +1,5 @@
|
||||
node_modules
|
||||
test
|
||||
History.md
|
||||
Makefile
|
||||
component.json
|
66
node_modules/express/node_modules/debug/node_modules/ms/History.md
generated
vendored
Normal file
66
node_modules/express/node_modules/debug/node_modules/ms/History.md
generated
vendored
Normal file
@ -0,0 +1,66 @@
|
||||
|
||||
0.7.1 / 2015-04-20
|
||||
==================
|
||||
|
||||
* prevent extraordinary long inputs (@evilpacket)
|
||||
* Fixed broken readme link
|
||||
|
||||
0.7.0 / 2014-11-24
|
||||
==================
|
||||
|
||||
* add time abbreviations, updated tests and readme for the new units
|
||||
* fix example in the readme.
|
||||
* add LICENSE file
|
||||
|
||||
0.6.2 / 2013-12-05
|
||||
==================
|
||||
|
||||
* Adding repository section to package.json to suppress warning from NPM.
|
||||
|
||||
0.6.1 / 2013-05-10
|
||||
==================
|
||||
|
||||
* fix singularization [visionmedia]
|
||||
|
||||
0.6.0 / 2013-03-15
|
||||
==================
|
||||
|
||||
* fix minutes
|
||||
|
||||
0.5.1 / 2013-02-24
|
||||
==================
|
||||
|
||||
* add component namespace
|
||||
|
||||
0.5.0 / 2012-11-09
|
||||
==================
|
||||
|
||||
* add short formatting as default and .long option
|
||||
* add .license property to component.json
|
||||
* add version to component.json
|
||||
|
||||
0.4.0 / 2012-10-22
|
||||
==================
|
||||
|
||||
* add rounding to fix crazy decimals
|
||||
|
||||
0.3.0 / 2012-09-07
|
||||
==================
|
||||
|
||||
* fix `ms(<String>)` [visionmedia]
|
||||
|
||||
0.2.0 / 2012-09-03
|
||||
==================
|
||||
|
||||
* add component.json [visionmedia]
|
||||
* add days support [visionmedia]
|
||||
* add hours support [visionmedia]
|
||||
* add minutes support [visionmedia]
|
||||
* add seconds support [visionmedia]
|
||||
* add ms string support [visionmedia]
|
||||
* refactor tests to facilitate ms(number) [visionmedia]
|
||||
|
||||
0.1.0 / 2012-03-07
|
||||
==================
|
||||
|
||||
* Initial release
|
20
node_modules/express/node_modules/debug/node_modules/ms/LICENSE
generated
vendored
Normal file
20
node_modules/express/node_modules/debug/node_modules/ms/LICENSE
generated
vendored
Normal file
@ -0,0 +1,20 @@
|
||||
(The MIT License)
|
||||
|
||||
Copyright (c) 2014 Guillermo Rauch <rauchg@gmail.com>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy of
|
||||
this software and associated documentation files (the "Software"), to deal in
|
||||
the Software without restriction, including without limitation the rights to
|
||||
use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
|
||||
the Software, and to permit persons to whom the Software is furnished to do so,
|
||||
subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
|
||||
FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
|
||||
COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
|
||||
IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
|
||||
CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
35
node_modules/express/node_modules/debug/node_modules/ms/README.md
generated
vendored
Normal file
35
node_modules/express/node_modules/debug/node_modules/ms/README.md
generated
vendored
Normal file
@ -0,0 +1,35 @@
|
||||
# ms.js: miliseconds conversion utility
|
||||
|
||||
```js
|
||||
ms('2 days') // 172800000
|
||||
ms('1d') // 86400000
|
||||
ms('10h') // 36000000
|
||||
ms('2.5 hrs') // 9000000
|
||||
ms('2h') // 7200000
|
||||
ms('1m') // 60000
|
||||
ms('5s') // 5000
|
||||
ms('100') // 100
|
||||
```
|
||||
|
||||
```js
|
||||
ms(60000) // "1m"
|
||||
ms(2 * 60000) // "2m"
|
||||
ms(ms('10 hours')) // "10h"
|
||||
```
|
||||
|
||||
```js
|
||||
ms(60000, { long: true }) // "1 minute"
|
||||
ms(2 * 60000, { long: true }) // "2 minutes"
|
||||
ms(ms('10 hours'), { long: true }) // "10 hours"
|
||||
```
|
||||
|
||||
- Node/Browser compatible. Published as [`ms`](https://www.npmjs.org/package/ms) in [NPM](http://nodejs.org/download).
|
||||
- If a number is supplied to `ms`, a string with a unit is returned.
|
||||
- If a string that contains the number is supplied, it returns it as
|
||||
a number (e.g: it returns `100` for `'100'`).
|
||||
- If you pass a string with a number and a valid unit, the number of
|
||||
equivalent ms is returned.
|
||||
|
||||
## License
|
||||
|
||||
MIT
|
125
node_modules/express/node_modules/debug/node_modules/ms/index.js
generated
vendored
Normal file
125
node_modules/express/node_modules/debug/node_modules/ms/index.js
generated
vendored
Normal file
@ -0,0 +1,125 @@
|
||||
/**
|
||||
* Helpers.
|
||||
*/
|
||||
|
||||
var s = 1000;
|
||||
var m = s * 60;
|
||||
var h = m * 60;
|
||||
var d = h * 24;
|
||||
var y = d * 365.25;
|
||||
|
||||
/**
|
||||
* Parse or format the given `val`.
|
||||
*
|
||||
* Options:
|
||||
*
|
||||
* - `long` verbose formatting [false]
|
||||
*
|
||||
* @param {String|Number} val
|
||||
* @param {Object} options
|
||||
* @return {String|Number}
|
||||
* @api public
|
||||
*/
|
||||
|
||||
module.exports = function(val, options){
|
||||
options = options || {};
|
||||
if ('string' == typeof val) return parse(val);
|
||||
return options.long
|
||||
? long(val)
|
||||
: short(val);
|
||||
};
|
||||
|
||||
/**
|
||||
* Parse the given `str` and return milliseconds.
|
||||
*
|
||||
* @param {String} str
|
||||
* @return {Number}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function parse(str) {
|
||||
str = '' + str;
|
||||
if (str.length > 10000) return;
|
||||
var match = /^((?:\d+)?\.?\d+) *(milliseconds?|msecs?|ms|seconds?|secs?|s|minutes?|mins?|m|hours?|hrs?|h|days?|d|years?|yrs?|y)?$/i.exec(str);
|
||||
if (!match) return;
|
||||
var n = parseFloat(match[1]);
|
||||
var type = (match[2] || 'ms').toLowerCase();
|
||||
switch (type) {
|
||||
case 'years':
|
||||
case 'year':
|
||||
case 'yrs':
|
||||
case 'yr':
|
||||
case 'y':
|
||||
return n * y;
|
||||
case 'days':
|
||||
case 'day':
|
||||
case 'd':
|
||||
return n * d;
|
||||
case 'hours':
|
||||
case 'hour':
|
||||
case 'hrs':
|
||||
case 'hr':
|
||||
case 'h':
|
||||
return n * h;
|
||||
case 'minutes':
|
||||
case 'minute':
|
||||
case 'mins':
|
||||
case 'min':
|
||||
case 'm':
|
||||
return n * m;
|
||||
case 'seconds':
|
||||
case 'second':
|
||||
case 'secs':
|
||||
case 'sec':
|
||||
case 's':
|
||||
return n * s;
|
||||
case 'milliseconds':
|
||||
case 'millisecond':
|
||||
case 'msecs':
|
||||
case 'msec':
|
||||
case 'ms':
|
||||
return n;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Short format for `ms`.
|
||||
*
|
||||
* @param {Number} ms
|
||||
* @return {String}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function short(ms) {
|
||||
if (ms >= d) return Math.round(ms / d) + 'd';
|
||||
if (ms >= h) return Math.round(ms / h) + 'h';
|
||||
if (ms >= m) return Math.round(ms / m) + 'm';
|
||||
if (ms >= s) return Math.round(ms / s) + 's';
|
||||
return ms + 'ms';
|
||||
}
|
||||
|
||||
/**
|
||||
* Long format for `ms`.
|
||||
*
|
||||
* @param {Number} ms
|
||||
* @return {String}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function long(ms) {
|
||||
return plural(ms, d, 'day')
|
||||
|| plural(ms, h, 'hour')
|
||||
|| plural(ms, m, 'minute')
|
||||
|| plural(ms, s, 'second')
|
||||
|| ms + ' ms';
|
||||
}
|
||||
|
||||
/**
|
||||
* Pluralization helper.
|
||||
*/
|
||||
|
||||
function plural(ms, n, name) {
|
||||
if (ms < n) return;
|
||||
if (ms < n * 1.5) return Math.floor(ms / n) + ' ' + name;
|
||||
return Math.ceil(ms / n) + ' ' + name + 's';
|
||||
}
|
47
node_modules/express/node_modules/debug/node_modules/ms/package.json
generated
vendored
Normal file
47
node_modules/express/node_modules/debug/node_modules/ms/package.json
generated
vendored
Normal file
@ -0,0 +1,47 @@
|
||||
{
|
||||
"name": "ms",
|
||||
"version": "0.7.1",
|
||||
"description": "Tiny ms conversion utility",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git://github.com/guille/ms.js.git"
|
||||
},
|
||||
"main": "./index",
|
||||
"devDependencies": {
|
||||
"mocha": "*",
|
||||
"expect.js": "*",
|
||||
"serve": "*"
|
||||
},
|
||||
"component": {
|
||||
"scripts": {
|
||||
"ms/index.js": "index.js"
|
||||
}
|
||||
},
|
||||
"gitHead": "713dcf26d9e6fd9dbc95affe7eff9783b7f1b909",
|
||||
"bugs": {
|
||||
"url": "https://github.com/guille/ms.js/issues"
|
||||
},
|
||||
"homepage": "https://github.com/guille/ms.js",
|
||||
"_id": "ms@0.7.1",
|
||||
"scripts": {},
|
||||
"_shasum": "9cd13c03adbff25b65effde7ce864ee952017098",
|
||||
"_from": "ms@0.7.1",
|
||||
"_npmVersion": "2.7.5",
|
||||
"_nodeVersion": "0.12.2",
|
||||
"_npmUser": {
|
||||
"name": "rauchg",
|
||||
"email": "rauchg@gmail.com"
|
||||
},
|
||||
"maintainers": [
|
||||
{
|
||||
"name": "rauchg",
|
||||
"email": "rauchg@gmail.com"
|
||||
}
|
||||
],
|
||||
"dist": {
|
||||
"shasum": "9cd13c03adbff25b65effde7ce864ee952017098",
|
||||
"tarball": "http://registry.npmjs.org/ms/-/ms-0.7.1.tgz"
|
||||
},
|
||||
"directories": {},
|
||||
"_resolved": "https://registry.npmjs.org/ms/-/ms-0.7.1.tgz"
|
||||
}
|
Some files were not shown because too many files have changed in this diff Show More
Loading…
Reference in New Issue
Block a user